Mastering Type Narrowing in TypeScript: A Comprehensive Guide
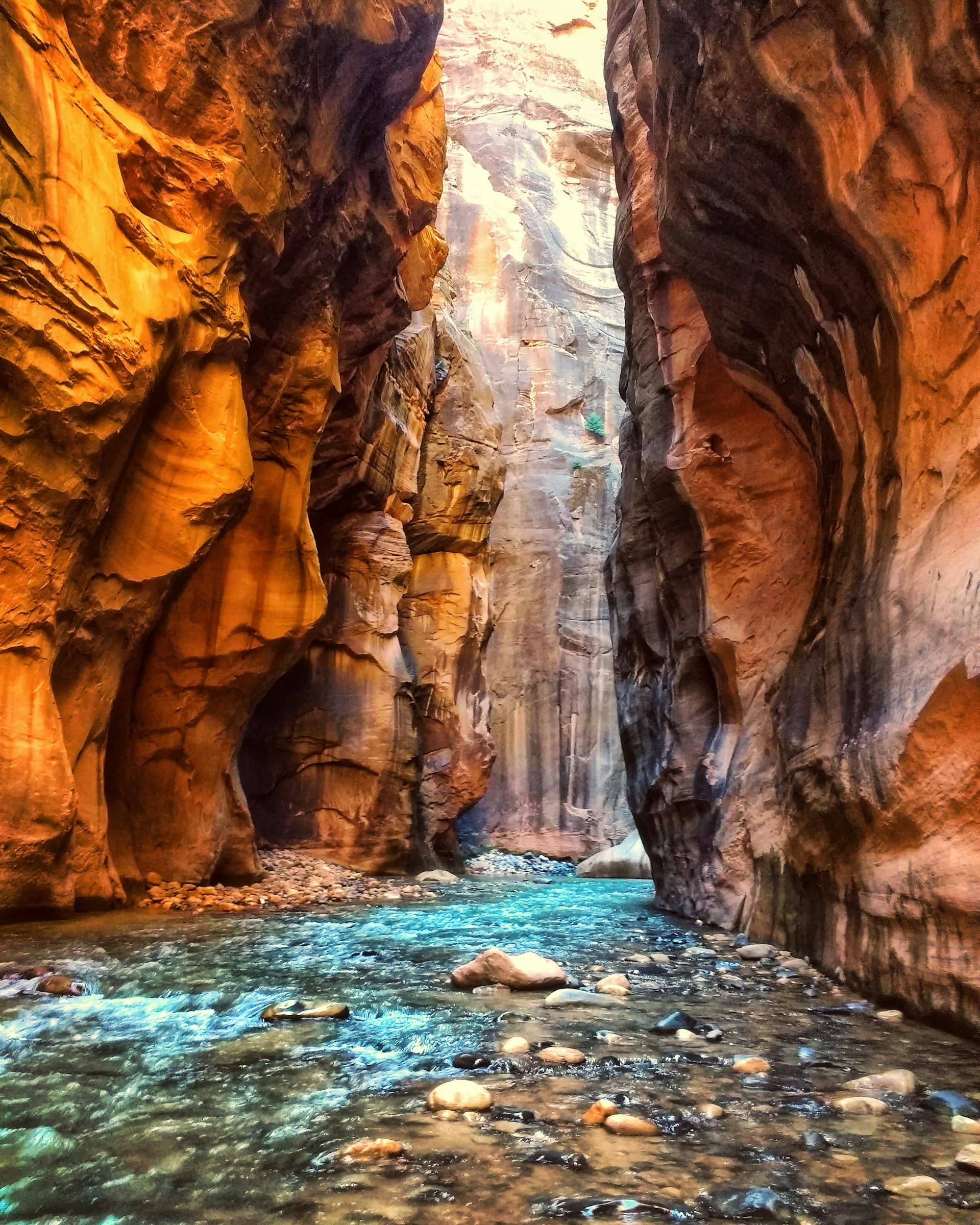
Table of Content
- What is Type Narrowing?
- Example:
- Why is Type Narrowing Important?
- Key Benefits:
- Techniques for Type Narrowing
- **1. Using `typeof`**
- **2. Using `instanceof`**
- **3. Using Equality Checks**
- **4. Using Type Predicates**
- **5. Using `in` Operator**
- **6. Discriminated Unions**
- Best Practices for Type Narrowing
- Example:
- Conclusion
TypeScript introduces powerful static type-checking capabilities that improve code quality and developer productivity. Among its standout features is type narrowing, a technique to refine and determine the type of a variable at runtime. This guide explores the concept of type narrowing, its importance, and how to apply it effectively in your projects.
What is Type Narrowing?
Type narrowing refers to refining a variable's type to a more specific type within a scope. TypeScript achieves this by analyzing control flow and using type guards or assertions. By narrowing a variable's type, developers gain confidence about the operations and methods that can safely be performed.
Example:
function process(value: string | number) {
if (typeof value === "string") {
console.log(value.toUpperCase()); // TypeScript knows `value` is a string here.
} else {
console.log(value.toFixed(2)); // TypeScript knows `value` is a number here.
}
}
Here, the typeof
operator helps TypeScript narrow value
to either string
or number
based on the condition.
Why is Type Narrowing Important?
TypeScript’s strict type system prevents many runtime errors but can introduce ambiguity with union types like string | number
. Type narrowing resolves this ambiguity, enabling safer and more precise code.
Key Benefits:
- Enhanced Safety: Avoid runtime errors by ensuring variables are correctly typed.
- Improved Readability: Conditions double as documentation for handling different types.
- Reduced Type Assertions: Minimize explicit casting (
as
) by letting TypeScript infer types naturally.
Techniques for Type Narrowing
1. Using typeof
The typeof
operator is commonly used for primitive types like string
, number
, boolean
, and symbol
.
function describe(value: string | number | boolean) {
if (typeof value === "string") {
console.log(`String: ${value.toUpperCase()}`);
} else if (typeof value === "number") {
console.log(`Number: ${value.toFixed(2)}`);
} else {
console.log(`Boolean: ${value ? "true" : "false"}`);
}
}
2. Using instanceof
The instanceof
operator narrows types to specific classes or object types.
class Dog {
bark() {
console.log("Woof!");
}
}
class Cat {
meow() {
console.log("Meow!");
}
}
function interact(animal: Dog | Cat) {
if (animal instanceof Dog) {
animal.bark();
} else {
animal.meow();
}
}
3. Using Equality Checks
Equality checks are useful for narrowing union types with literal values.
function checkStatus(status: "success" | "error" | "pending") {
if (status === "success") {
console.log("Operation succeeded!");
} else if (status === "error") {
console.log("An error occurred.");
} else {
console.log("Operation is still pending.");
}
}
4. Using Type Predicates
Custom type guards return a boolean, helping TypeScript infer narrowed types.
interface Fish {
swim: () => void;
}
interface Bird {
fly: () => void;
}
function isFish(animal: Fish | Bird): animal is Fish {
return (animal as Fish).swim !== undefined;
}
function interactWithAnimal(animal: Fish | Bird) {
if (isFish(animal)) {
animal.swim(); // TypeScript knows this is a Fish.
} else {
animal.fly(); // TypeScript knows this is a Bird.
}
}
5. Using in
Operator
The in
operator checks for property existence and narrows types based on it.
interface Car {
drive: () => void;
}
interface Plane {
fly: () => void;
}
function operate(vehicle: Car | Plane) {
if ("drive" in vehicle) {
vehicle.drive();
} else {
vehicle.fly();
}
}
6. Discriminated Unions
Discriminated unions use a common property (a "tag") to differentiate between types.
interface Circle {
kind: "circle";
radius: number;
}
interface Rectangle {
kind: "rectangle";
width: number;
height: number;
type Shape = Circle | Rectangle;
function calculateArea(shape: Shape) {
if (shape.kind === "circle") {
return Math.PI * shape.radius ** 2;
} else {
return shape.width * shape.height;
}
}
Best Practices for Type Narrowing
- Leverage Control Flow Analysis: TypeScript often eliminates the need for explicit assertions.
- Keep Checks Simple: Write clear and readable conditions for easy understanding.
- Avoid Over-Narrowing: Over-complicating narrowing logic can make code harder to maintain.
- Use Exhaustive Checks: Ensure all possible cases are handled using
never
.
Example:
function handleShape(shape: Shape) {
switch (shape.kind) {
case "circle":
console.log("Circle area:", Math.PI * shape.radius ** 2);
break;
case "rectangle":
console.log("Rectangle area:", shape.width * shape.height);
break;
default:
const _exhaustiveCheck: never = shape; // Error if new shape is not handled.
throw new Error("Unhandled shape!");
}
}
Conclusion
Type narrowing is an indispensable feature in TypeScript, enabling developers to handle complex types safely and effectively. By combining techniques like typeof
, instanceof
, custom type guards, and discriminated unions, you can write expressive, type-safe code that is both robust and maintainable.
Embrace type narrowing to unlock the full potential of TypeScript, and build applications that are safer and more reliable.