Understanding JavaScript Modules: An In-Depth Overview
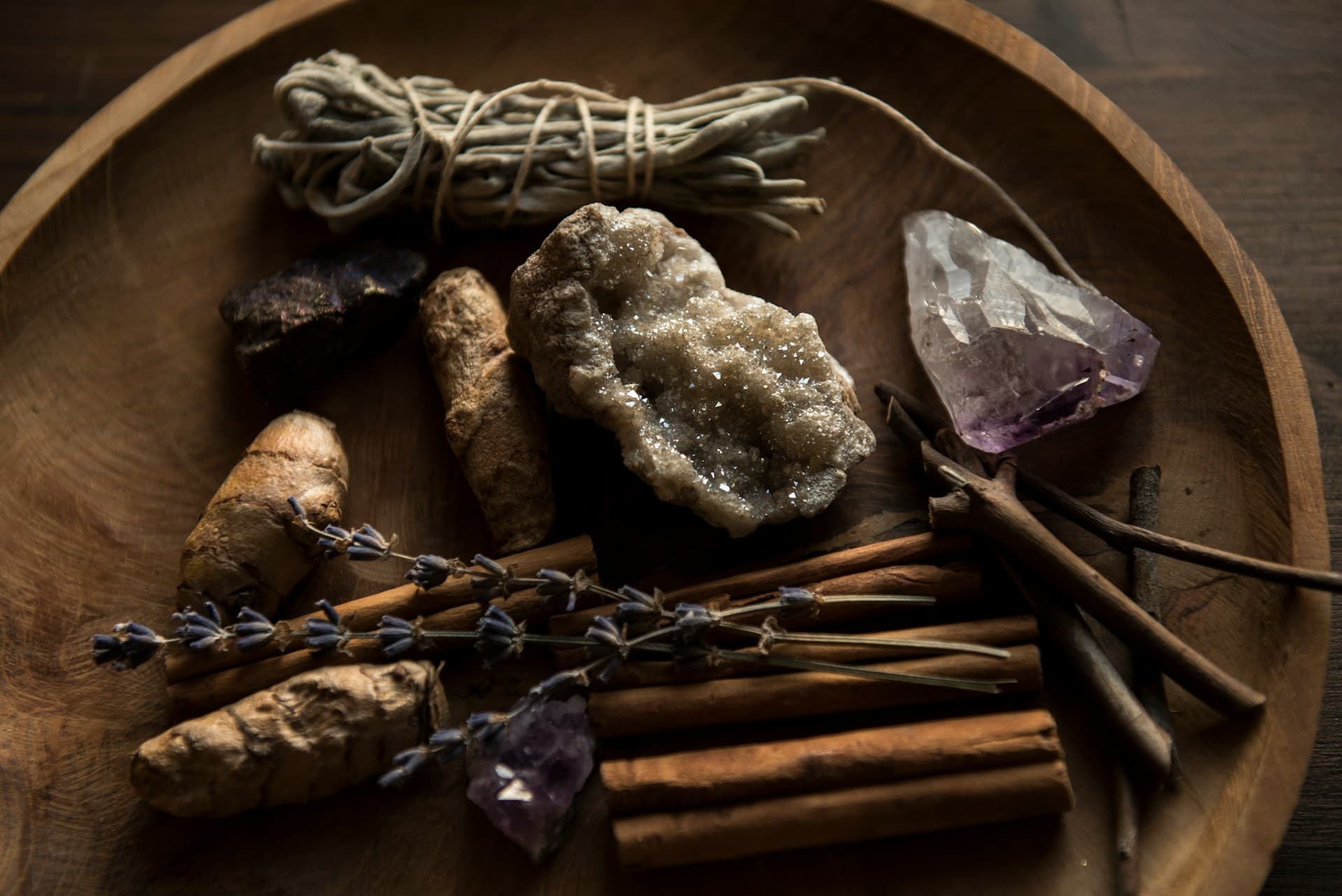
In JavaScript, modules are structures that allow you to organize code into reusable, self-contained blocks. They help manage code dependencies, encapsulate functionality, and avoid polluting the global namespace. JavaScript supports several types of modules, which have evolved over time to support different environments (like browsers and Node.js) and accommodate modern development needs. Here's an overview of the main types of modules in JavaScript:
1. CommonJS (CJS) Modules
- Environment: Node.js
- File Extension:
.js
CommonJS is the module system used by Node.js. It defines a way to load and export modules using require
and module.exports
. CommonJS modules are synchronous, meaning they load modules one at a time as the code runs, which is suitable for server-side environments like Node.js but less ideal for browsers.
Example:
// myModule.js
const greeting = "Hello, World!";
function greet() {
console.log(greeting);
}
module.exports = greet;
// main.js
const greet = require("./myModule");
greet(); // Outputs: Hello, World!
-
Usage:
require('./myModule')
imports the module.module.exports
orexports
exports functions, objects, or variables.
-
Pros:
- Simple and widely used in Node.js.
- Synchronous loading is effective for server-side applications.
-
Cons:
- Not natively supported in browsers without bundlers like Webpack.
- Synchronous loading is inefficient for large applications in client-side environments.
2. ECMAScript Modules (ESM)
- Environment: Modern Browsers and Node.js (from version 12+)
- File Extension:
.mjs
(optional in Node.js),.js
(in browsers and Node.js)
ES Modules (ESM) are part of the ES6 (ECMAScript 2015) standard and provide a more modern and standardized way of working with modules. They use the import
and export
syntax, and modules are loaded asynchronously, making them suitable for both client-side and server-side JavaScript.
Example:
// myModule.js
export const greeting = "Hello, World!";
export function greet() {
console.log(greeting);
}
// main.js
import { greet } from "./myModule.js";
greet(); // Outputs: Hello, World!
-
Usage:
import
keyword to import functions, objects, or variables.export
orexport default
to export elements from a module.
-
Pros:
- Native support in modern browsers and recent versions of Node.js.
- Asynchronous loading improves performance, especially in browsers.
- Supports tree-shaking (removal of unused code) in bundlers.
-
Cons:
- Requires
.mjs
extension or"type": "module"
inpackage.json
for Node.js to recognize the file as an ES module. - Older browsers require transpilers like Babel for compatibility.
- Requires
3. UMD (Universal Module Definition) Modules
- Environment: Browsers and Node.js
- File Extension:
.js
UMD modules are designed to work in multiple environments, including both Node.js and browsers. UMD tries to be compatible with AMD (Asynchronous Module Definition) and CommonJS, making it a versatile option for distributing libraries that need to work in different environments.
Example:
(function (root, factory) {
if (typeof module === "object" && typeof module.exports === "object") {
// Node.js/CommonJS
module.exports = factory();
} else if (typeof define === "function" && define.amd) {
// AMD (Asynchronous Module Definition)
define(factory);
} else {
// Browser global variable
root.myModule = factory();
}
})(typeof self !== "undefined" ? self : this, function () {
return {
greet: function () {
console.log("Hello, World!");
},
};
});
-
Pros:
- Works in both Node.js and browser environments.
- Useful for distributing libraries that need cross-platform support.
-
Cons:
- Complex and verbose syntax.
- Not as commonly used in modern development due to the rise of ES modules.
4. AMD (Asynchronous Module Definition) Modules
- Environment: Browsers
- File Extension:
.js
AMD modules are primarily designed for the browser and are often used with module loaders like RequireJS. They are loaded asynchronously, which is suitable for browser environments where blocking the page to load modules would be inefficient.
Example:
// myModule.js
define(["dependency1", "dependency2"], function (dep1, dep2) {
const greeting = "Hello, World!";
return {
greet: function () {
console.log(greeting);
},
};
});
// main.js
require(["myModule"], function (myModule) {
myModule.greet(); // Outputs: Hello, World!
});
-
Pros:
- Asynchronous loading is efficient for browsers.
- Modularizes code effectively for client-side JavaScript.
-
Cons:
- Requires a module loader (e.g., RequireJS).
- Less popular in modern development with the rise of ES modules and CommonJS in bundlers.
5. IIFE (Immediately Invoked Function Expression) Modules
- Environment: Browsers
- File Extension:
.js
Before the introduction of formal module systems like CommonJS or ES modules, developers used IIFEs to create modules. An IIFE is a function that runs immediately after it is defined, creating a private scope to prevent polluting the global namespace.
Example:
const myModule = (function () {
const greeting = "Hello, World!";
function greet() {
console.log(greeting);
}
return {
greet: greet,
};
})();
myModule.greet(); // Outputs: Hello, World!
-
Pros:
- Simple and doesn't require any special tooling.
- Still useful for small, self-contained scripts.
-
Cons:
- Does not handle dependencies well.
- Not scalable for larger applications.
6. ESM with Dynamic Import (import()
)
- Environment: Modern Browsers and Node.js
- File Extension:
.js
,.mjs
ES Modules support dynamic imports using the import()
function, which allows you to load modules on demand. This is useful for code-splitting and lazy loading, improving performance by loading code only when it’s needed.
Example:
async function loadModule() {
const { greet } = await import("./myModule.js");
greet();
}
loadModule();
-
Pros:
- Supports dynamic and conditional loading.
- Improves performance by loading code only when needed.
-
Cons:
- Requires an environment that supports ES modules (modern browsers or recent versions of Node.js).
Summary of Module Types
Type | Syntax | Environment | Example Usage |
---|---|---|---|
CommonJS | require , module.exports | Node.js | Server-side applications |
ES Modules | import , export | Modern Browsers, Node.js | Client-side and server-side apps |
UMD | Universal syntax | Browsers, Node.js | Cross-platform libraries |
AMD | define , require | Browsers | Browser scripts with RequireJS |
IIFE | Self-contained function | Browsers | Simple browser modules |
Conclusion
The evolution of JavaScript modules reflects the need for modular, scalable code that works across various environments. While CommonJS is still dominant in Node.js and ES Modules are the modern standard, other types like AMD and UMD provide backward compatibility and flexibility for specific use cases. Understanding these types helps you choose the right approach for your application and ensures compatibility and efficiency across platforms.