Understanding Prefix and Postfix Operators in JavaScript
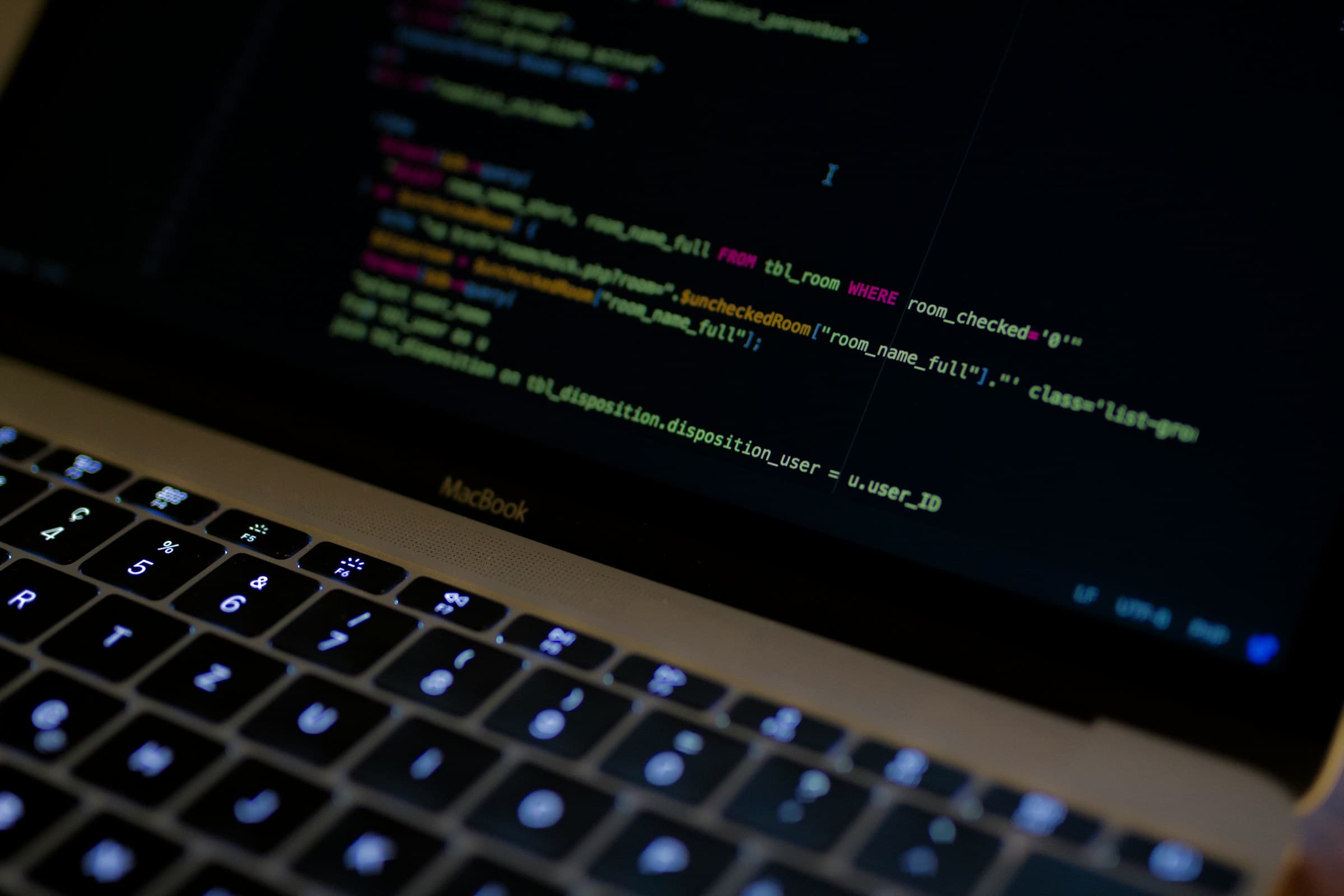
Table of Content
- 1. Prefix and Postfix Operators: An Overview
- 2. How the Prefix and Postfix Forms Work
- Increment Operator (++)
- Decrement Operator (--)
- 3. Examples to Illustrate Prefix and Postfix Behavior
- Example 1: Basic Usage of ++ and --
- Example 2: Using Prefix and Postfix in an Expression
- 4. Prefix and Postfix in Loops
- Example 3: Prefix and Postfix in a for Loop
- 5. Common Mistakes and How to Avoid Them
- 6. Best Practices for Using Prefix and Postfix Operators
- Summary
JavaScript is known for its flexibility and variety of operators, and among them are the prefix and postfix forms of the increment (++) and decrement (--) operators. These operators modify the value of a variable by adding or subtracting one and can be used in two different ways: prefix and postfix. While they might look similar, their effects are slightly different and can impact how expressions are evaluated.
This article covers everything you need to know about prefix and postfix operators in JavaScript, how they work, and examples to illustrate their behavior.
1. Prefix and Postfix Operators: An Overview
In JavaScript, both the increment (++) and decrement (--) operators can be used in two forms:
- Prefix (e.g.,
++x
or--x
): The operation (increment or decrement) is applied before the value is returned or used in the expression. - Postfix (e.g.,
x++
orx--
): The current value is returned or used in the expression first, and then the operation (increment or decrement) is applied.
These operators can be very useful in loops and calculations but need careful handling to avoid unexpected results.
2. How the Prefix and Postfix Forms Work
Increment Operator (++)
- Prefix (
++x
): Increments the value ofx
by 1, then returns the new value. - Postfix (
x++
): Returns the current value ofx
, then incrementsx
by 1.
Decrement Operator (--)
- Prefix (
--x
): Decrements the value ofx
by 1, then returns the new value. - Postfix (
x--
): Returns the current value ofx
, then decrementsx
by 1.
3. Examples to Illustrate Prefix and Postfix Behavior
Let’s look at some examples to better understand how these operators work in both prefix and postfix forms.
Example 1: Basic Usage of ++ and --
let a = 5;
console.log(++a); // Prefix: a becomes 6, then prints 6
console.log(a); // Prints 6
let b = 5;
console.log(b++); // Postfix: prints 5, then b becomes 6
console.log(b); // Prints 6
In this example:
++a
(prefix) incrementsa
and returns the new value immediately.b++
(postfix) returns the original value ofb
first, then incrementsb
.
Example 2: Using Prefix and Postfix in an Expression
let x = 3;
let y = ++x + 2; // Prefix: x becomes 4, then y = 4 + 2
console.log(x); // Prints 4
console.log(y); // Prints 6
let m = 3;
let n = m++ + 2; // Postfix: n = 3 + 2, then m becomes 4
console.log(m); // Prints 4
console.log(n); // Prints 5
Here:
- Prefix (
++x
):x
is incremented before it’s added to 2. - Postfix (
m++
):m
is added to 2 beforem
is incremented.
4. Prefix and Postfix in Loops
In loops, the prefix and postfix increment/decrement operators are particularly useful and commonly seen. However, understanding their difference is essential to avoid unintended loop behavior.
Example 3: Prefix and Postfix in a for Loop
for (let i = 0; i < 5; ++i) {
console.log(i); // Prints 0, 1, 2, 3, 4
}
for (let j = 0; j < 5; j++) {
console.log(j); // Prints 0, 1, 2, 3, 4
}
In the case of a for
loop, both ++i
and i++
will behave the same way in terms of the loop’s outcome because the increment operation is the last part of the loop execution. However, in contexts where the result of the increment or decrement operation is immediately used, the distinction becomes critical.
5. Common Mistakes and How to Avoid Them
The differences between prefix and postfix operators can lead to mistakes, especially when these operators are used in complex expressions. Here are some common issues to be aware of:
- Unintended Value Changes: Since postfix operators use the current value before applying the increment/decrement, using
x++
instead of++x
can sometimes lead to unexpected values in expressions. - Ignoring the Operator’s Return Value: When using these operators in a function or expression, remember that prefix and postfix forms will return different values.
- Misunderstanding Order of Execution: Prefix and postfix operators are evaluated according to JavaScript’s left-to-right execution order, so consider this when chaining multiple operations.
6. Best Practices for Using Prefix and Postfix Operators
To avoid confusion and bugs, here are some best practices:
- Use Prefix for Immediate Results: If you need the updated value immediately, use the prefix form (
++x
or--x
). - Use Postfix in Standalone Statements: When you don’t need the returned value, the postfix form (
x++
orx--
) is generally safe to use. - Be Clear in Complex Expressions: When using increment or decrement operators in complex expressions, consider breaking the expression into multiple lines or statements to make the code easier to read and debug.
Summary
The prefix and postfix forms of the increment (++) and decrement (--) operators in JavaScript provide flexible ways to increase or decrease a variable's value. While these operators can be convenient, understanding how they differ is essential for writing clear, bug-free code.
- Prefix: Updates the variable first, then returns the new value.
- Postfix: Returns the current value first, then updates the variable.
By using these operators thoughtfully and understanding when each form is appropriate, you can write more efficient and readable code in JavaScript.