The .with() Method in JavaScript: Working with Immutable Objects in ES2023
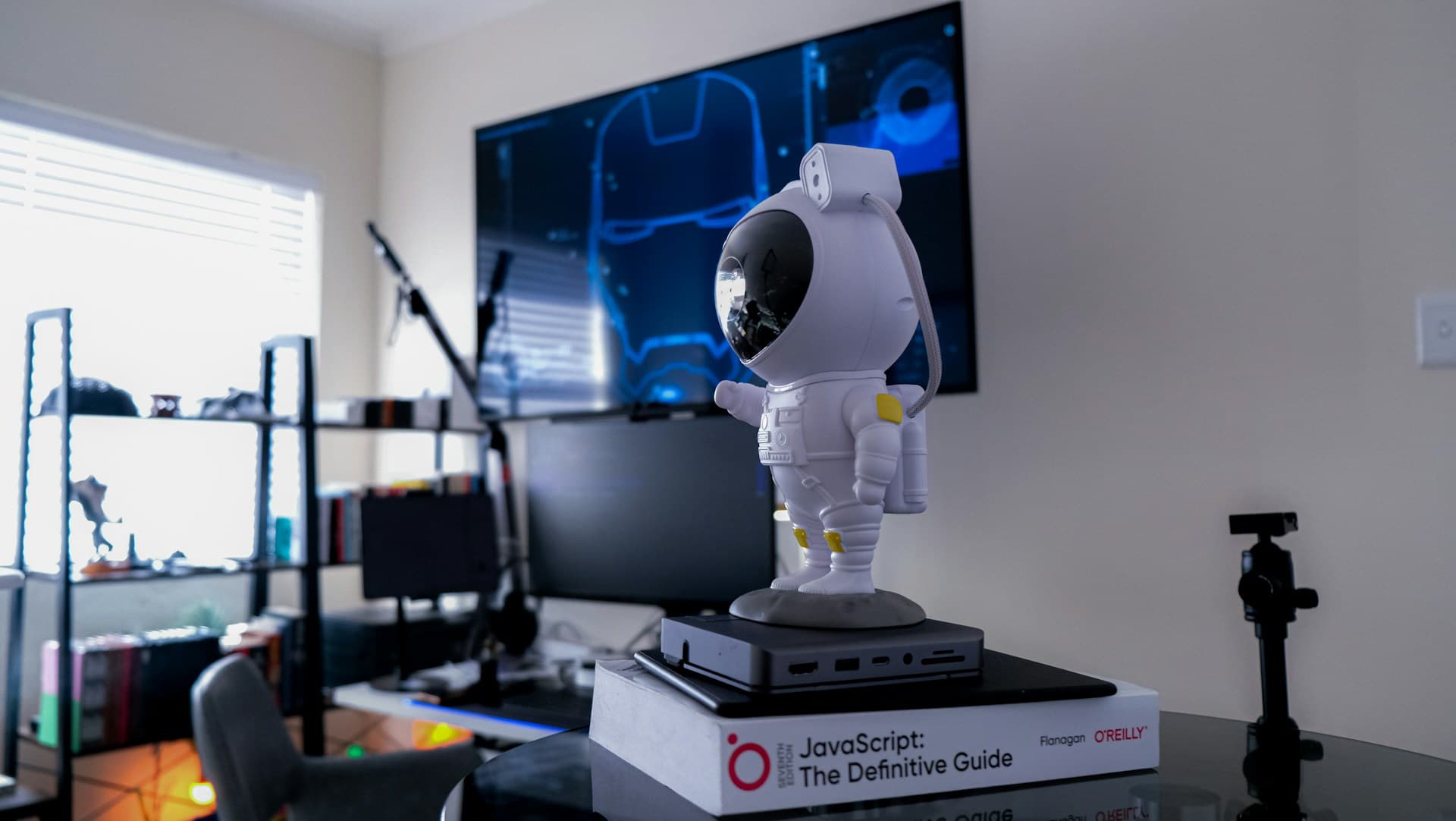
As of ES2023, JavaScript has introduced a new method called .with()
designed specifically for working with immutable objects. This method makes it easier to create copies of an object, allowing you to modify a specific property without altering the original object. This is particularly useful in contexts where data immutability is crucial, such as in applications that rely on immutable states, for example, using frameworks like React.
Syntax of the .with() Method
The usage of the .with()
method is simple. Its basic syntax is as follows:
newObject = originalObject.with(property, newValue);
Where:
property
: This is the key of the object you want to modify.newValue
: This is the new value you want to assign to that property.
The method creates a new copy of the original object, where only the specified property has been modified, while the rest of the object remains unchanged.
Practical Example
Let's assume you have an object representing a user, and you want to change their age without modifying the original object:
const user = { name: "Roy", age: 30 };
// Using the .with() method
const newUser = user.with("age", 31);
console.log(newUser); // { name: 'Roy', age: 31 }
console.log(user); // { name: 'Roy', age: 30 } (the original object remains unchanged)
In this example, the newUser
object is a copy of the original user
object, but with the age
property updated to 31. The original object remains immutable, ensuring that no accidental changes occur.
Benefits of the .with() Method
- Immutability: This method helps preserve immutability in objects, a key principle in functional programming and many modern application architectures.
- Simplicity: Unlike other approaches such as using the spread operator (
...
) orObject.assign()
, the.with()
method provides a more semantic and straightforward way to update properties.
Comparison with the Spread Operator
Before the introduction of the .with()
method, the usual way to update an object without mutating it was to use the spread operator:
const newUser = { ...user, age: 31 };
While this technique is still valid, using .with()
is a clearer and more concise alternative for this type of operation, eliminating the need for more manual techniques.
Important Considerations
- The
.with()
method only works with objects. It is not designed to be used with other data types like arrays. - Since this is a new feature in ES2023, it's important to ensure that the environment or browser you're working in supports this functionality. In some cases, you may need a transpiler or an updated runtime environment.
Conclusion
The .with()
method in JavaScript is a powerful tool for working with immutable objects. It provides a clear and efficient way to update properties without mutating the original object, which is especially useful in applications that rely on immutable states, such as those built with frameworks like React. This new addition to the language reduces code complexity and makes it easier to adopt functional programming patterns.