Understanding Literal Type Inference in TypeScript Generics: A Deep Dive
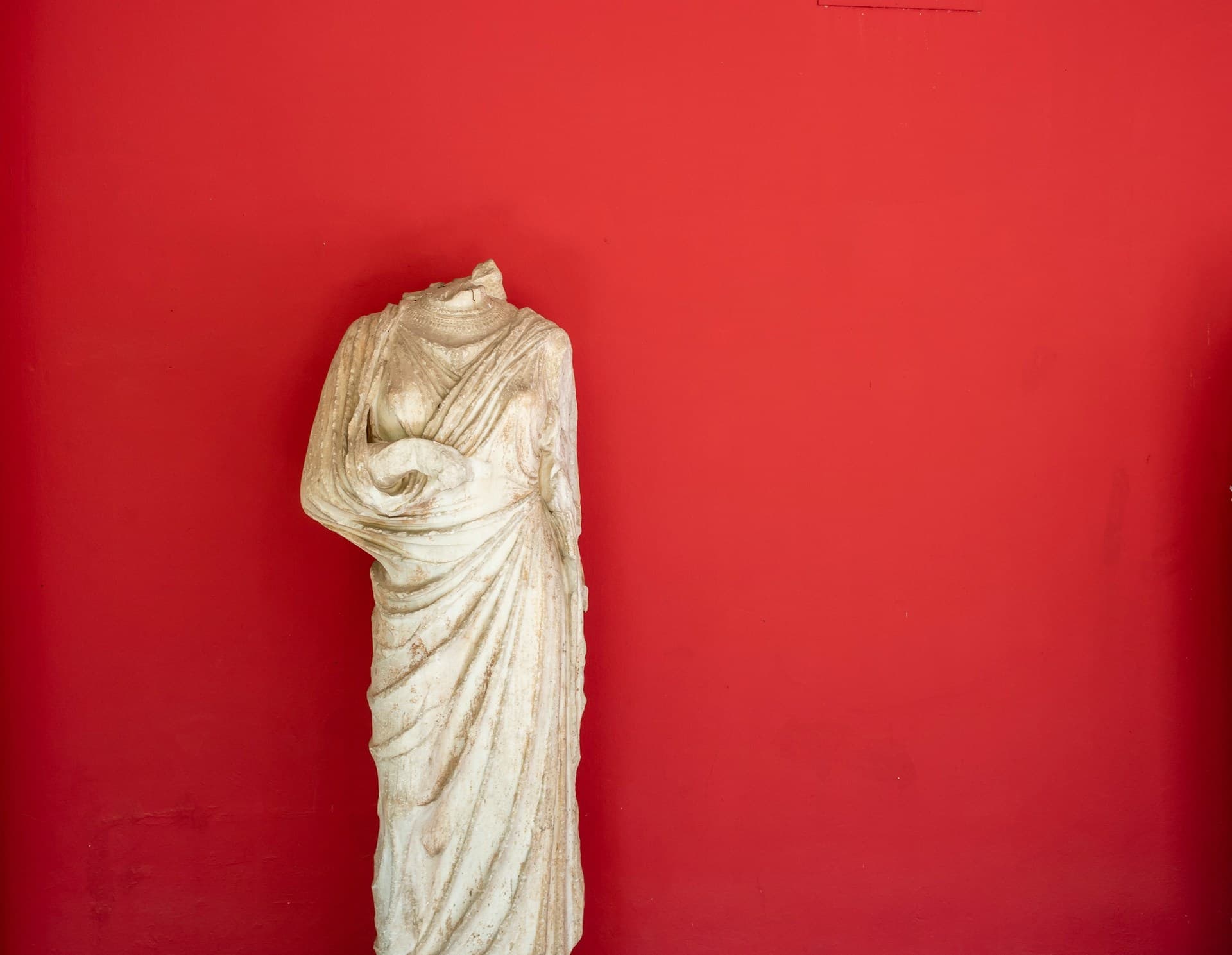
TypeScript’s generics empower us to write flexible and reusable code. However, one subtle nuance that can catch even experienced developers off guard is how literal types are inferred in generic functions. Depending on how you return your values, TypeScript may preserve the literal type or widen it to a more general type.
In this article, we’ll explore this behavior with fresh examples and learn how to guide TypeScript’s inference using constraints.
Direct Return of Values
Consider a simple generic function that returns the value passed in. Because the value is returned directly—without any additional wrapping—TypeScript preserves the literal type of the argument. Take a look at this example:
function identity<T>(input: T): T {
return input;
}
const directResult = identity("TypeScript");
// The inferred type of directResult is: "TypeScript"
Since the function directly returns the input, TypeScript infers the type of
directResult
as the literal"TypeScript"
. This is exactly what you might expect when dealing with a straightforward identity function.
Wrapping Values in an Object
Now, let’s see what happens when the value is returned inside an object. In this scenario, TypeScript widens the type instead of preserving the literal value:
function createBox<T>(input: T) {
return { content: input };
}
const boxedResult = createBox("Developer");
// The inferred type of boxedResult is: { content: string }
Even though we passed the literal
"Developer"
, the type ofboxedResult.content
is inferred asstring
rather than the specific literal"Developer"
. TypeScript makes this adjustment because wrapping the value in an object suggests that the property might later be changed or reassigned, so a broader type is considered more appropriate.
Using Constraints to Preserve Literal Types
If your goal is to maintain the literal type even when returning an object, you can leverage a type constraint on the generic parameter. For instance, by constraining the type to string
, TypeScript is forced to recognize and preserve the literal nature of the input:
function createTypedBox<T extends string>(input: T) {
return { content: input };
}
const typedBoxedResult = createTypedBox("Developer");
// The inferred type of typedBoxedResult is: { content: "Developer" }
With the constraint
T extends string
, TypeScript now understands thatinput
is always a string literal . This enables it to preserve the literal type in the resulting object. Using constraints in this way can be especially useful when you need to enforce exact values in your data structures.
Literal Inference in Arrays
A similar pattern emerges when returning values within an array. Without any constraints, TypeScript widens the literal type for the elements in the array:
function wrapInArray<T>(input: T): T[] {
return [input];
}
const arrayResult = wrapInArray(42);
// The inferred type of arrayResult is: number[]
Even though
42
is a literal number , when it’s placed inside an array, TypeScript infers the type asnumber[]
. The rationale here is that arrays are mutable collections ; their contents may change over time, so preserving the exact literal type isn’t as critical.
Conclusion
Understanding how TypeScript infers literal types in generics can significantly improve the predictability and safety of your code. When values are returned directly , TypeScript tends to preserve the literal type . However, once you wrap those values in objects or arrays, it opts for a broader type to allow for future modifications.By applying constraints to your generics, you can steer TypeScript to maintain literal types when that precision is necessary.
Keep these insights in mind as you develop more complex TypeScript applications, and experiment with constraints to fully harness the power of TypeScript’s type inference system . Happy coding! 🚀