Forcing Modules With moduleDetection in TypeScript
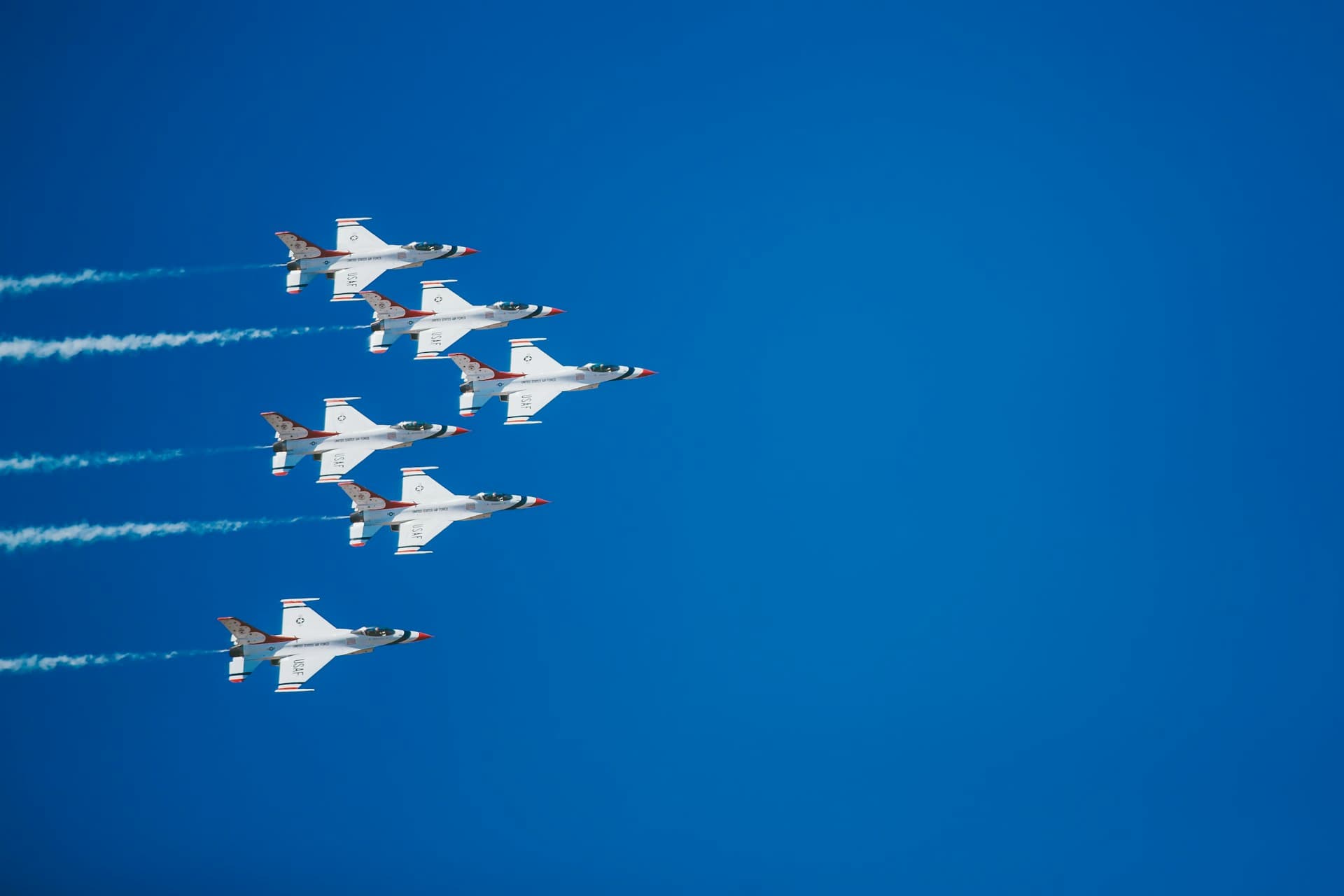
TypeScript’s
moduleDetection
setting plays a key role in determining how variables and functions are scoped across your project. This configuration ensures consistent behavior in the handling of modules, enabling better maintainability and fewer surprises. Let’s explore the three options available formoduleDetection
, with a focus on theforce
setting.
Understanding moduleDetection
Options
1. auto
(Default)
When set to auto
, TypeScript detects whether a file is a module based on the presence of import
or export
statements. Files without these statements are treated as global scripts, allowing functions and variables to be accessed without explicitly importing them.
2. force
The force
option treats all files in the project as modules, even if they lack import
or export
statements. This approach ensures that files are isolated by default, preventing unintentional global variable leakage. You must explicitly use import
and export
statements to share code between files.
3. legacy
The legacy
option is included for compatibility with older TypeScript versions. It’s rarely needed in modern projects and can generally be ignored.
Why Use the force
Option?
Switching to force
mode provides several benefits:
- Stronger Isolation: Each file is scoped as a module, reducing accidental variable overlap and global pollution.
- Real-World Alignment: Mimics how modules behave in modern JavaScript environments, preparing your code for real-world scenarios.
- Error Prevention: Ensures you explicitly declare dependencies between files, reducing unexpected runtime errors.
Updating tsconfig.json
for force
Updating your project to use force
is simple. Add the moduleDetection
setting to your tsconfig.json
file:
{
"compilerOptions": {
// ...other options...
"moduleDetection": "force"
}
}
Once this change is applied, all files in the project will be treated as modules. You’ll need to use import
and export
statements to access functions, variables, and classes across files. Here’s an example of the new structure:
Example: Code Before and After force
Without moduleDetection: force
:
// utils.ts
function greet(name: string) {
return `Hello, ${name}!`;
}
// main.ts
console.log(greet("Alice")); // Works without explicit import
With moduleDetection: force
:
// utils.ts
export function greet(name: string) {
return `Hello, ${name}!`;
}
// main.ts
import { greet } from "./utils";
console.log(greet("Alice")); // Explicit import required
Best Practices When Using force
- Organize Your Code: Use well-structured module imports and exports to ensure clear dependency relationships.
- Lint for Missing Imports: Employ tools like ESLint to detect and fix missing imports automatically.
- Refactor Gradually: If you’re updating an existing project, migrate files incrementally to avoid overwhelming changes.
Conclusion
The moduleDetection: "force"
setting is a great choice for modern TypeScript projects. It promotes modularity, reduces errors, and aligns your code with contemporary JavaScript practices. By explicitly defining dependencies between files, your codebase becomes easier to maintain and more robust.
Adopting this approach may require some initial adjustments, but the long-term benefits far outweigh the effort. Give it a try in your project today!