Mastering Memoization in React.js: Optimizing with useMemo, useCallback, and React.memo
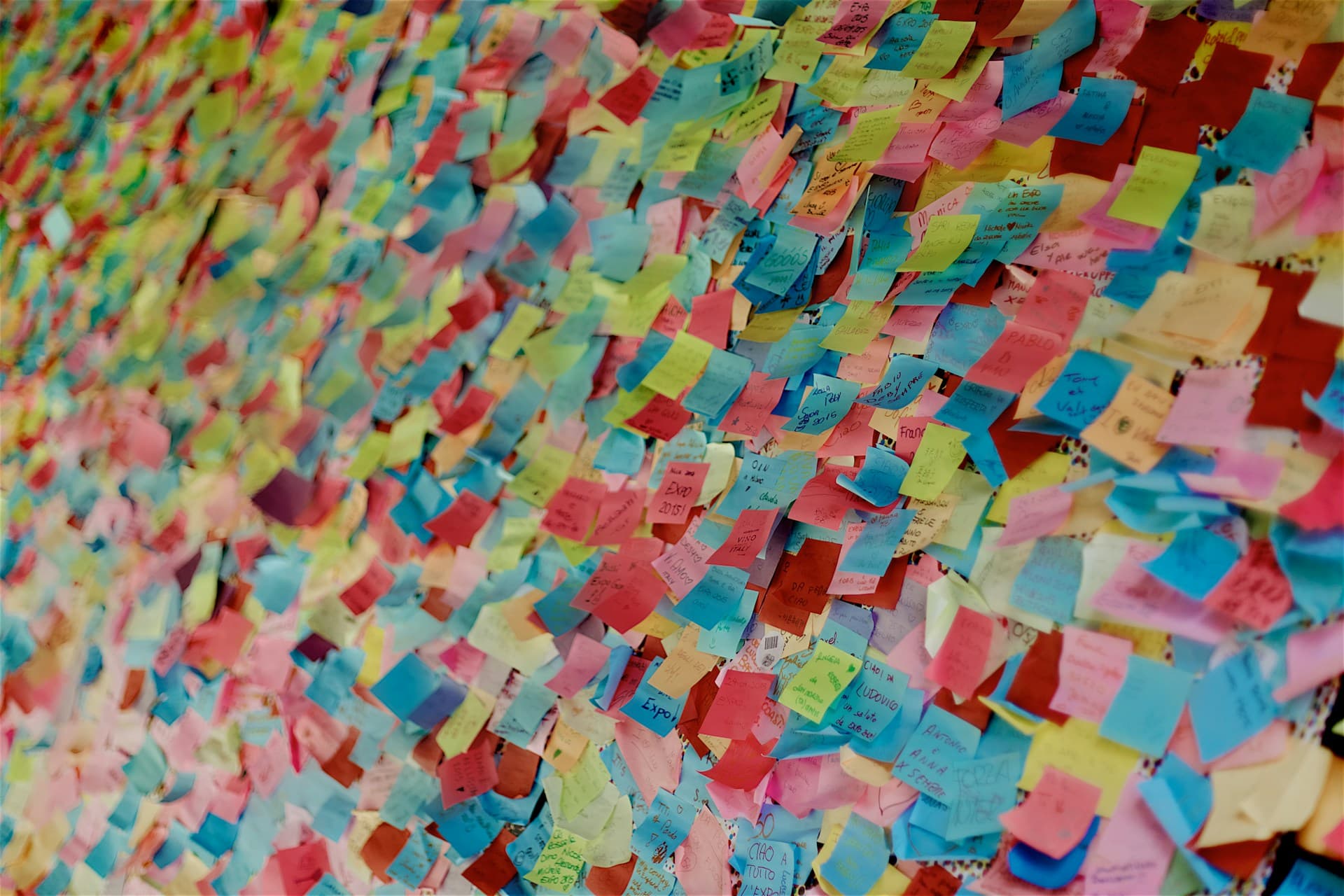
Table of Content
- 1. useMemo Hook
- Purpose
- Syntax
- When to Use useMemo
- 2. useCallback Hook
- Syntax
- Example Scenario: Passing Functions as Props
- When to Use useCallback
- 3. React.memo Higher-Order Component
- Syntax
- Example Scenario: Preventing Re-renders of Unchanged List Items
- When to Use React.memo
- Real-World Example: Combining useMemo, useCallback, and React.memo
- Conclusion
As applications grow in complexity, performance becomes increasingly important, especially in React, where even slight inefficiencies in rendering can lead to noticeable lag. Memoization, a technique for caching calculations and preserving references, is a key strategy React developers can use to avoid unnecessary renders and computations.
React provides three main tools for memoization: useMemo
, useCallback
, and React.memo
. Each has specific purposes and usage scenarios. This article will cover each of these tools, how they work, and when to use them to optimize your applications by preventing re-renders and recalculations. We'll also dive into practical examples showing where multiple re-renders would happen without memoization and how each hook addresses those issues.
Understanding Re-renders in React
Before we dive into the memoization hooks, let’s briefly review why and when React components re-render. React’s default behavior is to re-render components whenever their state or props change. While this approach keeps the UI in sync with data, it can lead to performance issues when:
- A component re-renders due to an unrelated state change in a parent.
- Expensive computations are re-run on every render.
- Functions or objects passed as props trigger child re-renders because their references change every render.
Memoization solves these issues by caching results or references so that React knows when a value or function hasn't actually changed, thus preventing needless re-renders.
1. useMemo Hook
Purpose
The useMemo
hook is used to memoize the return value of a function. This is particularly useful for caching the results of expensive calculations that should not be re-run on every render. It only re-computes the value when one of its dependencies changes.
Syntax
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
- The function passed as the first argument is your expensive calculation.
- The dependency array (
[a, b]
) tells React to re-run this calculation only whena
orb
changes.
Example Scenario: Expensive Computations
Imagine a component that performs a heavy computation, like generating a large data structure or performing intensive math calculations. Without useMemo, this computation would run on every render, potentially slowing down the UI.
import React, { useMemo, useState } from "react";
function ExpensiveComponent({ data }) {
const [counter, setCounter] = useState(0);
const computeStatistics = (data) => {
console.log("Computing statistics...");
return data.reduce((acc, value) => acc + value, 0) / data.length;
};
const average = useMemo(() => computeStatistics(data), [data]);
return (
<div>
<button onClick={() => setCounter(counter + 1)}>
Increment: {counter}
</button>
<p>Average: {average}</p>
</div>
);
}
In this example:
Without useMemo, computeStatistics would run every time the component re-renders (i.e., when the counter changes). With useMemo, computeStatistics only re-runs when data changes, optimizing the component's performance significantly.
When to Use useMemo
Use useMemo for caching the results of pure, expensive computations. Avoid using it for lightweight computations; the overhead of useMemo can sometimes outweigh the benefits in simple cases.
2. useCallback Hook
Purpose
useCallback is similar to useMemo, but instead of caching a computed value, it caches a function. This is helpful when passing functions to child components, as React redefines functions on every render. Without useCallback, these newly defined functions would cause child components to re-render, even if the actual functionality hasn’t changed.
Syntax
const memoizedCallback = useCallback(() => {
doSomething(a, b);
}, [a, b]);
Example Scenario: Passing Functions as Props
Imagine a parent component that passes a function as a prop to a child. If that function is redefined on each render, the child component will re-render even if the parent’s state or other values that don’t affect the child have changed.
import React, { useState, useCallback } from "react";
function ParentComponent() {
const [count, setCount] = useState(0);
const [text, setText] = useState("");
const handleIncrement = useCallback(() => {
setCount((c) => c + 1);
}, []);
return (
<div>
<input
value={text}
onChange={(e) => setText(e.target.value)}
placeholder="Type something..."
/>
<ChildComponent onIncrement={handleIncrement} />
</div>
);
}
function ChildComponent({ onIncrement }) {
console.log("ChildComponent rendered");
return <button onClick={onIncrement}>Increment</button>;
}
In this example:
Without useCallback, the handleIncrement function is redefined on each render of ParentComponent, causing ChildComponent to re-render. With useCallback, the same handleIncrement reference is used across renders, so ChildComponent doesn’t re-render unless necessary.
When to Use useCallback
Use useCallback to stabilize function references when passing functions as props to child components. Avoid using useCallback unless you encounter unnecessary re-renders due to changing function references.
3. React.memo Higher-Order Component
Purpose
React.memo is a higher-order component that memoizes the component itself. It prevents the component from re-rendering if its props haven’t changed, which can be especially beneficial for list items or components that re-render frequently due to parent state changes.
Syntax
const MemoizedComponent = React.memo(MyComponent);
Example Scenario: Preventing Re-renders of Unchanged List Items
Let’s look at a situation where we have a list of items in a parent component, each represented by a ListItem component. Without React.memo, changing any state in the parent component would cause every list item to re-render, even if their props haven’t changed.
import React, { useState } from "react";
const ListItem = React.memo(({ item }) => {
console.log("ListItem rendered:", item);
return <li>{item}</li>;
});
function ItemList() {
const [count, setCount] = useState(0);
const items = ["Item 1", "Item 2", "Item 3"];
return (
<div>
<button onClick={() => setCount(count + 1)}>
Increment Count: {count}
</button>
<ul>
{items.map((item) => (
<ListItem key={item} item={item} />
))}
</ul>
</div>
);
}
In this example:
Without React.memo, each ListItem component would re-render every time count changes in ItemList, even though the item prop hasn’t changed. With React.memo, each ListItem only re-renders if its item prop changes, greatly improving performance by avoiding unnecessary re-renders.
When to Use React.memo
Use React.memo to wrap components that rely on props that don’t change frequently. Avoid using React.memo if your component frequently receives new props, as this can actually degrade performance due to shallow prop comparisons.
Real-World Example: Combining useMemo, useCallback, and React.memo
In complex applications, combining all three memoization techniques often yields the best results. Here’s an example where each tool is used for a specific purpose to avoid unnecessary re-renders:
- Parent Component uses useMemo to memoize data that changes infrequently.
- Parent Component uses useCallback to memoize a function passed to children.
- Child Component uses React.memo to prevent re-renders unless its props change.
import React, { useState, useMemo, useCallback } from "react";
const Child = React.memo(({ item, onAction }) => {
console.log("Rendering:", item);
return <button onClick={() => onAction(item)}>Action on {item}</button>;
});
function ParentComponent() {
const [count, setCount] = useState(0);
const data = useMemo(() => ["Item A", "Item B", "Item C"], []);
const handleAction = useCallback((item) => {
console.log("Action on:", item);
}, []);
return (
<div>
<button onClick={() => setCount((c) => c + 1)}>Increment</button>
{data.map((item) => (
<Child key={item} item={item} onAction={handleAction} />
))}
<p>Count: {count}</p>
</div>
);
}
In this example:
- useMemo is used to memoize data, so it won’t be recreated unless the dependency changes.
- useCallback memoizes handleAction, preventing re-renders of child components that use this function.
Conclusion
Memoization is a powerful optimization strategy that can significantly improve the performance of React applications by preventing unnecessary re-renders. By using useMemo
, useCallback
, and React.memo
, you can:
- Reduce rendering costs by caching expensive computations with
useMemo
. - Stabilize function references with
useCallback
, avoiding unwanted re-renders in child components. - Control component rendering with
React.memo
, ensuring only components with updated props re-render.
Key Takeaway: Use memoization selectively and thoughtfully. While it’s a valuable tool, overuse or incorrect implementation may introduce complexity without real benefits. Start with identifying components experiencing performance issues and apply memoization only where it yields tangible improvements.
By combining these techniques, you create a smoother, more efficient user experience, especially as your application scales. Mastering these memoization hooks will make you a more proficient React developer, equipped to handle complex performance optimizations.