Controlling and Manipulating Loop Behavior in JavaScript: A Deep Dive
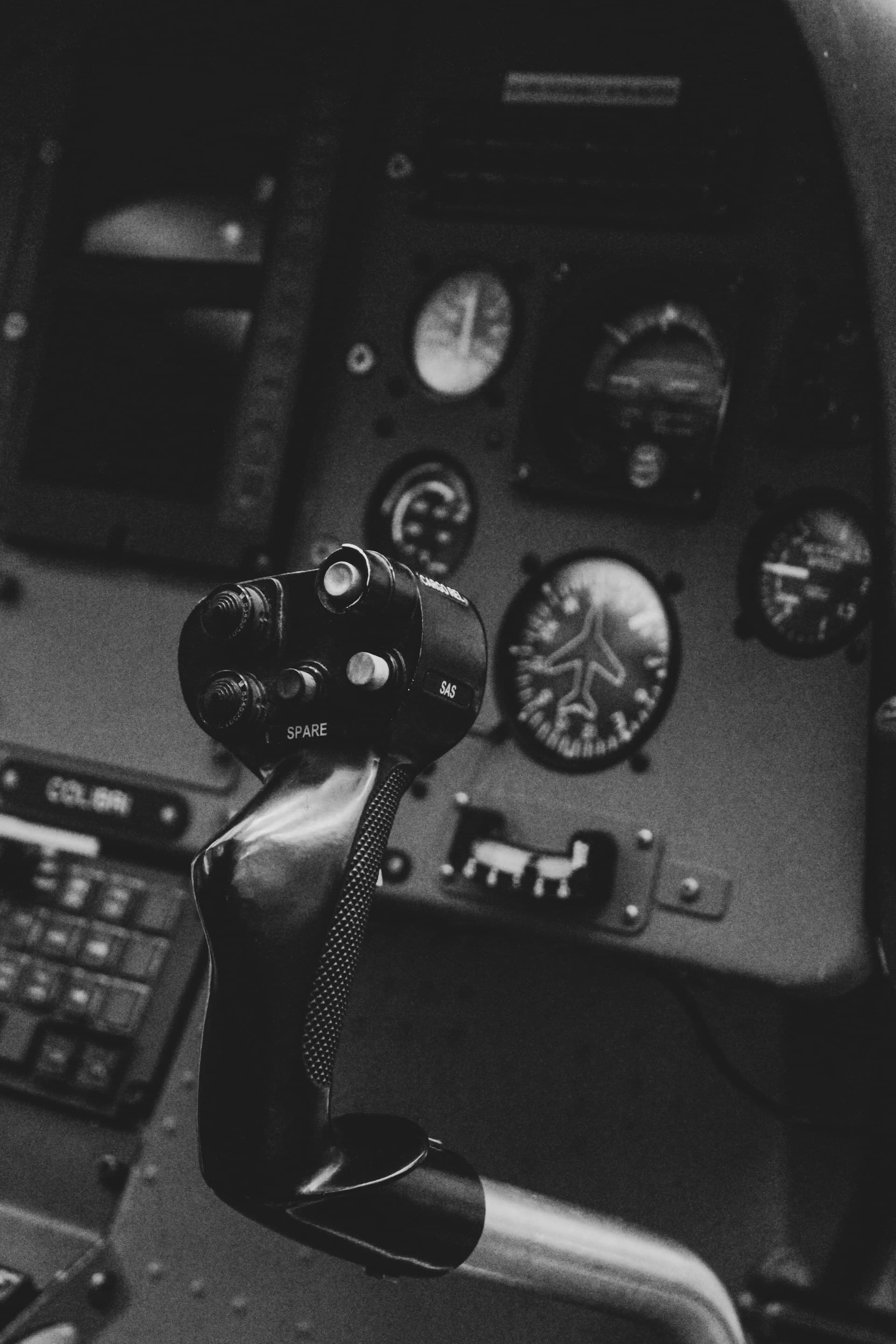
Loops are fundamental in JavaScript programming, providing the ability to iterate over data and execute repetitive tasks efficiently. JavaScript offers a rich set of keywords and constructs to control the behavior of loops, allowing developers to optimize workflows and handle complex scenarios effectively. This article explores these constructs, their applications, and practical examples to demonstrate their use.
1. break
: Exit a Loop Prematurely
The break
keyword is used to exit a loop immediately, skipping any remaining iterations. It is applicable to for
, while
, do-while
loops, and switch
statements.
Example:
for (let i = 0; i < 5; i++) {
if (i === 3) break;
console.log(i); // Output: 0, 1, 2
}
Use Case: Terminate a loop early when a specific condition is met.
2. continue
: Skip the Current Iteration
The continue
keyword skips the rest of the current iteration and proceeds to the next iteration of the loop. It works with for
, while
, and do-while
loops.
Example:
for (let i = 0; i < 5; i++) {
if (i === 3) continue;
console.log(i); // Output: 0, 1, 2, 4
}
Use Case: Skip specific iterations without terminating the loop.
3. return
: Exit the Function and the Loop
When used inside a loop within a function, the return
keyword terminates both the loop and the function. It is particularly useful when searching for a value or condition.
Example:
function findValue(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i; // Exits the loop and function
}
return -1;
}
console.log(findValue([1, 2, 3, 4], 3)); // Output: 2
Use Case: Stop execution and return a value as soon as the desired condition is met.
4. throw
: Exit with an Exception
The throw
keyword stops the loop and the surrounding code block by raising an exception. If used within a try...catch
block, the error can be handled gracefully.
Example:
try {
for (let i = 0; i < 5; i++) {
if (i === 3) throw new Error("Loop terminated");
console.log(i); // Output: 0, 1, 2
}
} catch (e) {
console.log(e.message); // Output: "Loop terminated"
}
Use Case: Exit loops with a specific error condition that requires external handling.
5. yield
: Pause Execution in Generators
Within generator functions, the yield
keyword pauses execution and allows the generator to return a value. When iterating with for...of
, yield
provides a mechanism for controlled iteration.
Example:
function* generator() {
for (let i = 0; i < 5; i++) {
yield i; // Pauses and returns i
}
}
const gen = generator();
console.log(gen.next().value); // Output: 0
console.log(gen.next().value); // Output: 1
Use Case: Create custom iterators or asynchronous sequences.
6. label
: Control Nested Loops
Labels provide a way to name a loop and enable break
or continue
to apply to a specific loop when working with nested loops.
Example (Breaking an Outer Loop):
outerLoop: for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
if (j === 1) break outerLoop; // Exits the outer loop
console.log(`i: ${i}, j: ${j}`);
}
}
// Output: i: 0, j: 0
Example (Continuing an Outer Loop):
outerLoop: for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
if (j === 1) continue outerLoop; // Skips the rest of the outer loop iteration
console.log(`i: ${i}, j: ${j}`);
}
}
// Output: i: 0, j: 0
// i: 1, j: 0
// i: 2, j: 0
Use Case: Manage nested loops with precision.
7. await
: Pause Asynchronous Loops
In async
functions, await
pauses execution until a Promise
resolves, making it possible to handle asynchronous operations within loops.
Example:
async function delayedLog(array) {
for (let item of array) {
await new Promise((resolve) => setTimeout(resolve, 1000));
console.log(item);
}
}
delayedLog([1, 2, 3]); // Logs 1, 2, 3 with a 1-second delay between each
Use Case: Sequentially process asynchronous operations.
Conclusion
Understanding the keywords and constructs that control loop behavior in JavaScript is essential for writing clean, efficient, and maintainable code. Each construct—break
, continue
, return
, throw
, yield
, label
, and await
—has a unique purpose and fits specific scenarios. Mastering these tools empowers developers to handle complex logic with clarity and precision, enhancing both code readability and performance.