Mastering React 19’s use() Hook: Simplified Asynchronous Data Handling and Flexible Context Consumption
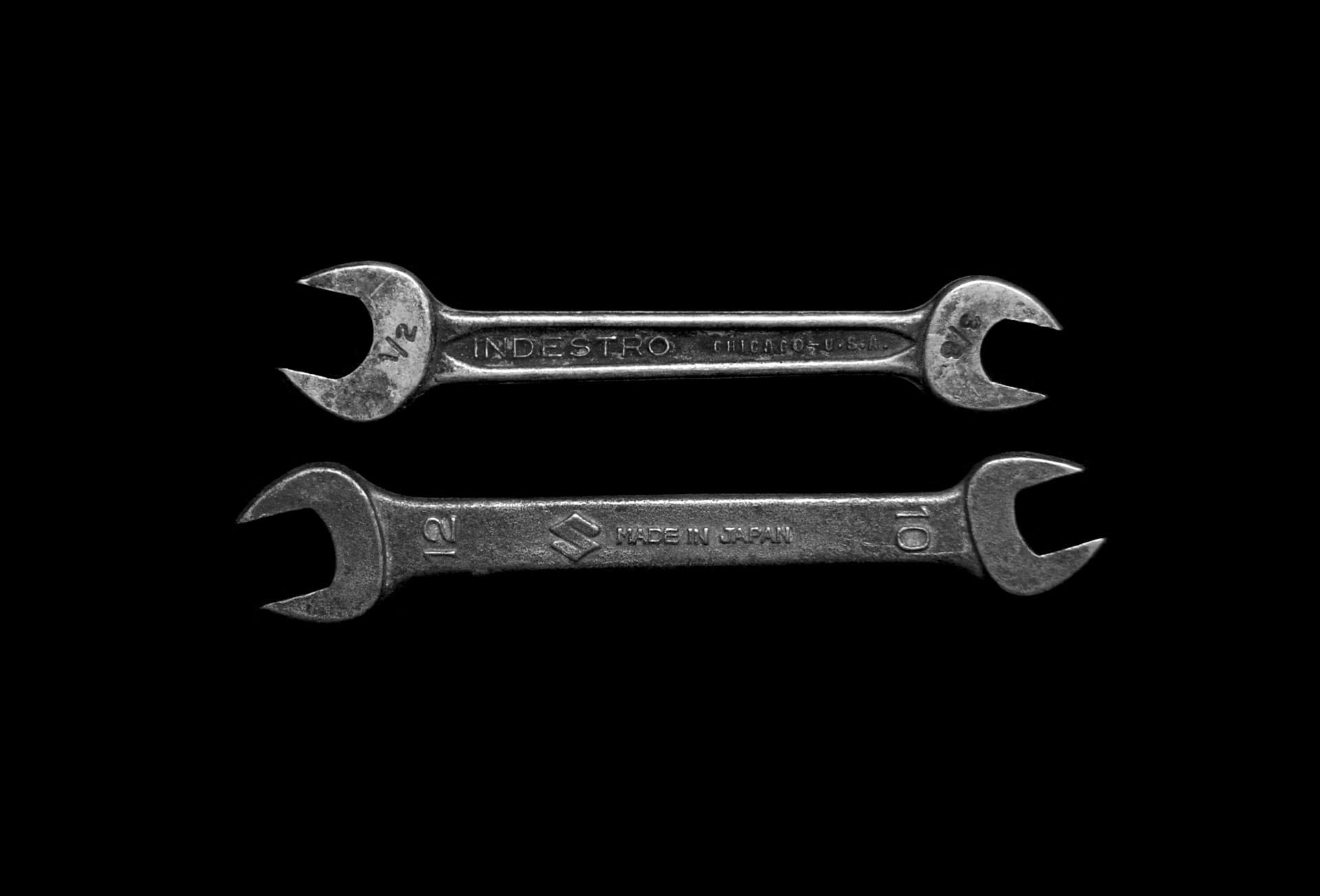
React 19 introduces the
use()
function, a versatile addition to the React API that simplifies asynchronous data handling and context consumption within components. Unlike traditional hooks,use()
can be invoked conditionally, offering greater flexibility in component logic.
Key Features of use()
-
Asynchronous Data Handling:
use()
allows components to read the value of aPromise
directly during rendering. When used with React'sSuspense
, it pauses the component's rendering until thePromise
resolves, streamlining data fetching processes. -
Context Consumption: Similar to
useContext
,use()
can access context values. However, it permits conditional usage, enabling context to be read within conditional statements or loops. -
Conditional Invocation: Unlike standard hooks,
use()
can be called inside conditional statements or loops, providing enhanced flexibility in component logic.
Using use()
with Promises
To fetch data asynchronously within a component using use()
, follow these steps:
Create an Asynchronous Function
Define a function that returns a Promise
, such as fetching data from an API.
async function fetchData() {
const response = await fetch("https://api.example.com/data");
if (!response.ok) {
throw new Error("Failed to fetch data");
}
return response.json();
}
Implement the Component
Within your component, call use()
with the Promise
returned by your asynchronous function.
import React, { use, Suspense } from "react";
function DataFetchingComponent() {
const data = use(fetchData());
return (
<div>
<h1>Data:</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
Handle Loading States with Suspense
Wrap your component with Suspense
to manage the loading state while the Promise
resolves.
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<DataFetchingComponent />
</Suspense>
);
}
In this setup,
use()
suspends the rendering ofDataFetchingComponent
untilfetchData()
resolves. During this period, the fallback content ("Loading..."
) specified inSuspense
is displayed.
Using use()
with Context
To consume context values conditionally within a component using use()
:
Create a Context
Define a context using createContext
.
import React, { createContext } from "react";
const ThemeContext = createContext("light");
Provide the Context Value
Wrap your component tree with the context provider.
function App() {
return (
<ThemeContext.Provider value="dark">
<ThemedComponent />
</ThemeContext.Provider>
);
}
Consume the Context Conditionally
Within your component, use use()
to access the context value conditionally.
import React from "react";
import { use } from "react";
function ThemedComponent({ showTheme }) {
if (!showTheme) {
return null;
}
const theme = use(ThemeContext);
return <div className={`theme-${theme}`}>Current theme: {theme}</div>;
}
In this example,
ThemedComponent
conditionally reads theThemeContext
value only ifshowTheme
is true, demonstrating the flexibility ofuse()
in conditional scenarios.
Important Considerations
-
Component Suspension: When
use()
is called with aPromise
, it suspends the component's rendering until thePromise
resolves. Ensure that the component is wrapped with aSuspense
boundary to handle this suspension appropriately. -
Error Handling: If the
Promise
passed touse()
rejects, it will trigger an error boundary. Implement error boundaries in your component tree to manage potential errors gracefully. -
Conditional Usage: The ability to call
use()
conditionally provides flexibility but requires careful management to maintain predictable component behavior.
The use()
function in React 19 enhances the developer experience by simplifying asynchronous data handling and context consumption within components. Its flexibility in conditional usage marks a significant advancement in React's API, promoting cleaner and more intuitive component logic.