Exploring Generic Inference with Object Arguments in TypeScript
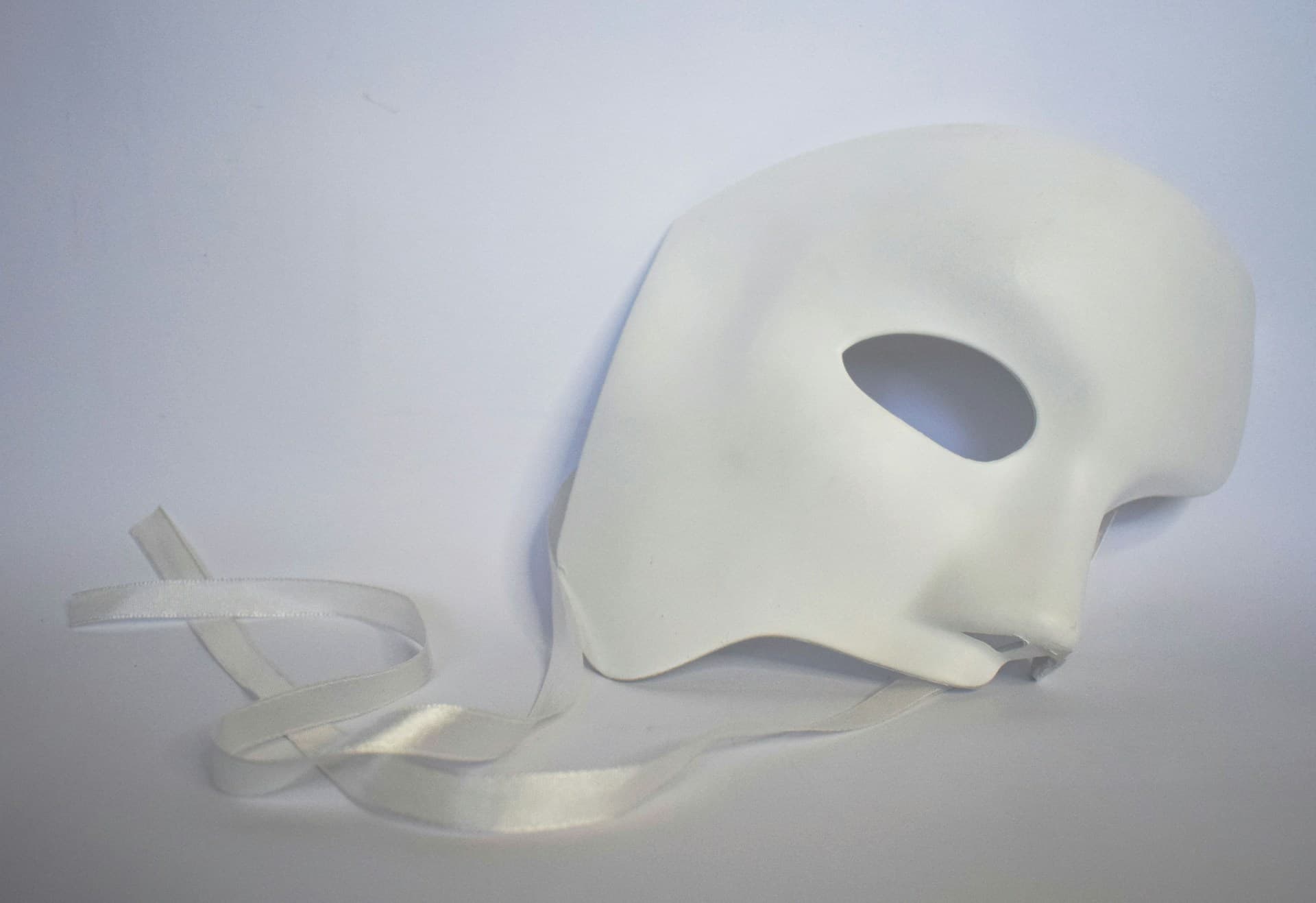
TypeScript’s generic inference is powerful—but its behavior can sometimes be surprising, especially when objects are involved.
In this article, we’ll dive into how TypeScript infers literal types when using objects as arguments. We’ll walk through examples illustrating how subtle changes in the function signature or the way you pass arguments can affect type inference.
Direct Value Inference
Let’s start with a simple generic function that returns the value you pass in. When the value is returned directly, TypeScript preserves its literal type:
function fetchValue<T>(value: T): T {
return value;
}
const directResult = fetchValue("hello");
// The inferred type of directResult is: "hello"
Here, the literal
"hello"
remains as"hello"
, not a widenedstring
. This direct inference is ideal when you want the exact type preserved .
Inference When Extracting a Field from an Object
When you pass a value wrapped inside an object , TypeScript may widen the literal type to a more general type. Consider this function:
function getContent<T>(obj: { data: T }): T {
return obj.data;
}
const contentResult = getContent({ data: "world" });
// The inferred type of contentResult is: string
Even though
"world"
is a literal , it is widened tostring
because TypeScript infers the type for the entire object property without extra hints .Preserving Literal Types withas const
To force TypeScript to preserve the literal type , use a constant assertion withas const
:
const contentLiteral = getContent({ data: "world" } as const);
// Now, contentLiteral is inferred as: "world"
When you use
as const
, the entire object becomes deeply immutable (readonly
properties), allowing TypeScript to capture the literal type of"world"
.
Guiding Inference with Field Constraints
If you want to ensure that the literal type is preserved when extracting a value from an object, apply a constraint on the generic parameter for the field :
function getConstrainedContent<T extends string>(obj: { data: T }): T {
return obj.data;
}
const constrainedResult = getConstrainedContent({ data: "TypeScript" });
// The inferred type of constrainedResult is: "TypeScript"
By constraining
T extends string
, TypeScript is forced to consider the literal value , preserving"TypeScript"
rather than widening it tostring
.
Inference When Constraining the Entire Object
A different scenario arises when the generic parameter represents the entire object rather than just a specific field:
function getWholeObject<T extends { data: string }>(obj: T): string {
return obj.data;
}
const wholeResult = getWholeObject({ data: "Generics", extra: 42 });
// The inferred type of wholeResult is: string
Because
T
represents the entire object , TypeScript only checks ifdata
is astring
but does not infer"Generics"
as a literal.Even if you try to useas const
, the result remains the same:
const wholeResultConst = getWholeObject({
data: "Generics",
extra: 42,
} as const);
// The inferred type of wholeResultConst remains: string
Since the entire object is the generic parameter, TypeScript focuses on the constraint
{ data: string }
, ignoring the literal type"Generics"
. The further you move away from the target value in your type definitions , the less likely TypeScript is to preserve the literal type .
🔑 Key Takeaways
✔ Direct Return Preserves Literals : When a function returns a value directly, TypeScript maintains its literal type .
✔ Object Wrapping Causes Widening : When a value is wrapped inside an object , TypeScript widens its type—unless you use as const
.
✔ Constraints Can Refine Inference : Applying a constraint directly on a field (e.g., T extends string
) can help maintain literal types .
✔ Whole-Object Constraints May Lose Precision : When the generic parameter applies to the entire object , even as const
may not preserve the literal type for nested properties.
Understanding these nuances in generic inference helps you write more precise TypeScript code . By carefully choosing where to place type constraints and how you pass your arguments , you can control whether TypeScript infers a literal type or a broader type —ensuring that your type system behaves as expected .Happy coding with TypeScript! 🚀