Go's select Statement: Managing Concurrency in Go with Meaningful Examples
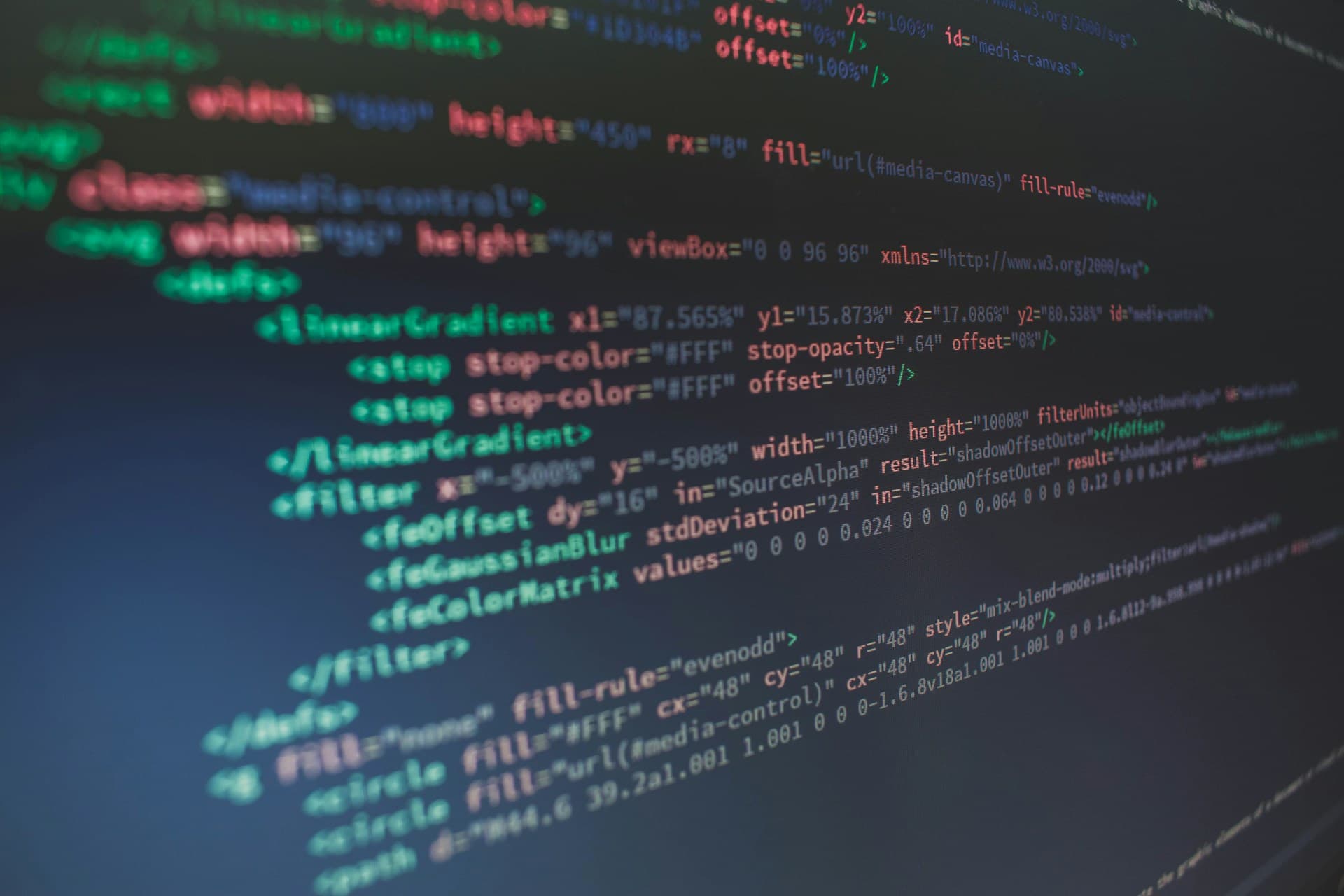
Go's select
statement is a powerful tool that helps developers handle concurrency effectively. It provides a way for Goroutines to communicate over multiple channels without favoring any one channel, which avoids common issues like starvation (where one case always gets picked, preventing others from executing) and deadlock (where Goroutines get stuck waiting on each other indefinitely). In this article, we’ll walk through the basics of select
, explore useful patterns, and provide beginner-friendly examples.
Concurrency in Go: The
select
statement in Go enables efficient management of multiple channel operations, helping developers prevent deadlocks and ensure responsiveness in concurrent applications.
What is the select Statement?
The select
statement in Go is used to wait on multiple channel operations, and it executes one of the ready cases. It’s similar to a switch statement but works with channels, enabling you to handle concurrent operations gracefully. Unlike a switch, select
does not prioritize cases; instead, it picks a ready case randomly if multiple cases are possible, preventing one case from "starving" others.
Here’s a basic example:
select {
case v := <-ch1:
fmt.Println("Received from ch1:", v)
case v := <-ch2:
fmt.Println("Received from ch2:", v)
case ch3 <- 42:
fmt.Println("Sent 42 to ch3")
default:
fmt.Println("No channels ready")
}
- Each case represents a channel operation (reading or writing).
- If multiple channels are ready, one is chosen randomly.
- The
default
case executes if no other cases are ready, making this a non-blockingselect
.
Using select to Avoid Deadlocks
Deadlock is a common concurrency issue where Goroutines end up waiting on each other indefinitely. Here’s a typical example that would lead to deadlock:
func main() {
ch1 := make(chan int)
ch2 := make(chan int)
go func() {
ch1 <- 1 // Sends 1 to ch1
val := <-ch2 // Waits to receive from ch2
fmt.Println("Goroutine received:", val)
}()
ch2 <- 2 // Sends 2 to ch2
val := <-ch1 // Waits to receive from ch1
fmt.Println("Main received:", val)
}
This code will produce a deadlock because:
- The main Goroutine waits to receive from
ch1
. - The anonymous Goroutine waits to receive from
ch2
.
Each Goroutine is waiting for the other to proceed, creating a deadlock situation. When you run this in The Go Playground, you’ll get an error message indicating that all Goroutines are asleep, meaning the program is deadlocked.
Fixing Deadlock with select
We can use select
to avoid this deadlock by allowing either channel to proceed if it’s ready:
func main() {
ch1 := make(chan int)
ch2 := make(chan int)
go func() {
ch1 <- 1
val := <-ch2
fmt.Println("Goroutine received:", val)
}()
var val int
select {
case ch2 <- 2:
case val = <-ch1:
}
fmt.Println("Main received:", val)
}
With select
, if ch2
is ready, it will receive 2; if ch1
is ready, it will receive the value from ch1
into val
. This ensures that the program does not deadlock because select
checks if any channels are ready to proceed and selects one randomly.
Example: Using select in a for Loop
A common use of select
is in a for
loop, often called a for-select loop. This combination is useful for continuous monitoring of channels until a certain condition is met.
func main() {
ch := make(chan int)
done := make(chan bool)
// Goroutine to send values to ch
go func() {
for i := 1; i <= 5; i++ {
ch <- i
}
done <- true
}()
// Loop to receive values from ch
for {
select {
case val := <-ch:
fmt.Println("Received:", val)
case <-done:
fmt.Println("Done receiving")
return
}
}
}
In this example:
- A Goroutine sends numbers to
ch
, then signals completion by sending a value todone
. - The main Goroutine uses a for-select loop to receive values from
ch
untildone
receives a value, indicating that the Goroutine has finished.
This example avoids deadlock and processes values as they become available, making it an ideal pattern for handling continuous data streams.
The Default Case in select: Non-Blocking Operations
The default
case in a select
block allows you to perform a non-blocking operation, meaning it won’t wait for channels to be ready.
select {
case val := <-ch:
fmt.Println("Read from ch:", val)
default:
fmt.Println("No value available, proceeding without blocking")
}
If ch
has no values, select
will skip the read and execute default
immediately. This is helpful if you want to check for values on a channel without waiting.
Example: Using select with Timeout
Sometimes, you want a Goroutine to wait only for a certain amount of time. This can be achieved with a timeout using select
and time.After
:
import "time"
func main() {
ch := make(chan int)
select {
case val := <-ch:
fmt.Println("Received:", val)
case <-time.After(2 * time.Second):
fmt.Println("Timed out")
}
}
In this code:
- If
ch
has no value within 2 seconds, the<-time.After
case executes, printing "Timed out." - This pattern is useful for preventing indefinite waits on channels.
Backpressure with select and default
Backpressure is a common technique to prevent overwhelming a system when resources are scarce. With select
, you can use the default
case to discard data when the channel is full.
func main() {
ch := make(chan int, 1)
for i := 1; i <= 5; i++ {
select {
case ch <- i:
fmt.Println("Sent:", i)
default:
fmt.Println("Discarded:", i)
}
}
}
Here:
- We attempt to send values 1-5 to
ch
. - If
ch
is full, thedefault
case executes, discarding the value and avoiding blocking.
Summary
The select
statement in Go is a powerful tool for managing concurrency, providing a way to handle multiple channels elegantly and avoid common pitfalls like deadlock and starvation. Here's a quick recap:
- Use
select
to wait on multiple channels and avoid deadlock. - Combine
for
loops andselect
for continuous channel monitoring. - The
default
case enables non-blocking operations. - Implement timeouts and backpressure to manage Goroutine communication effectively.
By incorporating select
into your concurrency patterns, you’ll be able to write more robust, efficient, and deadlock-free Go programs.