Barrel Files: Why You Should Rethink Using Them in Your Projects
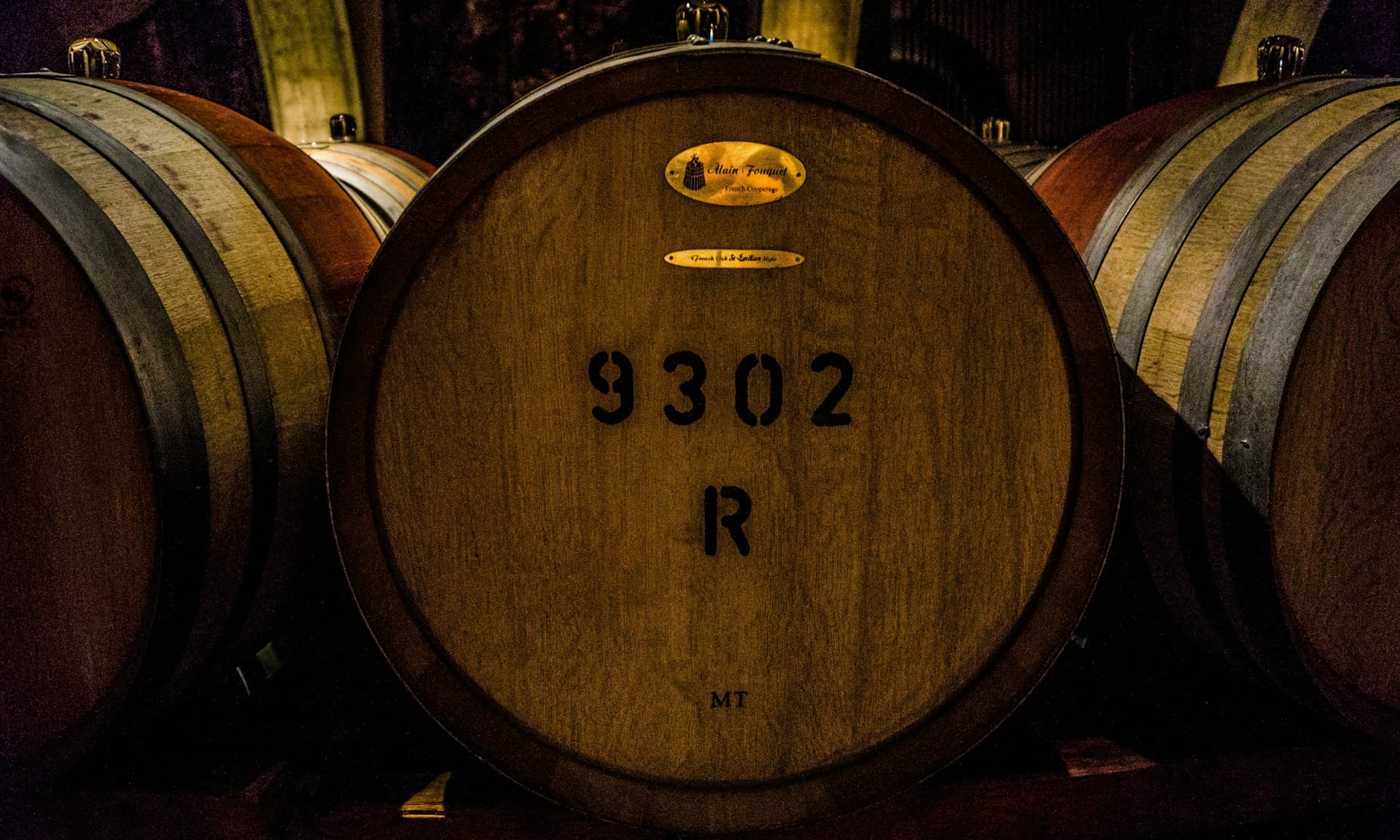
Table of Content
In the realm of software development, small architectural decisions can have significant impacts on performance, scalability, and maintainability. One such decision is whether to use barrel files, a pattern that, while seemingly convenient, comes with hidden costs, especially in frontend applications.
This article delves into the drawbacks of barrel files and explains why you might want to reconsider their usage in your projects.
What Are Barrel Files?
Barrel files are a pattern for simplifying import paths. They aggregate exports from multiple files into a single "barrel," allowing developers to import everything from one central location.
Example:
// ./barrelFiles/used.ts
export const HEY = "hey used";
// ./barrelFiles/notUsed.ts
export const HEY_NOT_USED = "hey NOT used";
// ./barrelFiles/index.ts
export { HEY_NOT_USED } from "./notUsed";
export { HEY } from "./used";
Using Barrel Files
Instead of importing directly:
import { HEY } from "./barrelFiles/used";
You can import everything via the barrel file:
import { HEY } from "./barrelFiles";
While this seems cleaner and improves developer experience initially, the downsides often outweigh the benefits.
The Hidden Costs of Barrel Files
1. Increased Application Bundle Size
A key concern with barrel files is that importing from them often pulls in more code than needed. Even if you only import HEY
, everything in the barrel file is loaded.
Example:
// ./barrelFiles/used.ts
export const HEY = "hey used";
console.log("I am used");
// ./barrelFiles/notUsed.ts
export const HEY_NOT_USED = "hey NOT used";
console.log("I am NOT used");
When you import HEY
via the barrel file:
import { HEY } from "./barrelFiles";
All console.log
statements from both used.ts
and notUsed.ts
will execute, increasing the bundle size and potentially impacting performance.
Bundle Size Comparison:
- Using barrel files: 189.83 KB
- Without barrel files: 189.67 KB
While the difference may seem small, it scales significantly in larger projects, especially in monorepos or applications with shared folders.
2. Circular Dependencies
Barrel files are prone to circular dependencies, especially in large-scale projects. These can cause runtime errors and make debugging significantly harder.
Example:
// fileA.ts
export const A = "A";
// fileB.ts
import { A } from "./fileA";
export const B = "B";
// index.ts (barrel file)
export { A } from "./fileA";
export { B } from "./fileB";
If fileA
also imports something from the barrel file, you create a circular dependency, which can crash your app or cause unexpected behavior.
3. Performance Impact on Unit Tests
In testing environments, barrel files can dramatically increase test execution time. Testing engines like Jest and Vitest don’t optimize imports the way bundlers like Webpack or Vite do. As a result, unnecessary imports slow down tests.
Example:
// helper.ts
import { HEY } from "./barrelFiles";
export const helper = () => console.log(HEY);
// helper2.ts
import { HEY } from "./barrelFiles";
export const helper2 = () => console.log(HEY);
// Tests
// helper.spec.ts
import { helper } from "./helper";
helper();
// helper2.spec.ts
import { helper2 } from "./helper2";
helper2();
Test Execution Times:
- With barrel files: 2.61 seconds
- Without barrel files: 1.32 seconds
The difference grows as the test suite expands.
When Barrel Files Might Still Be Useful
While barrel files have significant drawbacks in frontend applications, there are scenarios where they might still make sense:
- Library Development: If you're building a package where a consistent API is crucial, barrel files can improve usability for consumers.
- Small, Well-Scoped Projects: In smaller projects with minimal imports, the performance impact may be negligible.
- Explicitly Managed Barrel Files: If you carefully curate barrel files to avoid unused exports, they can still be beneficial.
Best Practices for Managing Imports
-
Import Directly When Possible: Avoid barrel files and prefer importing directly from the source file.
import { HEY } from "./barrelFiles/used";
-
Use Tree-Shaking-Friendly Modules: Modern bundlers like Webpack and Vite can optimize imports, but only if your modules are structured correctly.
-
Monitor Bundle Sizes: Regularly analyze your application bundle to identify unnecessary dependencies.
-
Enforce Code Standards: Use tools like ESLint to enforce consistent and optimized import practices.
Conclusion
Barrel files can simplify import paths, but their hidden costs, such as increased bundle size, circular dependencies, and slower test performance, make them a suboptimal choice for many frontend applications. By avoiding barrel files and importing directly, you can build faster, leaner, and more maintainable projects.
As with any pattern, context matters. Evaluate your project's specific needs before adopting barrel files, and when in doubt, prioritize performance and clarity.
Enjoyed this article? Follow me for more insights on optimizing your development workflow! 🚀