Understanding Go's Module System: Repositories, Modules, and Packages
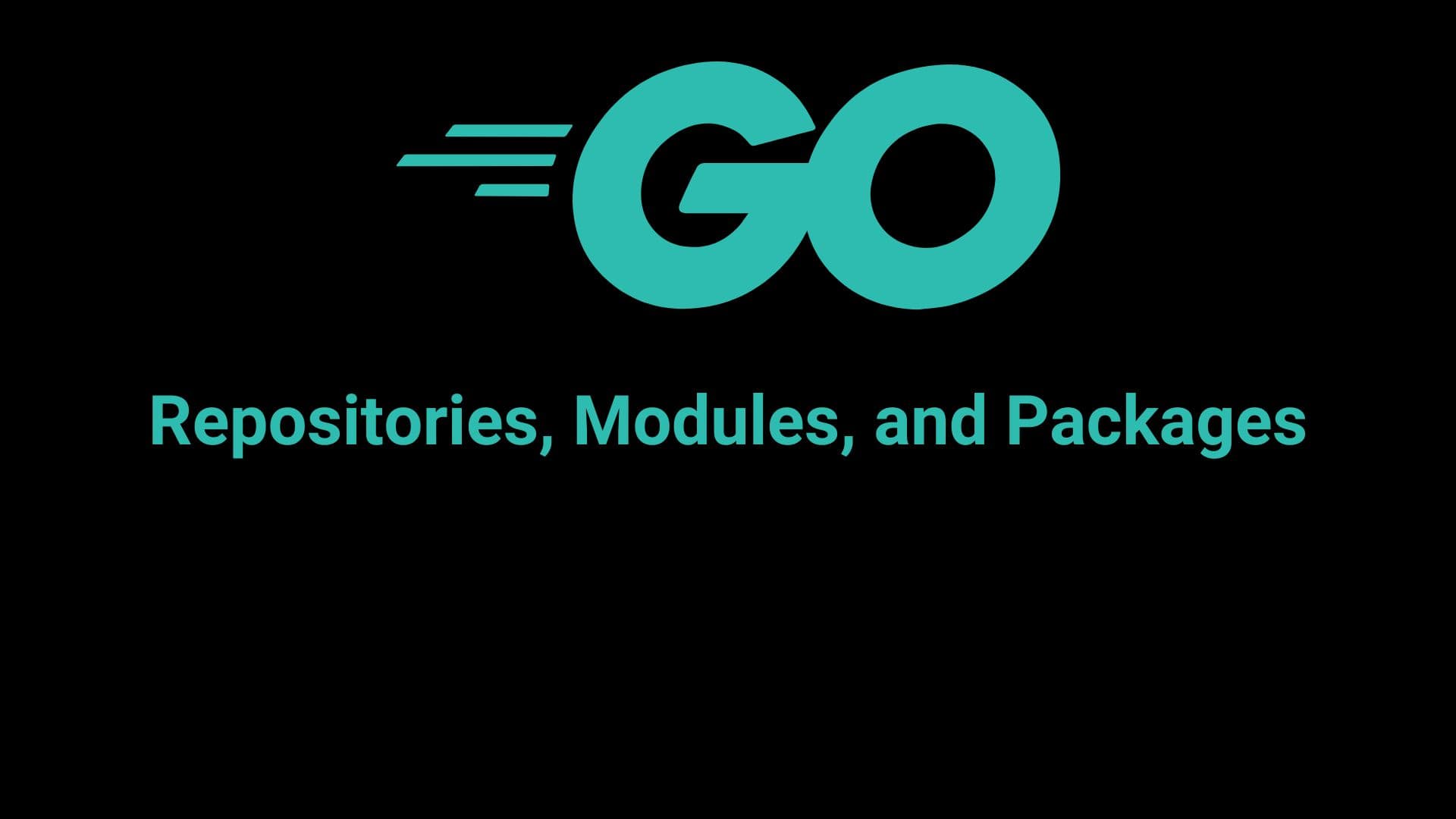
Table of Content
- Repositories, Modules, and Packages: The Foundation
- The Relationship Between Repositories, Modules, and Packages
- Creating and Working with Modules
- Declaring a New Module
- Example go.mod File:
- Managing Dependencies
- Packages: Organizing Code Within a Module
- Creating a Package
- add.go (in the `mathutils` package):
- subtract.go (in the `mathutils` package):
- Using the `mathutils` Package:
- Versioning and Releasing Modules
- go.mod File in Depth
- Key Sections of go.mod:
- Conclusion
- Key Takeaways:
Go's module system is an integral part of how the language organizes, manages, and shares code across projects. This system is built around three key concepts: repositories, modules, and packages. Understanding how these concepts interact allows Go developers to effectively manage dependencies and organize their code into reusable components.
Repositories, Modules, and Packages: The Foundation
Before diving into Go-specific details, let's start with a few basic definitions:
- Repository: A repository is where source code is stored in a version control system (VCS) like Git. A repository may hold code for multiple modules, but this is generally discouraged in Go.
- Module: A module is a collection of Go packages stored in a repository. It serves as the unit of versioning in Go, meaning that when you release new versions of your module, all the packages within that module are versioned together.
- Package: A package is a single unit of code organization within a module. It’s made up of one or more
.go
source files that are stored in the same directory and share the same package name.
The Relationship Between Repositories, Modules, and Packages
Think of it this way: A repository holds the code, a module represents a versioned collection of packages in that repository, and each package is a logical group of related code (types, functions, constants, etc.).
Example structure:
github.com/your-username/myproject # Repository
├── go.mod # Defines the module
├── pkg # Package directory
│ └── mathutils # Package
│ ├── add.go # Package file
│ └── subtract.go # Package file
└── main.go # Application entry point
In this example, the repository contains a Go module named github.com/your-username/myproject
, which includes the mathutils
package.
Creating and Working with Modules
Declaring a New Module
A module is declared by creating a go.mod
file in the root directory of your project. You don’t typically create this file manually; instead, Go provides the go mod
tool for handling this.
To initialize a new module, navigate to your project directory and run:
go mod init github.com/your-username/myproject
This command does two things:
- It creates a
go.mod
file in the current directory. - It declares the current directory as the root of a Go module and assigns the provided path (
github.com/your-username/myproject
) as the module path.
Example go.mod File:
module github.com/your-username/myproject
go 1.20
require (
github.com/sirupsen/logrus v1.8.1
github.com/shopspring/decimal v1.2.0
)
The module
directive specifies the unique path of your module, typically the URL where the module can be found (e.g., a GitHub repository).
The go
directive specifies the minimum Go version required to use the module. The require
section lists the other Go modules that your module depends on.
Managing Dependencies
Once your module is initialized, you can import third-party packages by referencing their module paths in your code. Go will automatically update your go.mod
file to reflect these dependencies.
For example, to use the decimal
package from the shopspring/decimal
module, you would import it in your Go code:
import "github.com/shopspring/decimal"
func main() {
price := decimal.NewFromFloat(19.99)
fmt.Println("Price:", price)
}
After running your program or go build
, Go will add the required module to your go.mod
file.
Packages: Organizing Code Within a Module
A package in Go is a collection of .go
files that are located in the same directory and share the same package name. Every Go program is made up of at least one package (the main
package), but larger applications typically consist of multiple packages.
Creating a Package
Let’s create a simple package called mathutils
in a module:
Directory structure:
github.com/your-username/myproject
├── go.mod
├── pkg
│ └── mathutils
│ ├── add.go
│ └── subtract.go
└── main.go
add.go (in the mathutils
package):
package mathutils
// Add returns the sum of two integers.
func Add(a, b int) int {
return a + b
}
subtract.go (in the mathutils
package):
package mathutils
// Subtract returns the difference between two integers.
func Subtract(a, b int) int {
return a - b
}
Using the mathutils
Package:
In main.go
, you can import and use the mathutils
package as follows:
package main
import (
"fmt"
"github.com/your-username/myproject/pkg/mathutils"
)
func main() {
sum := mathutils.Add(5, 3)
difference := mathutils.Subtract(5, 3)
fmt.Println("Sum:", sum)
fmt.Println("Difference:", difference)
}
Versioning and Releasing Modules
One of the major benefits of using Go modules is versioning. Every time you make changes to your module, you can release a new version by tagging the appropriate commit in your VCS (e.g., Git).
Example:
git tag v1.0.0
git push origin v1.0.0
Other projects that depend on your module can now update to version v1.0.0
by running:
go get github.com/your-username/myproject@v1.0.0
go.mod File in Depth
We've already seen some key elements of a go.mod
file. Here’s a breakdown:
Key Sections of go.mod:
- module: Declares the module path, which is typically the repository URL.
- go: Specifies the minimum Go version required.
- require: Lists the dependencies of your module, along with their minimum versions.
- replace: Optionally replaces a dependency with a different module or path.
- exclude: Prevents a specific version of a module from being used.
Example with replace
:
replace github.com/old/module v1.0.0 => github.com/new/module v1.2.0
This tells Go to use github.com/new/module v1.2.0
in place of github.com/old/module v1.0.0
.
Conclusion
Go’s module system offers a clean and powerful way to manage dependencies, organize code, and ensure your projects remain consistent across environments. By understanding how repositories, modules, and packages work together, you can build and share Go code more effectively.
Key Takeaways:
- A module is a collection of Go packages, versioned together and defined by a
go.mod
file. - Packages are Go’s way of organizing code into reusable components.
- The
go.mod
file is essential for managing dependencies and versioning in Go. - Use
go mod init
to create a new module andgo get
to manage external dependencies. - By adhering to Go's module system, you'll not only make your own life easier when managing projects, but you'll also make your code more accessible and usable by others in the Go ecosystem.