Mastering Go: Building Packages and Understanding Imports
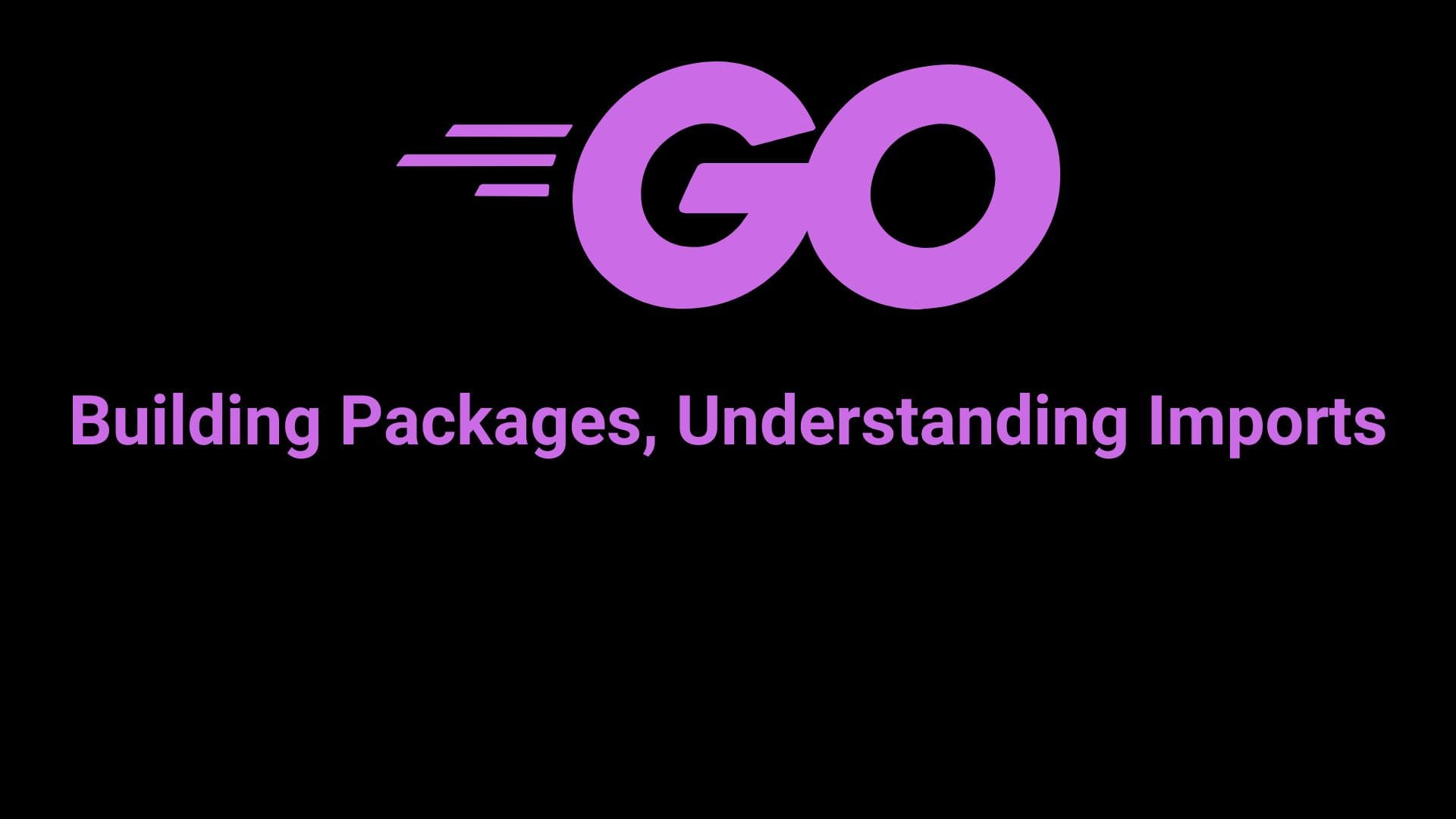
Introduction
In this article, we dive deep into building packages in Go, understanding imports, and using packages to organize your code. We'll explore how Go's import system works, and best practices for creating packages that are clean, maintainable, and well-structured.
Understanding Imports and Exports in Go
We’ve been using the import
statement in Go without a detailed explanation of its inner workings. Go’s import
statement allows access to exported constants, variables, functions, and types from another package. In Go, package visibility is determined by capitalization: identifiers starting with an uppercase letter are exported and visible outside the package, while those starting with a lowercase letter remain private to the package.
Example: Exporting and Importing in Go
Here’s a basic example demonstrating how to create a package and import it.
In the calculator
package (in the calculator
directory):
// file: calculator.go
package calculator
func Add(a int, b int) int {
return a + b
}
In the main application (in the root directory):
// file: main.go
package main
import (
"fmt"
"example.com/myapp/calculator"
)
func main() {
result := calculator.Add(3, 4)
fmt.Println("The sum is:", result)
}
In the example above, the calculator
package contains an exported Add
function, and the main program imports this package and uses the function.
Creating and Organizing Packages
In Go, creating a package is straightforward. Every Go source file starts with a package
declaration, and all files in the same directory should use the same package name. Let’s build a package and see how we can structure it effectively.
Imagine we are creating a math utility library with two packages: mathutils
and formatter
.
mathutils
Package
In the mathutils
directory, you might have a file like this:
// file: mathutils.go
package mathutils
func Multiply(x int, y int) int {
return x * y
}
formatter
Package
In the formatter
directory, you can create a formatting function:
// file: formatter.go
package formatter
import "fmt"
func FormatOutput(result int) string {
return fmt.Sprintf("The result is: %d", result)
}
The Main Program
Finally, in the root of your project, you’d use these packages in your main.go
file:
// file: main.go
package main
import (
"fmt"
"example.com/myapp/formatter"
"example.com/myapp/mathutils"
)
func main() {
result := mathutils.Multiply(5, 10)
formattedOutput := formatter.FormatOutput(result)
fmt.Println(formattedOutput)
}
When you run this program, the output will be:
$ go run main.go
The result is: 50
Package Naming and Best Practices
Avoid Ambiguous Package Names
Go requires every package to have a unique name in the import path. A common mistake is creating packages with generic names like utils
. Instead, your package name should describe its purpose clearly. For instance, instead of:
package utils
Use:
package stringutils
This clarity makes it easier to understand what each package does, especially when reading code that imports and uses these packages.
Name Functions Clearly
It’s crucial to avoid redundant naming inside your packages. If your package is named mathutils
, don’t create a function named MathMultiply
. Instead, just use Multiply
—the package name already provides context.
For example:
package mathutils
func Multiply(a int, b int) int {
return a * b
}
This function would be referred to as mathutils.Multiply
in your code, which is much cleaner.
Conclusion
Go’s package system is simple but powerful, enabling developers to create modular, maintainable codebases. By adhering to best practices such as using clear package names, understanding the rules of imports, and properly structuring packages, you can ensure your Go projects remain scalable and easy to maintain.
If you’re starting with Go or trying to better organize your code, these principles are essential for writing clean, effective Go programs.