Mastering JavaScript: A Deep Dive into the New groupBy Method
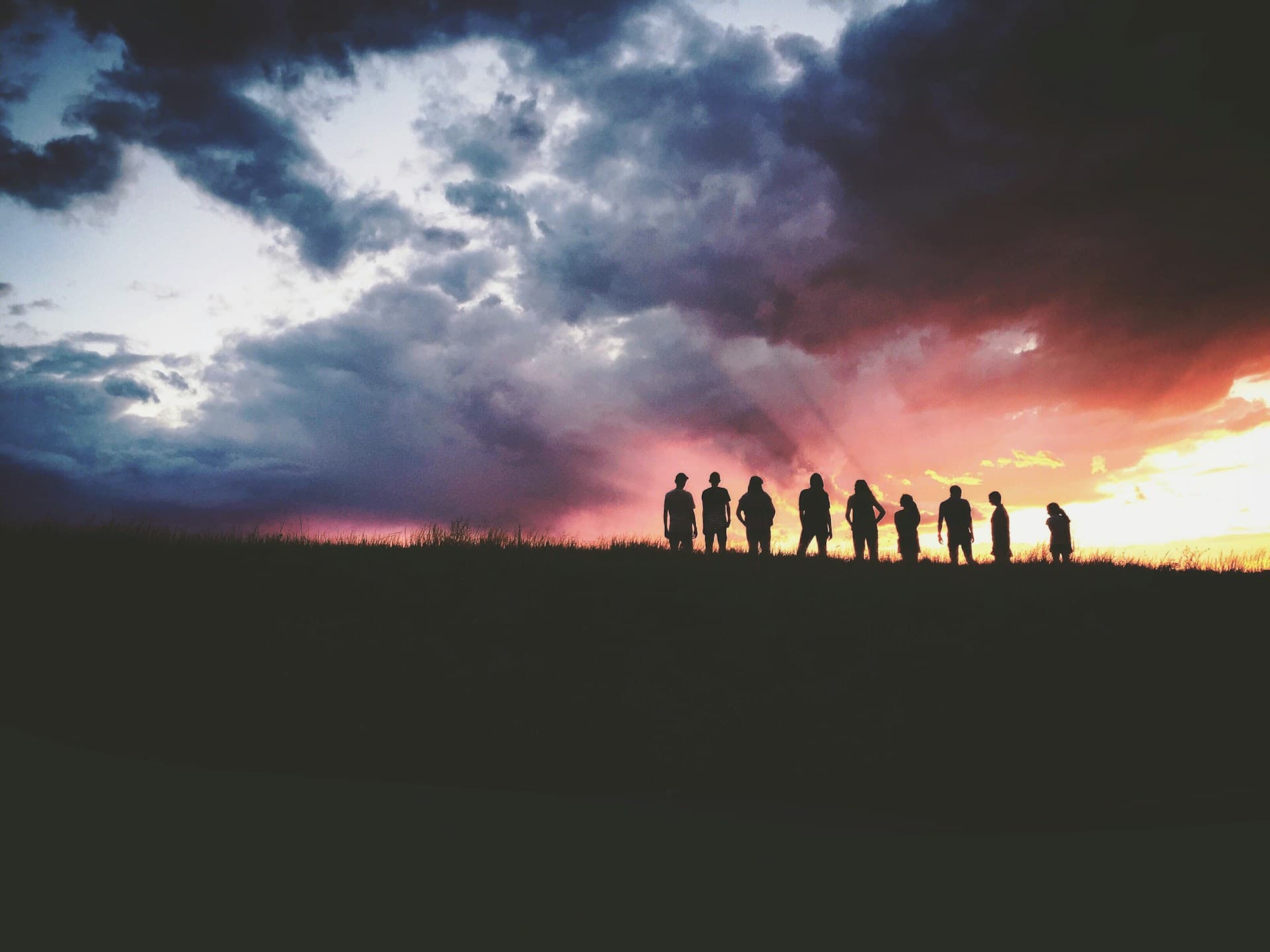
Table of Content
- What is groupBy?
- Syntax
- Why is groupBy Important?
- Before groupBy: Using reduce
- With groupBy
- Practical Examples
- 1. Grouping Objects by a Property
- 2. Grouping Numbers by Even or Odd
- 3. Grouping Strings by Length
- Handling Edge Cases
- 1. Empty Arrays
- 2. Duplicate Keys
- Browser and Runtime Support
- Polyfill Example
- Conclusion
- Ready to Embrace the Future?
JavaScript continues to evolve, and the introduction of the groupBy
method in ECMAScript 2024 (ES2024) is another step toward making the language more expressive and developer-friendly. Whether you’re managing complex datasets or simply categorizing elements in an array, groupBy
simplifies the process significantly.
In this article, we’ll explore what the
groupBy
method is, how it works, and practical use cases where it shines.
What is groupBy?
The groupBy
method is a new addition to Array.prototype
. It allows you to group the elements of an array into categories based on a grouping function you define. It returns an object where each key corresponds to a group, and the values are arrays containing the elements that belong to each group.
This new method eliminates much of the boilerplate code previously required to achieve similar results using reduce
.
Syntax
Array.prototype.groupBy(callbackFn, thisArg);
callbackFn
: A function executed on each array element. It determines the group key for each element.thisArg
(optional): A value to use asthis
when executingcallbackFn
.
The return value is an object where:
- Keys: The group identifiers generated by
callbackFn
. - Values: Arrays containing elements that belong to each group.
Why is groupBy Important?
Previously, grouping array elements in JavaScript required verbose code using reduce
or manual iteration. While these approaches work, they can be error-prone and less readable. With groupBy
, you now have a concise, declarative way to group elements in an array.
Before groupBy: Using reduce
const people = [
{ name: "Alice", age: 25 },
{ name: "Bob", age: 30 },
{ name: "Charlie", age: 25 },
{ name: "David", age: 30 },
];
const grouped = people.reduce((acc, person) => {
const key = person.age;
if (!acc[key]) {
acc[key] = [];
}
acc[key].push(person);
return acc;
}, {});
console.log(grouped);
With groupBy
const grouped = people.groupBy((person) => person.age);
console.log(grouped);
This not only reduces the code length but also makes the intention clearer.
Practical Examples
1. Grouping Objects by a Property
Imagine you have a list of people and want to group them by age:
const people = [
{ name: "Alice", age: 25 },
{ name: "Bob", age: 30 },
{ name: "Charlie", age: 25 },
{ name: "David", age: 30 },
];
const groupedByAge = people.groupBy((person) => person.age);
console.log(groupedByAge);
/*
{
25: [
{ name: 'Alice', age: 25 },
{ name: 'Charlie', age: 25 }
],
30: [
{ name: 'Bob', age: 30 },
{ name: 'David', age: 30 }
]
}
*/
2. Grouping Numbers by Even or Odd
groupBy
is equally useful for simpler data like numbers. Here’s an example of categorizing numbers as even or odd:
const numbers = [1, 2, 3, 4, 5, 6];
const groupedByParity = numbers.groupBy((num) =>
num % 2 === 0 ? "even" : "odd",
);
console.log(groupedByParity);
/*
{
odd: [1, 3, 5],
even: [2, 4, 6]
}
*/
3. Grouping Strings by Length
You can group strings based on their lengths using groupBy
:
const words = ["apple", "banana", "pear", "peach", "grape"];
const groupedByLength = words.groupBy((word) => word.length);
console.log(groupedByLength);
/*
{
5: ['apple', 'pear', 'peach', 'grape'],
6: ['banana']
}
*/
Handling Edge Cases
1. Empty Arrays
If the input array is empty, groupBy
simply returns an empty object:
const emptyArray = [];
const grouped = emptyArray.groupBy(() => "key");
console.log(grouped); // {}
2. Duplicate Keys
If multiple elements produce the same key, they are grouped into the same array.
Browser and Runtime Support
Since groupBy
is part of ES2024, it may not yet be available in all environments. You can check compatibility for your specific use case or use a polyfill for environments that don’t support it.
Polyfill Example
Here’s a simple polyfill for groupBy
:
if (!Array.prototype.groupBy) {
Array.prototype.groupBy = function (callbackFn, thisArg) {
return this.reduce((acc, item, index) => {
const key = callbackFn.call(thisArg, item, index, this);
acc[key] = acc[key] || [];
acc[key].push(item);
return acc;
}, {});
};
}
Conclusion
The introduction of groupBy
in JavaScript is a significant step forward, making it easier than ever to group and organize data in a declarative and intuitive way. Whether you’re working with arrays of objects, numbers, or strings, groupBy
simplifies previously verbose code and enhances readability.
As JavaScript continues to evolve, features like
groupBy
demonstrate the language’s commitment to improving developer productivity and code clarity. If you’re working in a modern environment or can use a polyfill, it’s time to addgroupBy
to your toolkit!
Ready to Embrace the Future?
Try incorporating groupBy
in your next project and see how it transforms the way you work with arrays. Let us know in the comments how you’ve used this new feature!