Understanding Scope in JavaScript: A Comprehensive Guide
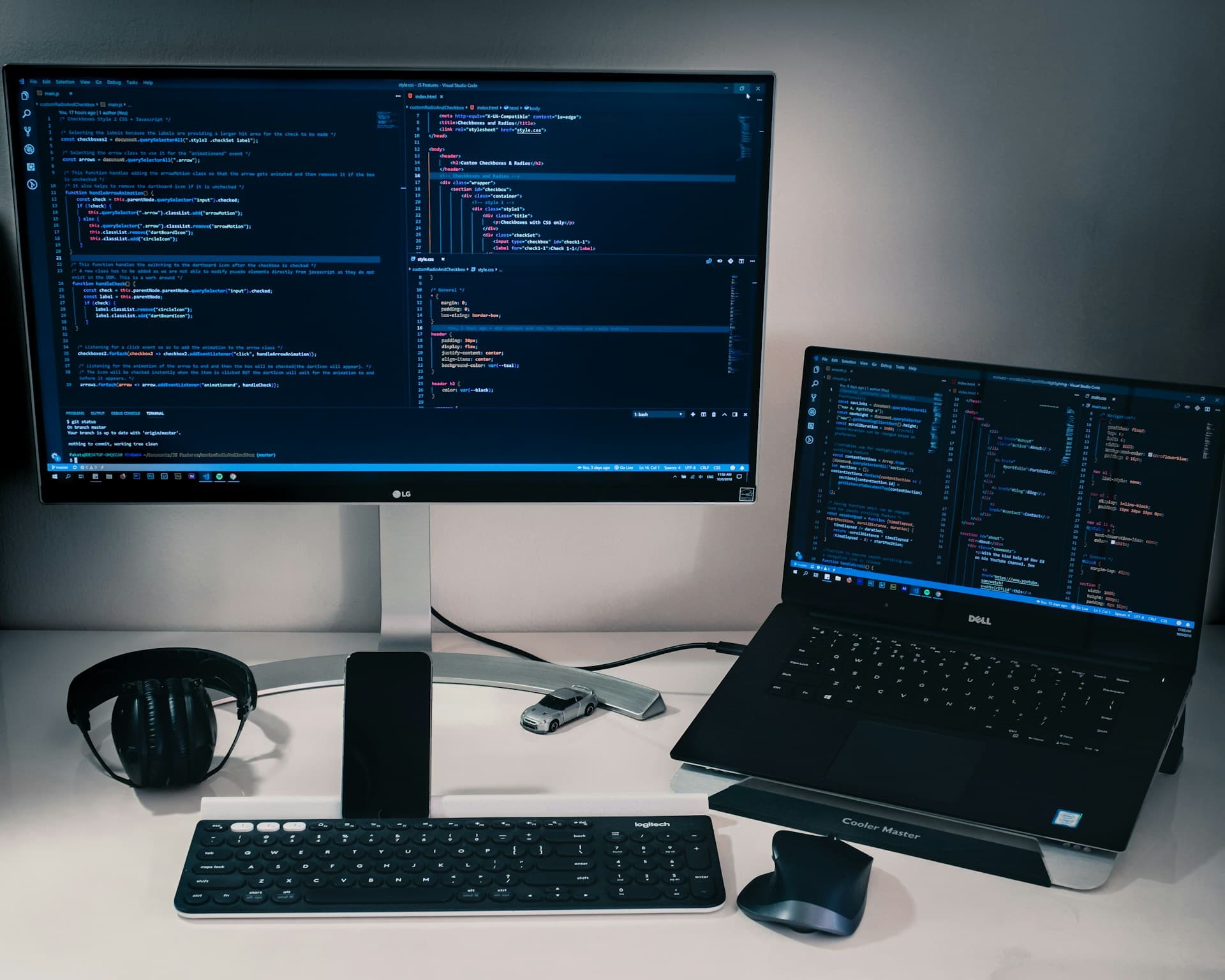
Table of Content
Scope is a fundamental concept in JavaScript that determines the visibility and accessibility of variables throughout your code. In this post, we'll delve into the intricacies of scope, exploring the different types and their impact on variable lifetime.
Global Scope
Variables declared outside of any function or block have global scope. They can be accessed from anywhere in your code, making them accessible throughout the entire program.
var globalVar = "I am a global variable";
function exampleGlobalScope() {
console.log(globalVar); // Outputs: I am a global variable
}
However, relying heavily on global variables can lead to naming conflicts and make code harder to maintain, so it's essential to use them judiciously.
Local Scope
Function Scope
Variables declared inside a function have function scope, meaning they are accessible only within that function. This helps encapsulate variables and avoid unintended interactions with other parts of the code.
function exampleFunctionScope() {
var localVar = "I am a local variable";
console.log(localVar); // Outputs: I am a local variable
}
console.log(localVar); // Error: localVar is not defined
Block Scope
Introduced with ES6, block scope allows variables to be scoped to a specific block of code, such as an if statement or a for loop.
if (true) {
let blockVar = "I am a block-scoped variable";
console.log(blockVar); // Outputs: I am a block-scoped variable
}
console.log(blockVar); // Error: blockVar is not defined
Lexical Scope
JavaScript uses lexical scoping, which means the scope of a variable is determined by its position within the code. When a function is defined, it captures the scope in which it is defined, allowing it to access variables from that scope even if it's called in a different scope.
function outerFunction() {
var outerVar = "I am an outer variable";
function innerFunction() {
console.log(outerVar); // Outputs: I am an outer variable
}
innerFunction();
}
outerFunction();
Hoisting
JavaScript hoists variable declarations to the top of their scope during the compilation phase. This means you can use a variable before it's declared, but the assignment will not be hoisted.
console.log(hoistedVar); // Outputs: undefined
var hoistedVar = "I am hoisted";
Understanding scope is crucial for writing clean and bug-free JavaScript code. By grasping the different types of scope and their implications, you'll be better equipped to design robust and maintainable applications.
Happy coding!