Mastering TypeScript: A Deep Dive into Type Guard Functions
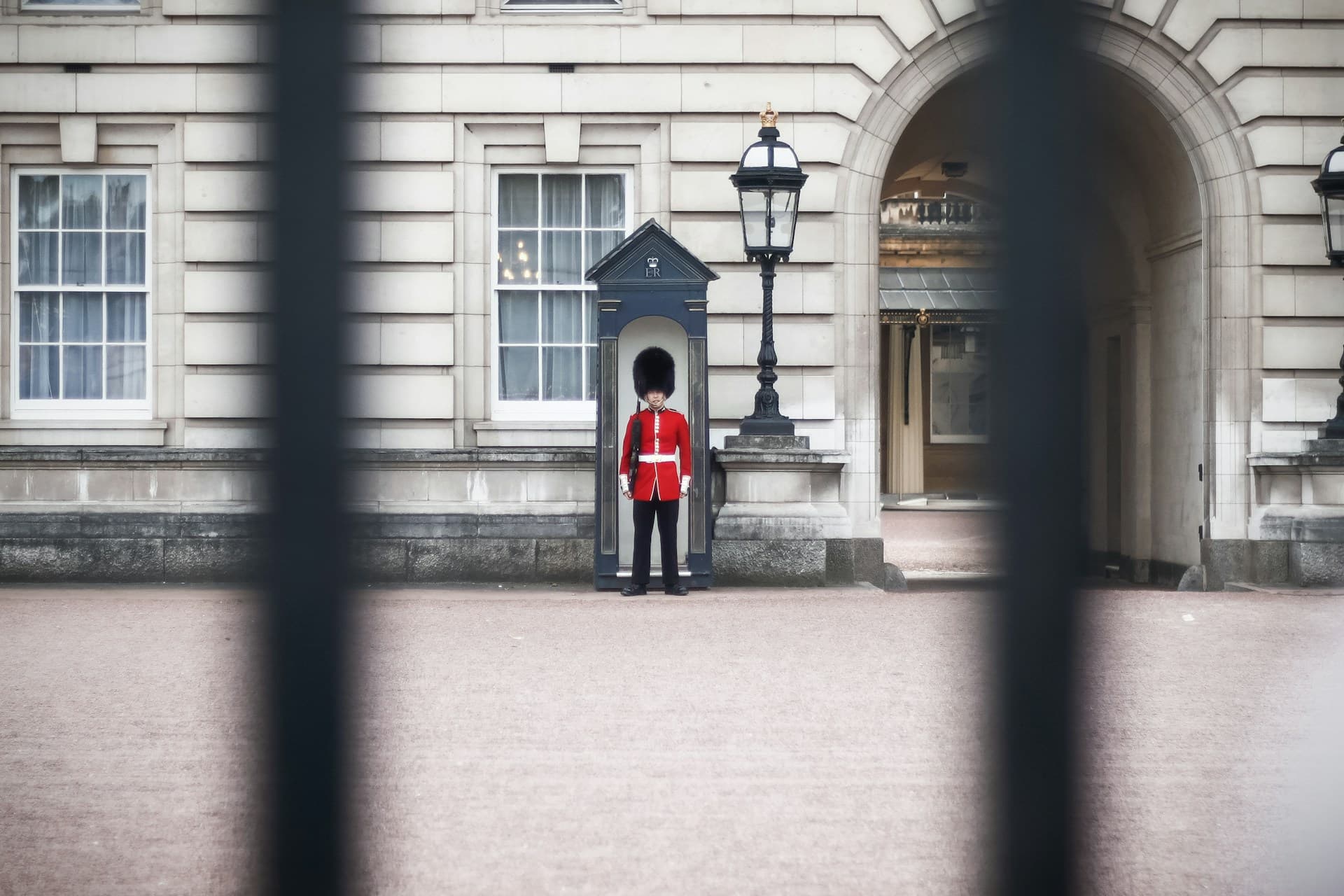
Table of Content
- What Are Type Guard Functions?
- Key Concepts
- Why Use Type Guards?
- Example Scenario:
- Built-in Type Guards
- `typeof`
- `instanceof`
- `in`
- Custom Type Guard Functions
- Syntax
- Examples
- 1. Checking for a String
- 2. Validating Array Types
- 3. Handling Interfaces
- Using Type Guards in Real Projects
- Example: Dynamic Function Behavior
- Example: Complex Data Validation
- Best Practices for Type Guards
- Common Pitfalls
- Conclusion
TypeScript is a powerful tool for building robust applications by introducing static typing to JavaScript. Among its many features, type guards are essential for handling dynamic data while maintaining type safety. This article explores type guard functions, their use cases, and best practices.
What Are Type Guard Functions?
A type guard function is a function that refines the type of a value at runtime, allowing TypeScript to narrow down its type within a conditional block. This makes it easier to work with complex or union types and ensures safer code execution.
Key Concepts
- Type Refinement: Type guards inform TypeScript that a specific variable has a particular type after passing a condition.
- Type Predicate: A type guard function returns a special type annotation in the form
value is Type
.
Why Use Type Guards?
Type guards are particularly useful when:
- Working with union types or data of uncertain types.
- Handling data from external APIs or user input.
- Ensuring runtime safety while leveraging TypeScript's compile-time type checking.
Example Scenario:
type User = { id: number; name: string };
type Admin = { id: number; name: string; admin: true };
function getUserRole(user: User | Admin) {
if ("admin" in user) {
console.log("Admin role detected");
} else {
console.log("Regular user");
}
}
Here, the type guard ('admin' in user
) determines if the object is of type Admin
or User
.
Built-in Type Guards
TypeScript offers several built-in operators that act as type guards:
typeof
function isString(value: unknown): boolean {
return typeof value === "string";
}
instanceof
function isDate(value: unknown): value is Date {
return value instanceof Date;
}
in
function hasAdminProperty(value: object): value is { admin: boolean } {
return "admin" in value;
}
Custom Type Guard Functions
Custom type guards refine types based on specific logic. They follow this general pattern:
Syntax
function isTypeName(value: unknown): value is TypeName {
return /* boolean expression */;
}
Examples
1. Checking for a String
function isString(value: unknown): value is string {
return typeof value === "string";
}
2. Validating Array Types
function isNumberArray(value: unknown): value is number[] {
return (
Array.isArray(value) && value.every((item) => typeof item === "number")
);
}
3. Handling Interfaces
interface User {
id: number;
name: string;
}
function isUser(value: unknown): value is User {
return (
typeof value === "object" &&
value !== null &&
"id" in value &&
"name" in value
);
}
Using Type Guards in Real Projects
Example: Dynamic Function Behavior
function processInput(input: string | number | Date): void {
if (isString(input)) {
console.log(`String length: ${input.length}`);
} else if (typeof input === "number") {
console.log(`Number squared: ${input * input}`);
} else if (isDate(input)) {
console.log(`Date: ${input.toISOString()}`);
}
}
Example: Complex Data Validation
type Shape =
| { kind: "circle"; radius: number }
| { kind: "rectangle"; width: number; height: number };
function isCircle(shape: Shape): shape is { kind: "circle"; radius: number } {
return shape.kind === "circle";
}
function calculateArea(shape: Shape): number {
if (isCircle(shape)) {
return Math.PI * shape.radius ** 2;
} else {
return shape.width * shape.height;
}
}
Best Practices for Type Guards
- Keep It Simple: Focus on a single responsibility for each type guard.
- Reusability: Write type guards for reusable components or complex validation logic.
- Error Handling: Ensure runtime safety by accounting for edge cases.
- Documentation: Clearly define the purpose and expected input/output for each type guard.
Common Pitfalls
- Overusing
any
orunknown
: Only use custom type guards when types are genuinely ambiguous. - Complex Logic: Avoid overly complex type guards that are hard to understand and maintain.
- Omitting Null/Undefined Checks: Always account for
null
orundefined
when dealing with objects.
Conclusion
Type guard functions are a cornerstone of TypeScript's type safety features, bridging the gap between static and dynamic typing. By using them effectively, you can write robust, maintainable, and type-safe applications. Whether you're validating API responses, refining union types, or enhancing runtime safety, mastering type guards will significantly enhance your TypeScript skills.
Happy coding! 🎉