#golang
Are Channels Always Needed When You Use Go Routines?
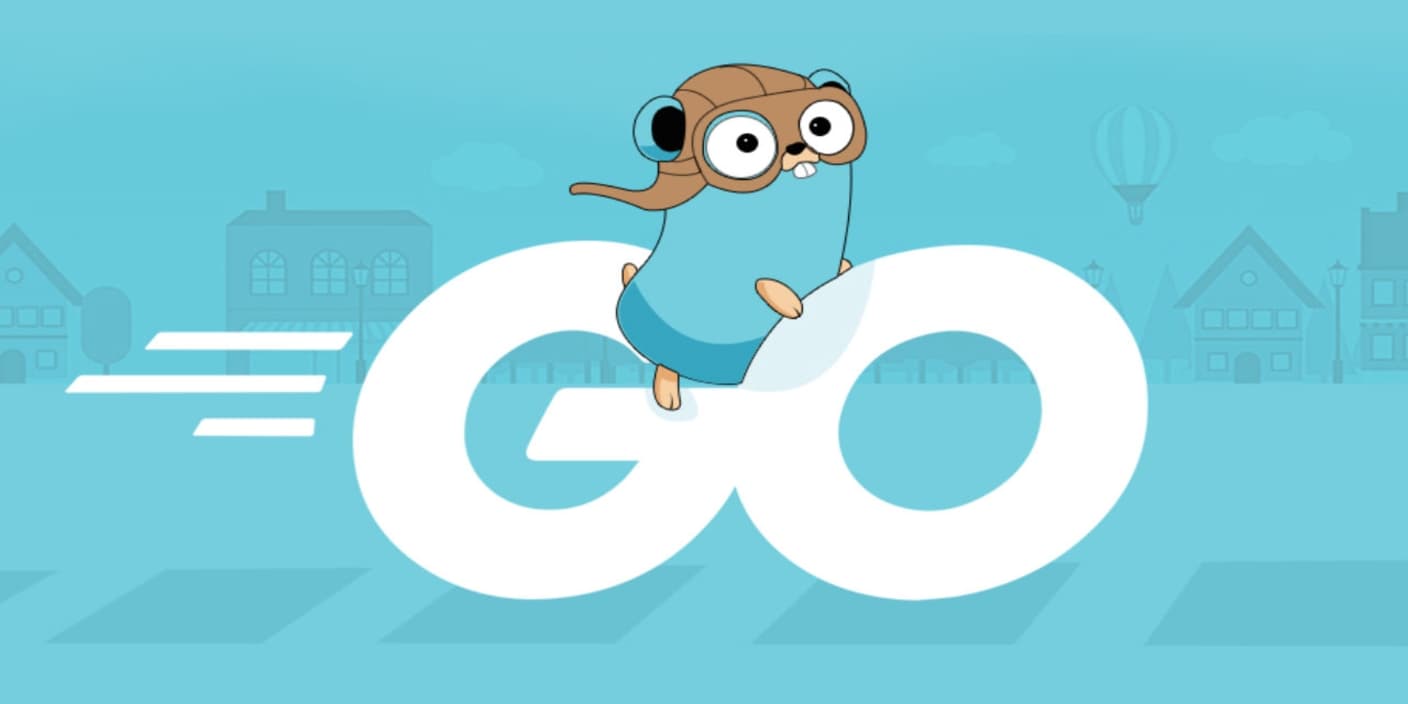
Are Channels Always Needed When You Use Go Routines?
No, you do not need to open a channel every time you use goroutines in Go. Channels are one way to communicate and synchronize between goroutines, but they are not always required. You only need to use channels when you want goroutines to send or receive data to/from each other or from the main goroutine.
When to Use Channels with Goroutines:
- Communication Between Goroutines: If you have multiple goroutines that need to send data to one another or back to the main function, channels provide a safe way to do so without data races.
- Synchronization: Channels can be used to synchronize the execution of goroutines. For example, you can block the main goroutine until it receives data from other goroutines.
When Channels Are Not Needed:
- Independent Tasks: If your goroutines perform independent work and don't need to share results or data between them, you don't need a channel. For instance, if each goroutine writes to separate files or performs calculations that don't need to be aggregated or communicated to other goroutines, channels are unnecessary.
- Using other synchronization mechanisms: You can use other concurrency primitives such as
sync.WaitGroup
,sync.Mutex
, orsync.Once
without channels. For example,sync.WaitGroup
can be used to wait for a group of goroutines to finish without channels.
Example Without Channels:
Here’s an example where you use a sync.WaitGroup
to ensure that all goroutines complete without needing a channel to communicate data back to the main function:
package main
import (
"fmt"
"sync"
)
// Worker function that just prints the work ID
func worker(id int, wg *sync.WaitGroup) {
defer wg.Done() // Signal that this goroutine is done
fmt.Printf("Worker %d starting\n", id)
// Simulate doing some work
// time.Sleep(time.Second)
fmt.Printf("Worker %d done\n", id)
}
func main() {
var wg sync.WaitGroup // Create a WaitGroup
for i := 1; i <= 5; i++ {
wg.Add(1) // Increment the WaitGroup counter
go worker(i, &wg) // Launch a goroutine
}
// Wait for all goroutines to finish
wg.Wait()
fmt.Println("All workers done.")
}
Explanation:
- We launch 5 goroutines, each running the
worker
function. - No channels are needed because the main goroutine just waits for all goroutines to finish using a
sync.WaitGroup
. - The
wg.Add(1)
increments the number of goroutines that the main goroutine will wait for, and each goroutine callswg.Done()
when it completes.
Example with a Channel:
Let’s look at a scenario where we do need a channel. For example, if each worker has to return some result back to the main function, a channel would be appropriate:
package main
import (
"fmt"
"sync"
)
// Worker function that sends results back to a channel
func worker(id int, wg *sync.WaitGroup, results chan<- int) {
defer wg.Done()
fmt.Printf("Worker %d starting\n", id)
// Simulate doing some work and send result
results <- id * 10
fmt.Printf("Worker %d done\n", id)
}
func main() {
var wg sync.WaitGroup
results := make(chan int, 5) // Buffered channel to collect results
for i := 1; i <= 5; i++ {
wg.Add(1)
go worker(i, &wg, results) // Launch goroutines, passing the channel
}
// Close the results channel after all workers are done
go func() {
wg.Wait()
close(results)
}()
// Collect results from the workers
for result := range results {
fmt.Printf("Result: %d\n", result)
}
fmt.Println("All workers done, results collected.")
}
Explanation:
- Channel: We use a channel
results
to collect the output from the worker goroutines. - Buffered channel: The channel is buffered so that workers can send data without waiting for the receiver to consume it immediately.
- WaitGroup: We still use a
WaitGroup
to wait for all goroutines to finish. - The main function collects the results from the channel after all workers have completed.
When You Might Use Channels:
- Data Collection: If each goroutine needs to return some data to the main function or another goroutine, you would use a channel.
- Concurrency Management: Channels can also be used to control how many goroutines are running simultaneously, for example, by using a semaphore-like pattern.
Conclusion:
- Not always required: You do not need to use channels every time you use goroutines. Use channels only when you need to communicate between goroutines or synchronize them.
- Other options: For synchronization, you can also use other mechanisms like
sync.WaitGroup
,sync.Mutex
, orsync.Once
if there's no need for communication between goroutines.