Mastering the File System Module in Node.js: Essential Techniques and Examples
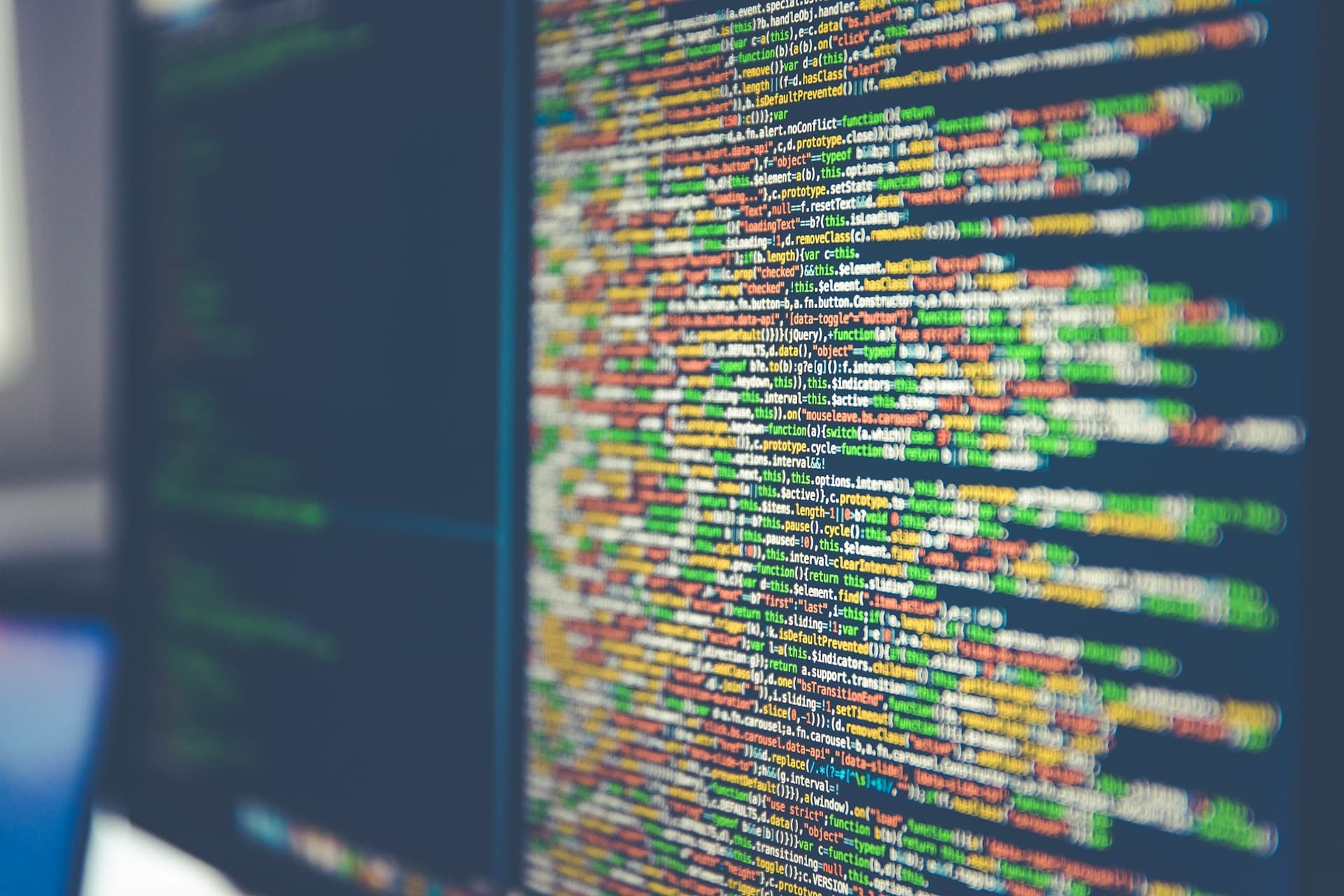
Table of Content
- Importing the fs Module
- Synchronous vs. Asynchronous Methods
- Key Methods in the fs Module
- 1. Reading Files
- Asynchronous: `fs.readFile()`
- Synchronous: `fs.readFileSync()`
- 2. Writing Files
- Asynchronous: `fs.writeFile()`
- Synchronous: `fs.writeFileSync()`
- 3. Appending Data to Files
- Asynchronous: `fs.appendFile()`
- Synchronous: `fs.appendFileSync()`
- 4. Deleting Files
- Asynchronous: `fs.unlink()`
- Synchronous: `fs.unlinkSync()`
- 5. Creating Directories
- Asynchronous: `fs.mkdir()`
- Synchronous: `fs.mkdirSync()`
- 6. Removing Directories
- Asynchronous: `fs.rmdir()`
- Synchronous: `fs.rmdirSync()`
- 7. Renaming Files or Directories
- Asynchronous: `fs.rename()`
- Synchronous: `fs.renameSync()`
- Watching Files for Changes
- File Permissions and Access Checking
- Promises API in the fs Module
- Conclusion
- LinkedIn Copy
The File System (fs
) module is a core module in Node.js that provides powerful methods to interact with the server's file system. This module allows developers to read, write, update, delete, and monitor files and directories with ease. It includes both synchronous and asynchronous methods, making it versatile for a range of applications that require file manipulation.
In this article, we’ll dive into the fs
module, covering its most frequently used methods and providing practical examples to help you get started.
Importing the fs Module
Since fs
is part of Node.js’s core, there’s no need to install it separately. Simply import it into your project using the require
function:
const fs = require("fs");
Synchronous vs. Asynchronous Methods
Most fs
functions are available in two forms:
- Synchronous methods: Block the code execution until the operation completes.
- Asynchronous methods: Non-blocking, these methods work with callbacks or promises, ideal for handling multiple operations concurrently.
Key Methods in the fs Module
Here’s an overview of the most commonly used fs
methods.
1. Reading Files
Asynchronous: fs.readFile()
Reads a file’s content asynchronously.
fs.readFile("example.txt", "utf8", (err, data) => {
if (err) throw err;
console.log(data);
});
Synchronous: fs.readFileSync()
The synchronous alternative for file reading.
const data = fs.readFileSync("example.txt", "utf8");
console.log(data);
Using 'utf8'
encoding reads the file as a string. Without it, the data is returned as a Buffer object.
2. Writing Files
Asynchronous: fs.writeFile()
Writes data to a file, creating it if it doesn’t exist or overwriting it if it does.
fs.writeFile("example.txt", "Hello, World!", (err) => {
if (err) throw err;
console.log("File written successfully");
});
Synchronous: fs.writeFileSync()
The synchronous version of file writing.
fs.writeFileSync("example.txt", "Hello, World!");
console.log("File written successfully");
Tip: Use fs.appendFile()
to add data to an existing file without overwriting.
3. Appending Data to Files
Asynchronous: fs.appendFile()
fs.appendFile('example.txt', '
New Line of Text', (err) => {
if (err) throw err;
console.log('Data appended to file');
});
Synchronous: fs.appendFileSync()
fs.appendFileSync('example.txt', '
New Line of Text');
console.log('Data appended to file');
4. Deleting Files
Asynchronous: fs.unlink()
fs.unlink("example.txt", (err) => {
if (err) throw err;
console.log("File deleted");
});
Synchronous: fs.unlinkSync()
fs.unlinkSync("example.txt");
console.log("File deleted");
5. Creating Directories
Asynchronous: fs.mkdir()
fs.mkdir("newFolder", { recursive: true }, (err) => {
if (err) throw err;
console.log("Directory created");
});
Synchronous: fs.mkdirSync()
fs.mkdirSync("newFolder", { recursive: true });
console.log("Directory created");
Setting { recursive: true }
allows nested directories to be created if needed.
6. Removing Directories
Asynchronous: fs.rmdir()
This function removes only empty directories. For directories with content, use fs.rm()
with { recursive: true }
.
fs.rmdir("newFolder", (err) => {
if (err) throw err;
console.log("Directory removed");
});
Synchronous: fs.rmdirSync()
fs.rmdirSync("newFolder");
console.log("Directory removed");
7. Renaming Files or Directories
Asynchronous: fs.rename()
fs.rename("oldName.txt", "newName.txt", (err) => {
if (err) throw err;
console.log("File renamed");
});
Synchronous: fs.renameSync()
fs.renameSync("oldName.txt", "newName.txt");
console.log("File renamed");
Watching Files for Changes
Using fs.watch()
allows you to monitor a file or directory. When a change is detected, an event is triggered.
fs.watch("example.txt", (eventType, filename) => {
console.log(`Event Type: ${eventType}`);
console.log(`Filename: ${filename}`);
});
File Permissions and Access Checking
The fs
module also provides methods for checking and modifying file permissions.
fs.access()
: Checks if a file or directory exists and if you have the required permissions.
fs.access("example.txt", fs.constants.F_OK, (err) => {
console.log(`${err ? "File does not exist" : "File exists"}`);
});
fs.chmod()
andfs.chown()
: Modify the permissions and ownership of files.
Promises API in the fs Module
Starting with Node.js v10, the fs
module introduced Promise-based methods that support async/await
, streamlining asynchronous operations without callbacks. To use them, import fs/promises
:
const fsPromises = require("fs/promises");
(async () => {
try {
const data = await fsPromises.readFile("example.txt", "utf8");
console.log(data);
} catch (err) {
console.error("Error reading file:", err);
}
})();
The Promises API simplifies code and makes it easier to read and maintain.
Conclusion
The fs module in Node.js is indispensable for applications that manage files or directories. It enables data persistence, file manipulation, and access control on the server. With both asynchronous and synchronous methods, fs
provides the flexibility needed to work efficiently with the file system, making it a core element of many Node.js projects.
Mastering these methods will allow you to build robust applications that can interact seamlessly with the filesystem, laying a foundation for efficient backend development.
LinkedIn Copy
🔹 Mastering File Manipulation in Node.js 🔹
Want to interact with files and directories in your Node.js applications? The fs
module in Node.js is packed with methods for reading, writing, deleting, and monitoring files and directories. Dive into this article to see key fs
methods explained with examples, from basic file operations to advanced file watching and permissions handling.
Discover how to work with both synchronous and asynchronous methods, and take advantage of the Promises API for clean, readable code. Perfect for anyone aiming to enhance their backend skills! 🚀
#NodeJS #BackendDevelopment #ProgrammingTips #JavaScript #FileSystem