Understanding TypeScript's reduce with Type Arguments
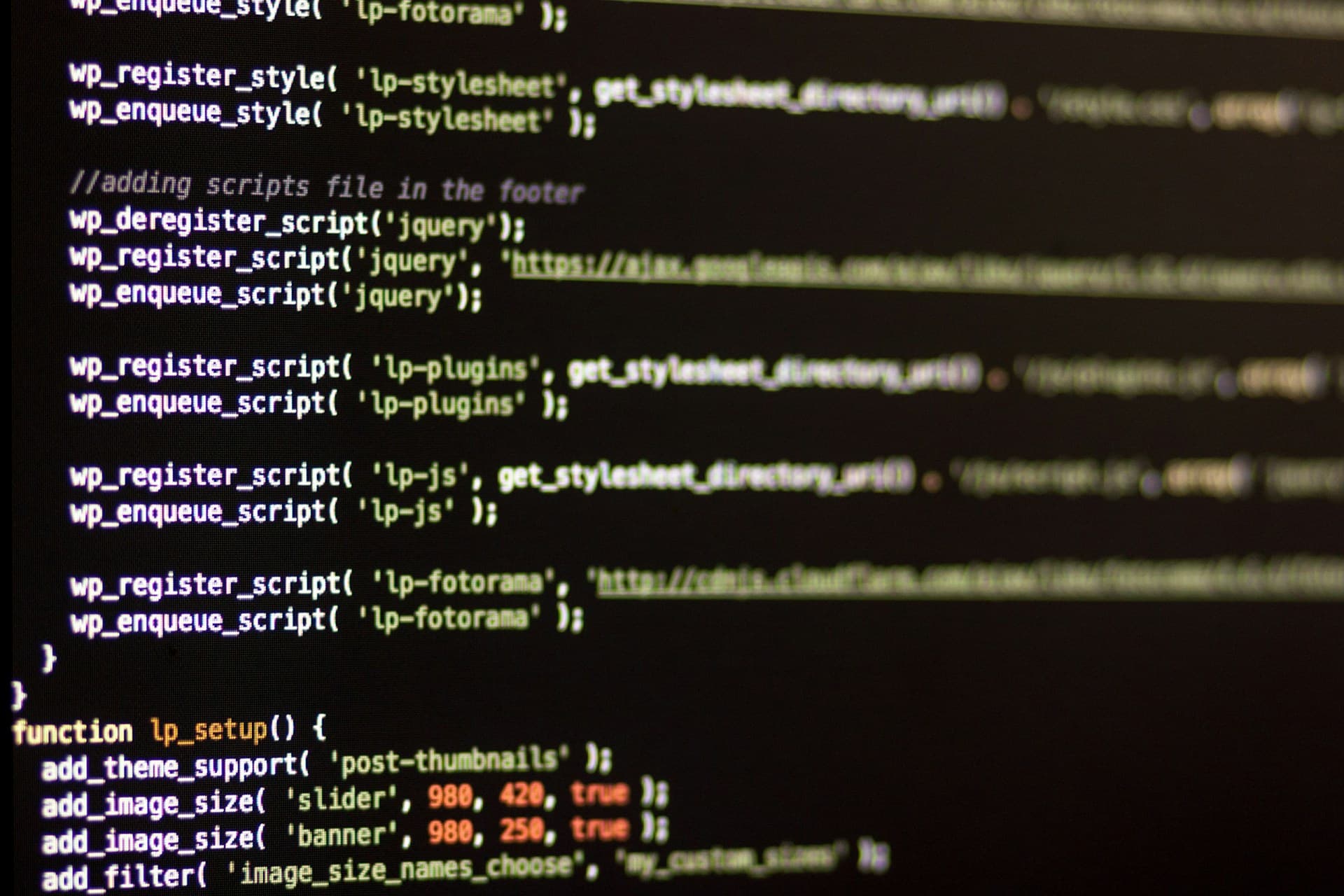
Table of Content
- The Type Signature of `reduce`
- 1. When No Initial Value is Provided
- 2. With an Initial Value Matching the Array Element Type
- 3. Transforming to a Different Type
- Inferring Types in `reduce`
- Basic Implementation
- Updating the Type Argument
- 1. Using Type Arguments
- 2. Using Type Assertions
- 3. Explicitly Typing the Accumulator Parameter
- Comparing the Approaches
- Real-World Example
- Conclusion
When working with TypeScript, understanding how to leverage type arguments in array methods like
reduce
can significantly improve type safety and code clarity. In this article, we'll explore how to pass type arguments to thereduce
function, with practical examples to showcase the concepts.
The Type Signature of reduce
The reduce
method has multiple type signatures, known as function overloads. These overloads determine the behavior of reduce
based on the parameters passed. Let’s break them down:
1. When No Initial Value is Provided
reduce(
callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: readonly T[]) => T
): T;
This version is used when the accumulator's type is the same as the array's element type (T
), and no initialValue
is passed.
2. With an Initial Value Matching the Array Element Type
reduce(
callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: readonly T[]) => T,
initialValue: T
): T;
Here, the accumulator type and the array element type are the same (T
), but an initialValue
is provided.
3. Transforming to a Different Type
reduce<U>(
callbackfn: (previousValue: U, currentValue: T, currentIndex: number, array: readonly T[]) => U,
initialValue: U
): U;
This overload allows transforming the array into a different type (U
). The type of the accumulator (U
) is inferred from the initialValue
.
Inferring Types in reduce
Consider an array of objects:
const array = [{ name: "Alice" }, { name: "Bob" }];
If we want to transform this array into a Record<string, { name: string }>
where the name serves as the key, we use reduce
like this:
Basic Implementation
const result = array.reduce((accum, item) => {
accum[item.name] = item;
return accum;
}, {});
However, this leads to a type inference issue. TypeScript initially infers the accumulator (accum
) as {}
(an empty object), which doesn't support assigning properties dynamically without type assertions or explicit typing.
Updating the Type Argument
We can explicitly define the desired accumulator type in one of the following ways:
1. Using Type Arguments
The cleanest solution is to use a type argument for reduce
:
const result = array.reduce<Record<string, { name: string }>>((accum, item) => {
accum[item.name] = item;
return accum;
}, {});
Here, we declare the accumulator type (Record<string, { name: string }>
) directly. TypeScript now understands what the accumulator should look like.
2. Using Type Assertions
Another option is to cast the initial value as the desired type:
const result = array.reduce(
(accum, item) => {
accum[item.name] = item;
return accum;
},
{} as Record<string, { name: string }>,
);
While effective, this approach can be less preferred due to the reliance on type assertions, which bypass TypeScript's inference system.
3. Explicitly Typing the Accumulator Parameter
You can also explicitly annotate the accumulator parameter within the callback:
const result = array.reduce((accum: Record<string, { name: string }>, item) => {
accum[item.name] = item;
return accum;
}, {});
This method is concise and ensures TypeScript enforces the desired accumulator type throughout the callback function.
Comparing the Approaches
Approach | Pros | Cons |
---|---|---|
Type Arguments | Clean, concise, no casting required. | Slightly less obvious for beginners. |
Type Assertions | Works well for quick solutions. | Skips type inference; less robust. |
Explicit Accumulator | Clear intent and strict type enforcement. | Slightly verbose in callback signature. |
In most cases, type arguments or an explicit accumulator type are preferred, as they maintain clarity and leverage TypeScript’s type system effectively.
Real-World Example
Imagine we have an array of user objects, and we want to create a lookup table with user IDs as keys:
const users = [
{ id: 1, name: "Alice" },
{ id: 2, name: "Bob" },
];
const userMap = users.reduce<Record<number, { id: number; name: string }>>(
(accum, user) => {
accum[user.id] = user;
return accum;
},
{},
);
console.log(userMap);
/*
{
1: { id: 1, name: 'Alice' },
2: { id: 2, name: 'Bob' }
}
*/
Here, the type argument ensures that accum
is always treated as Record<number, { id: number; name: string }>
, and TypeScript enforces this structure during compilation.
Conclusion
Using type arguments in reduce
allows you to unlock the full potential of TypeScript’s type inference, ensuring type-safe and expressive transformations. While there are multiple ways to define the accumulator's type, using type arguments or explicitly typing the accumulator are the most robust approaches. By understanding reduce
’s type signatures, you can handle complex data transformations with confidence.