The Power of Maps in JavaScript: Benefits and How to Use Them
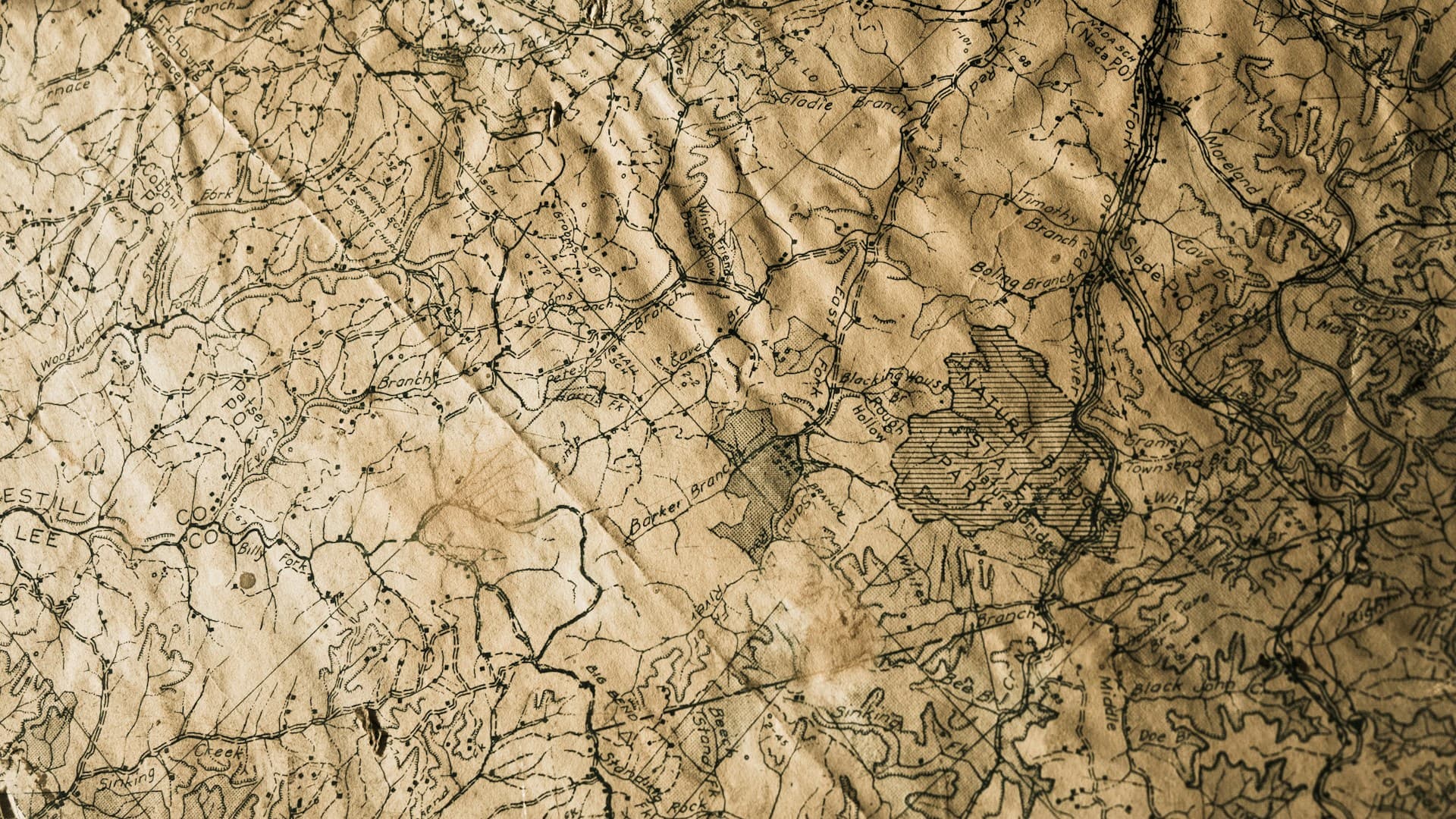
Table of Content
- Benefits of Using Maps
- Flexible Key Types
- Maintains Key Order
- Improved Performance with Large Datasets
- Built-in Size Property
- Intuitive Methods
- Creating and Using Maps
- Basic Map Operations
- Creating a Map
- Adding Key-Value Pairs
- Retrieving Values
- Checking if a Key Exists
- Removing Entries
- Checking the Map Size
- Iterating Over Maps
- Using a `for...of` Loop
- Using `forEach`
- Converting to Arrays
- Common Use Cases for Maps
- 1. Counting Occurrences
- 2. Caching or Memoization
- 3. Associating Metadata with Objects
- When to Use (and Not Use) Maps
- Use a Map When:
- Use an Object When:
- Avoid Maps When:
- Using Maps with TypeScript
- Declaring a Map with Specific Types
- Using Complex Types as Keys
- Conclusion
JavaScript offers several options for data storage, but for specific use cases, the Map object introduced in ECMAScript 6 (ES6) provides a flexible and efficient alternative to traditional objects. In this article, we’ll dive into the benefits of using Maps in JavaScript and explore some practical use cases and examples.
Benefits of Using Maps
Flexible Key Types
Unlike objects, which restrict keys to strings or symbols, a Map can use any data type as a key—including functions, objects, and even other maps. This flexibility makes Maps suitable for complex data structures or scenarios where non-string keys are needed.
Maintains Key Order
Map entries are stored in the order they’re added, unlike objects which don’t guarantee key order. This feature is especially useful for scenarios that require predictable iteration.
Improved Performance with Large Datasets
Maps are optimized for frequent addition and deletion of entries, making them better than plain objects when working with large datasets or dynamically changing collections.
Built-in Size Property
Maps come with a .size
property that instantly provides the count of key-value pairs. This is more efficient than objects, where calculating size requires iterating over keys.
Intuitive Methods
Maps offer built-in methods like .set()
, .get()
, .has()
, .delete()
, and .clear()
that make the code more readable and reduce the need for workarounds.
Creating and Using Maps
Let’s look at some common operations with Maps, along with practical examples.
Basic Map Operations
Creating a Map
const userRoles = new Map();
Adding Key-Value Pairs
userRoles.set("admin", "Read and Write Access");
userRoles.set("editor", "Read Access Only");
Retrieving Values
console.log(userRoles.get("admin")); // Output: "Read and Write Access"
Checking if a Key Exists
console.log(userRoles.has("viewer")); // Output: false
Removing Entries
userRoles.delete("editor");
userRoles.clear(); // Clears all entries
Checking the Map Size
console.log(userRoles.size); // Output: 0 after clear()
Iterating Over Maps
Maps provide multiple ways to iterate over entries.
Using a for...of
Loop
const mapExample = new Map([
["apple", 2],
["banana", 5],
["orange", 3],
]);
for (let [key, value] of mapExample) {
console.log(`${key}: ${value}`);
}
Using forEach
mapExample.forEach((value, key) => {
console.log(`${key}: ${value}`);
});
Converting to Arrays
Maps can be converted into arrays, allowing use of array methods such as .map()
and .filter()
.
const entries = Array.from(mapExample);
console.log(entries); // Output: [["apple", 2], ["banana", 5], ["orange", 3]]
Common Use Cases for Maps
1. Counting Occurrences
Maps can efficiently count occurrences, such as word frequencies in a text:
const text = "this is a sample text and this is just a sample";
const wordCount = new Map();
text.split(" ").forEach((word) => {
wordCount.set(word, (wordCount.get(word) || 0) + 1);
});
console.log(wordCount);
2. Caching or Memoization
Maps are ideal for caching, especially when functions may be called with the same arguments multiple times.
const factorialCache = new Map();
function factorial(n) {
if (n === 0) return 1;
if (factorialCache.has(n)) return factorialCache.get(n);
let result = n * factorial(n - 1);
factorialCache.set(n, result);
return result;
}
3. Associating Metadata with Objects
You can associate metadata with objects by using the object as the key in a map.
const userPreferences = new Map();
const user1 = { name: "Alice" };
const user2 = { name: "Bob" };
userPreferences.set(user1, { theme: "dark", notifications: true });
userPreferences.set(user2, { theme: "light", notifications: false });
console.log(userPreferences.get(user1)); // Output: { theme: 'dark', notifications: true }
When to Use (and Not Use) Maps
Use a Map When:
- Keys are non-strings: Maps allow any data type as keys, unlike objects.
- Insertion order matters: Maps maintain key insertion order.
- Frequent additions/removals: Maps are optimized for these operations.
- You need size tracking: Maps have a
.size
property. - Iteration is key: Maps are natively iterable.
Use an Object When:
- Simple string-keyed data: For straightforward key-value pairs with string keys.
- JSON serialization is required: Objects can easily be serialized with JSON.
- Prototypal inheritance is needed: Objects support inheritance, while Maps do not.
Avoid Maps When:
- Simple data structure with string keys: Objects are typically more efficient for simple use cases.
- Serialization needs: Maps lack native support for JSON serialization.
Using Maps with TypeScript
In TypeScript, you can specify the types for both keys and values when creating a Map, enhancing type safety.
Declaring a Map with Specific Types
const ageMap: Map<string, number> = new Map();
Using Complex Types as Keys
interface User {
id: number;
name: string;
}
const userRoles: Map<User, string> = new Map();
const user1 = { id: 1, name: "Alice" };
userRoles.set(user1, "Admin");
TypeScript helps avoid errors by enforcing type compatibility, making Maps a powerful option in type-safe environments.
Conclusion
The Map object in JavaScript is a powerful data structure, offering flexibility, performance, and convenience. Whether you need non-string keys, predictable order, or frequent modification, Maps provide advantages over traditional objects. With these methods and use cases, you can enhance your JavaScript applications with efficient and well-organized data handling.