Understanding os.Stdout in Go with Practical Examples
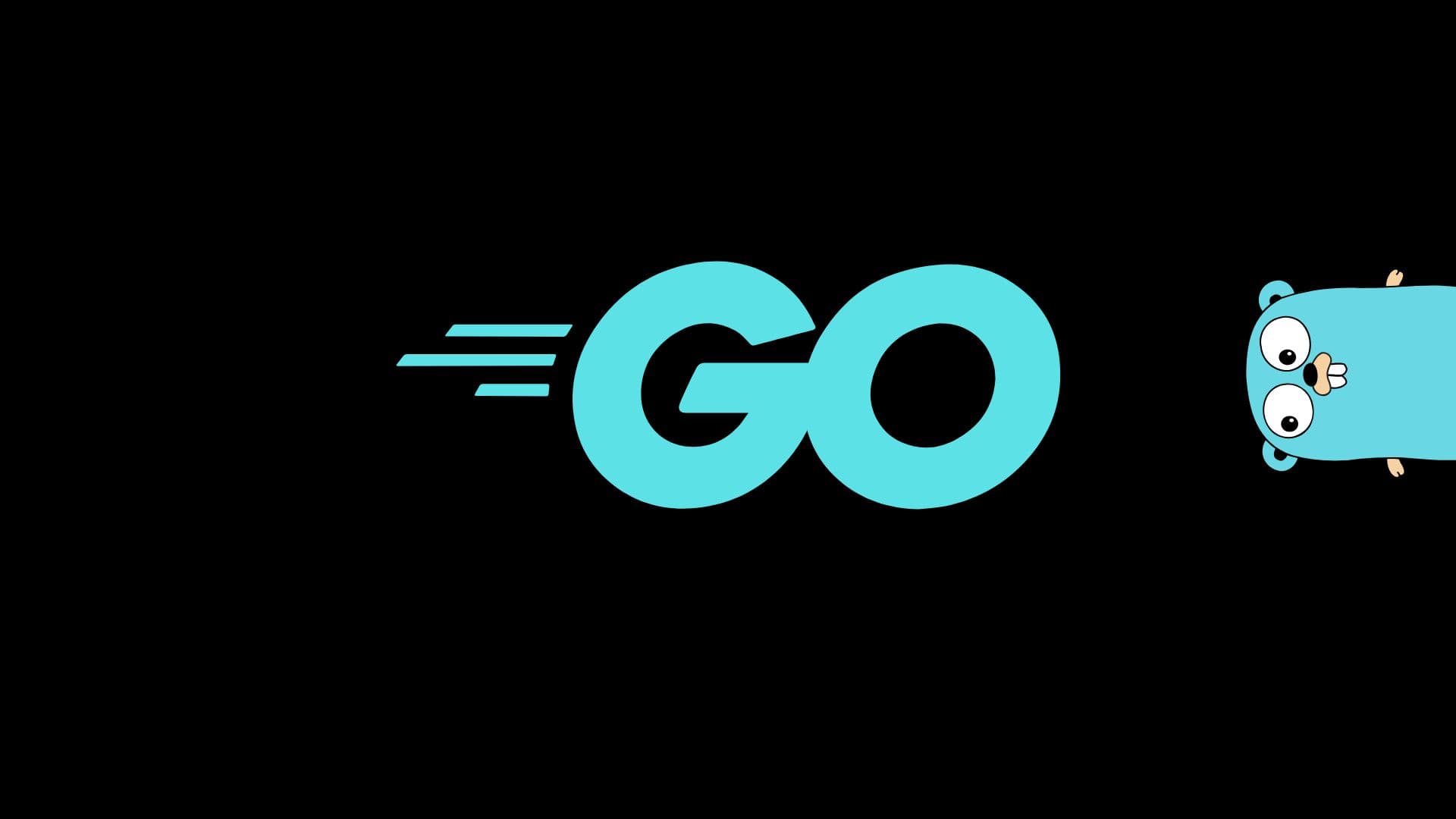
In Go, os.Stdout
is an essential part of the language’s standard library, used for interacting with the standard output stream. Whether you're writing to the terminal or redirecting output to a file, os.Stdout
provides a flexible and platform-independent way to manage how data gets outputted from your Go programs. In this post, we’ll cover the purpose of os.Stdout
, how it works, and provide examples of its usage, including how to redirect output.
Purpose of os.Stdout
os.Stdout
is a pre-declared variable of type *os.File
, which is a pointer to an os.File
object. This object represents the standard output stream of your Go program. By default, it points to the terminal where your program is being executed, but it can also be redirected to other destinations such as a file or a pipe.
In practical terms, when you write to os.Stdout
, you're sending output to the console or whatever output stream is currently being used. This is particularly useful for logging, debugging, and sending data to users through the terminal.
Example: Writing to os.Stdout
Here’s a simple example of how you can write data to os.Stdout
using both fmt
and lower-level I/O operations provided by os.File
.
package main
import (
"fmt"
"os"
)
func main() {
// Writing a string to os.Stdout using fmt
fmt.Fprintln(os.Stdout, "Hello, World!")
// Writing bytes directly to os.Stdout using os.File.Write
os.Stdout.Write([]byte("Hello again, World!
"))
}
In this example:
- We use
fmt.Fprintln()
to print a string directly toos.Stdout
. This is the higher-level approach, most commonly used for formatted output. - We use
os.Stdout.Write()
to write raw byte slices directly to the standard output. This demonstrates lower-level access to the output stream.
Key Points
- File Representation:
os.Stdout
is of type*os.File
, meaning it behaves like any file object in Go. You can use methods likeWrite()
,WriteString()
,WriteAt()
, andClose()
on it (though closingos.Stdout
is generally not recommended). - Standard Output: By default,
os.Stdout
outputs to the terminal, but it will automatically redirect output if the stream is redirected, such as when output is piped to a file. - Common Uses: Typically,
os.Stdout
is used with functions likefmt.Fprintln()
,fmt.Fprintf()
, and similar for formatted text output, but it can also be used with low-level I/O methods for greater control.
Redirecting Output
One of the powerful features of os.Stdout
is its ability to be temporarily replaced, allowing you to redirect program output to a file or any other os.File
. Here’s an example where we redirect os.Stdout
to write to a file:
package main
import (
"fmt"
"os"
)
func main() {
// Open a file for writing
file, err := os.Create("output.txt")
if err != nil {
fmt.Println("Error creating file:", err)
return
}
defer file.Close()
// Temporarily replace os.Stdout with the file
originalStdout := os.Stdout
os.Stdout = file
// This will be written to the file instead of the terminal
fmt.Println("This goes to the file!")
// Restore original os.Stdout
os.Stdout = originalStdout
fmt.Println("This goes to the terminal again!")
}
How the Redirection Works:
- We open a file using
os.Create()
, which returns an*os.File
object for the newly created file. - We save the original
os.Stdout
to a variable so that we can restore it later. - We then set
os.Stdout
to the file, meaning any output intended for the console will now go to the file instead. - After writing to the file, we restore
os.Stdout
to its original value so that the terminal can receive output again.
Summary
os.Stdout
is a platform-independent representation of the standard output stream in Go, usually tied to the terminal.- It can be written to directly or used with higher-level functions like
fmt.Println
. os.Stdout
can be redirected, allowing flexibility in where program output is sent.- Understanding how
os.Stdout
works allows you to manage output more effectively, whether to the console, files, or other streams.
Conclusion
Understanding os.Stdout
and its capabilities is critical for Go developers who want to control where their program output goes. By utilizing this built-in functionality, you can handle terminal output, file writing, and redirection with ease.
LinkedIn Post:
🌟 Understanding os.Stdout
in Go 🌟
Curious about how Go handles standard output? In this article, we dive deep into the power of os.Stdout
—a key part of Go’s I/O operations. Learn how to use it to manage terminal output, write to files, and even redirect streams with ease.
Check it out! 👇
#GoLang #Coding #SoftwareDevelopment #Stdout #Programming
[Read the full article here]