Mastering TypeScript's reduce with Explicit Generic Type Arguments
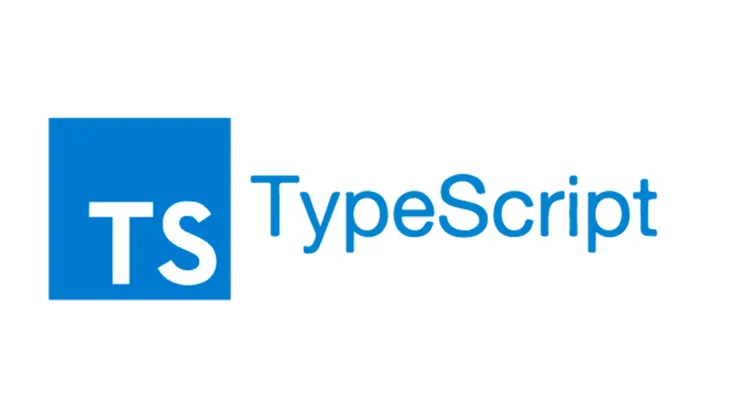
TypeScript’s reduce
method is a powerful tool for transforming arrays into a single accumulated value. When the type of the accumulator differs from that of the array elements, TypeScript’s inference can sometimes need a helping hand. In this article, we’ll explore how to explicitly pass type arguments to reduce
so that your accumulator is correctly typed, ensuring both type safety and clarity in your code.
Understanding
reduce
's Type Signatures
The reduce
function comes with multiple overloads. Here’s a simplified look at its type signatures:
Without an Initial Value
When no initial value is provided, the accumulator’s type is assumed to be the same as the array element’s type:
reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T): T;
With an Initial Value Matching the Array Element
If you supply an initial value that matches the array’s element type, TypeScript continues to assume both are the same:
reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T, initialValue: T): T;
With an Initial Value of a Different Type
When the accumulator should be of a different type, you can let TypeScript know by providing a generic type argument:
reduce<U>(callbackfn: (previousValue: U, currentValue: T, currentIndex: number, array: T[]) => U, initialValue: U): U;
Here, the generic parameter <U>
represents the type of the accumulator, which is inferred from the type of the initial value you supply.
A Practical Example: Converting an Array of Users into a RecordImagine you have an array of user objects and you want to transform it into a record (or dictionary) where each key is a user's unique identifier and the value is the user object. First, define a simple
User
interface and an array of users:
interface User {
id: string;
username: string;
age: number;
}
const users: User[] = [
{ id: "u1", username: "alice", age: 30 },
{ id: "u2", username: "bob", age: 25 },
];
Since the accumulator will be a record with string keys and User
values (Record<string, User>
), we need to instruct TypeScript accordingly. There are several ways to do this.
Option 1: Passing a Generic Type Argument Directly
You can explicitly specify the accumulator’s type by providing the generic type argument to reduce
:
const userRecord = users.reduce<Record<string, User>>((acc, user) => {
acc[user.id] = user;
return acc;
}, {});
In this example, Record<string, User>
tells TypeScript that the accumulator is an object whose keys are strings and whose values are of type User
.
Option 2: Asserting the Type of the Initial Value
Another approach is to use a type assertion on the initial value:
const userRecord = users.reduce(
(acc, user) => {
acc[user.id] = user;
return acc;
},
{} as Record<string, User>,
);
By asserting that the initial empty object is of type Record<string, User>
, TypeScript infers the correct accumulator type throughout the reduction.
Option 3: Annotating the Accumulator Parameter
A third option is to annotate the accumulator parameter in the callback function:
const userRecord = users.reduce((acc: Record<string, User>, user) => {
acc[user.id] = user;
return acc;
}, {});
This inline annotation provides an immediate hint about the type of acc
and ensures the return value of the callback matches the expected type.
Why Explicit Type Arguments MatterWhen the initial value is ambiguous—such as an empty object (
{}
)—TypeScript may struggle to infer the intended type. Explicitly providing the accumulator’s type through one of the methods above helps prevent unexpected behavior, catches potential bugs at compile time, and makes your code more self-documenting.
Conclusion
Passing explicit generic type arguments to the reduce
function is an effective way to leverage TypeScript’s strong typing system, particularly when transforming an array into a different structure. Whether you choose to use a generic type parameter, a type assertion, or direct parameter annotation, you can ensure that your code remains clear, concise, and type-safe.
Happy coding in TypeScript!