Best Practices for Using Environment Variables in Node.js Applications
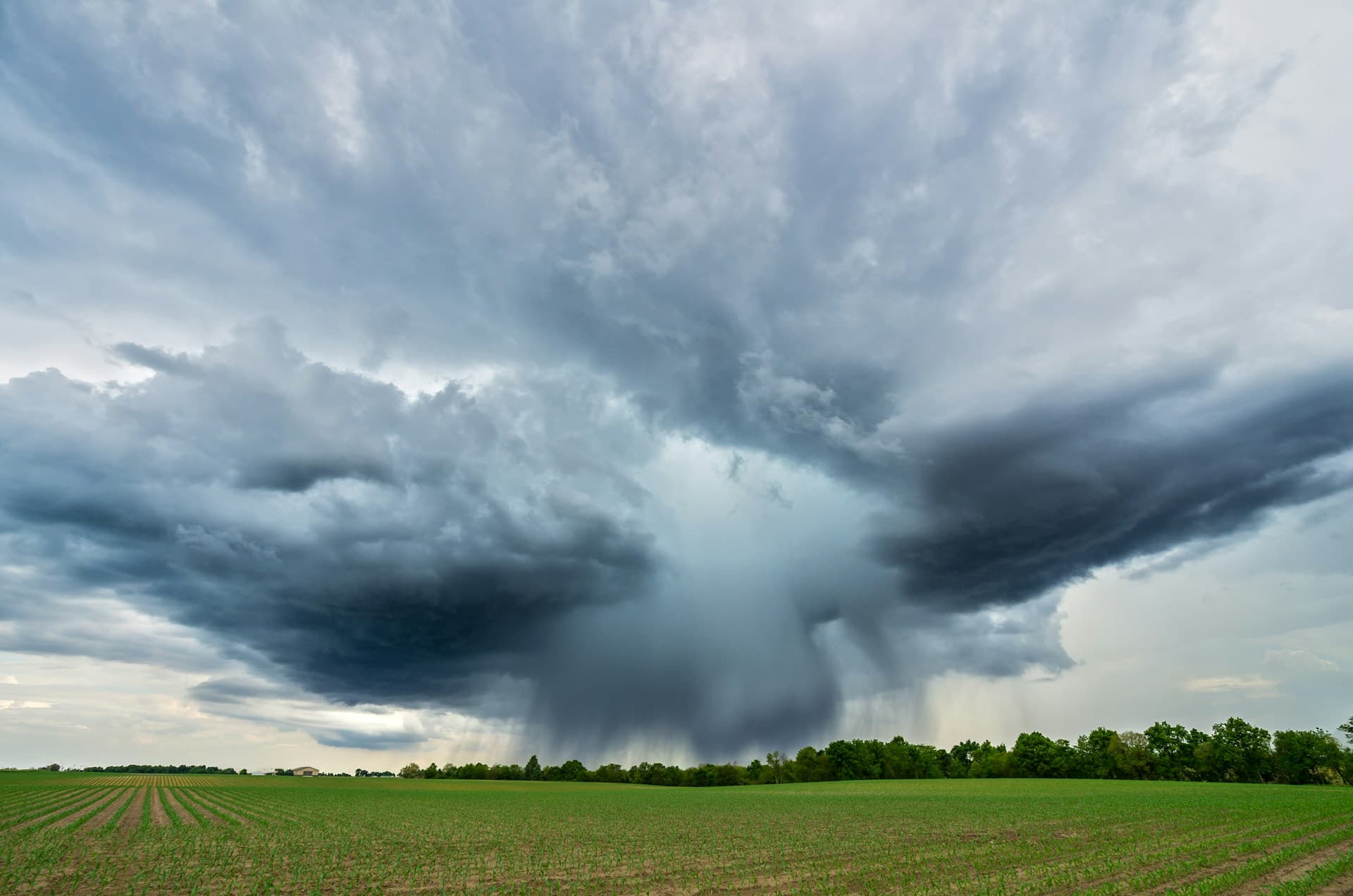
Table of Content
- Best Practices for Managing Environment Variables
- 1. Use a `.env` File
- 2. Keep Secrets Out of Your Codebase
- 3. Validate Environment Variables
- 4. Default Values and Fallbacks
- 5. Fail Fast on Missing or Invalid Variables
- 6. Avoid Exposing Secrets
- 7. Use Strong Secrets
- 8. Leverage Infrastructure for Secret Management
- Validating Environment Variables with Zod
- Step 1: Install Required Dependencies
- Step 2: Define a Schema for Validation
- Step 3: Load and Validate Environment Variables
- Error Handling Example
- Benefits of Using Zod for Validation
- Conclusion
- Key Takeaways:
Environment variables are crucial for storing configuration values that are unique to each environment (e.g., development, production, testing). They allow you to separate code from configuration, which is a cornerstone of the 12-Factor App methodology. However, improper handling of environment variables can lead to security vulnerabilities, misconfigurations, or difficult-to-debug errors.
This article covers best practices for working with environment variables and demonstrates how to validate them using the powerful Zod library in Node.js.
Why Environment Variables Matter
Environment variables enable developers to:
- Decouple configuration from code: Separate sensitive or environment-specific settings.
- Enhance security: Avoid hardcoding secrets (e.g., API keys, database credentials).
- Facilitate deployment: Easily switch configurations between environments.
- Improve flexibility: Simplify runtime customization without modifying code.
Best Practices for Managing Environment Variables
1. Use a .env
File
Store environment variables in a .env
file during local development. This file should be excluded from version control using .gitignore
to prevent sensitive information from being committed.
Example .env
file:
NODE_ENV=development
PORT=3000
CLIENT_URL=http://localhost:3000
SESSION_SECRET=supersecret
Use a library like dotenv
to load the .env
file:
require("dotenv").config();
2. Keep Secrets Out of Your Codebase
Never hardcode sensitive information like API keys, database credentials, or secrets directly in your code. Always reference these values through environment variables.
3. Validate Environment Variables
Use a schema validation library like Zod to validate environment variables at runtime. This ensures the application fails fast if required variables are missing or invalid, reducing potential issues.
4. Default Values and Fallbacks
Provide sensible defaults for non-critical environment variables to ensure your application runs in most environments.
5. Fail Fast on Missing or Invalid Variables
Your application should immediately terminate if critical environment variables are missing or invalid. This prevents running in a partially configured state.
6. Avoid Exposing Secrets
Be cautious not to expose secrets to the frontend. For example, filter variables that are injected into client-side code through tools like Webpack or Vite.
7. Use Strong Secrets
Ensure that secrets like SESSION_SECRET
and JWT_SECRET
are long and randomly generated.
8. Leverage Infrastructure for Secret Management
In production, use secret management services like AWS Secrets Manager, Azure Key Vault, or HashiCorp Vault instead of .env
files.
Validating Environment Variables with Zod
Step 1: Install Required Dependencies
Install the Zod library:
npm install zod
Step 2: Define a Schema for Validation
Create a schema for your environment variables using Zod. This ensures each variable has the correct type, is required when necessary, and provides clear error messages.
Example schema:
import { z } from "zod";
const envSchema = z.object({
NODE_ENV: z
.enum(["development", "production", "test"])
.default("development"),
ENVIRONMENT: z
.string()
.min(1, "ENVIRONMENT is required")
.default("development"),
PORT: z.string().min(1, "PORT is required"),
CLIENT_URL: z.string().url().default("http://localhost:3000"),
SESSION_SECRET: z.string().min(1, "SESSION_SECRET is required"),
JWT_SECRET: z.string().min(1, "JWT_SECRET is required"),
DATABASE_URL: z.string().url().min(1, "DATABASE_URL is required"),
});
const parsedEnv = envSchema.safeParse(process.env);
if (!parsedEnv.success) {
console.error(
"Environment variable validation failed:",
parsedEnv.error.format(),
);
process.exit(1); // Terminate the application
}
export default parsedEnv.data;
Step 3: Load and Validate Environment Variables
Ensure the .env
file is loaded before validation:
import "dotenv/config";
import parsedEnv from "./config/env"; // Example path to the above schema
console.log("Environment variables loaded successfully:", parsedEnv);
Error Handling Example
If validation fails, the application terminates with a clear error message:
Environment variable validation failed:
{
PORT: [ 'PORT is required' ],
DATABASE_URL: [ 'DATABASE_URL is required' ]
}
This ensures misconfigurations are caught early, improving developer experience and deployment reliability.
Benefits of Using Zod for Validation
- Type safety: Works seamlessly with TypeScript.
- Clear error messages: Provides detailed and actionable errors.
- Composable schemas: Easily reusable and extensible for different environments.
Conclusion
Environment variables are a critical part of modern application development. By following best practices and using tools like Zod for validation, you can ensure your application is secure, robust, and maintainable.
Key Takeaways:
- Always validate your environment variables.
- Keep sensitive information out of your codebase.
- Use secret management tools in production.
Start implementing these practices today to enhance the reliability and security of your applications!