Understanding process.on in Node.js: A Comprehensive Guide
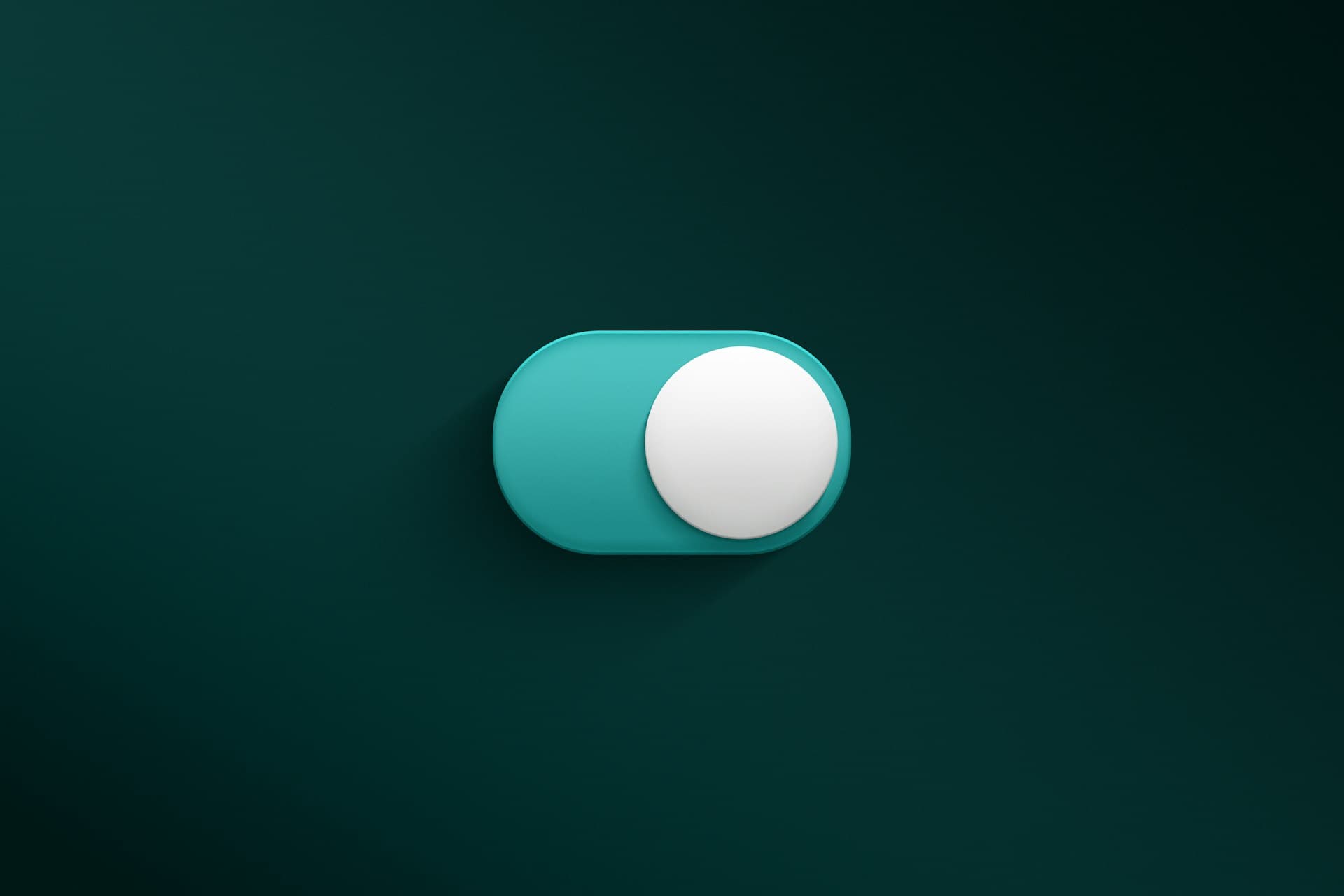
Table of Content
- What is process.on?
- Syntax
- Key Events and Real-World Use Cases
- 1. Handling `exit` Events
- 2. Graceful Shutdown with System Signals (`SIGINT`, `SIGTERM`)
- 3. Catching `uncaughtException`
- 4. Handling `warning` Events
- 5. Using Custom Events with `process.emit`
- Best Practices for Using process.on
- 1. Always Clean Up Resources
- 2. Avoid Relying on `uncaughtException`
- 3. Gracefully Shut Down Servers
- 4. Log Warnings for Proactive Debugging
- 5. Be Mindful of Asynchronous Operations
- Conclusion
Node.js is a powerful runtime for building server-side applications, and the
process
object plays a crucial role in managing the lifecycle of these applications. Among its many features, theprocess.on
method allows developers to handle important events during the lifecycle of a Node.js process.
This article provides a detailed explanation of process.on
, explores real-world use cases, and outlines best practices to follow for optimal application design.
What is process.on?
The process.on
method is a key API in Node.js that enables developers to listen for and respond to events emitted by the process
object. These events are crucial for managing application behavior in scenarios like unexpected errors, graceful shutdowns, or receiving system signals.
Syntax
process.on(eventName, listener);
eventName
: The name of the event to listen for (e.g.,'exit'
,'SIGINT'
,'uncaughtException'
).listener
: A callback function that will be invoked when the specified event is emitted.
Key Events and Real-World Use Cases
1. Handling exit
Events
The exit
event is emitted when the Node.js process is about to terminate. This is often used to clean up resources like file handles, database connections, or in-memory caches.
Example: Cleaning Up Database Connections
const db = require("./db"); // Assume this initializes a database connection
process.on("exit", (code) => {
console.log(`Process exiting with code: ${code}`);
db.close(); // Close database connection
});
Use Case: Ensures that all connections are properly closed to avoid potential resource leaks.
2. Graceful Shutdown with System Signals (SIGINT
, SIGTERM
)
When running a Node.js application in a production environment, it’s essential to handle termination signals (SIGINT
, SIGTERM
) for a clean shutdown. These signals are sent by the operating system when you terminate a process (e.g., pressing Ctrl+C or stopping a Docker container).
Example: Stopping an Express Server
const express = require("express");
const app = express();
const server = app.listen(3000, () => {
console.log("Server is running on port 3000");
});
const cleanup = () => {
console.log("Shutting down server...");
server.close(() => {
console.log("Server stopped.");
process.exit(0);
});
};
process.on("SIGINT", cleanup);
process.on("SIGTERM", cleanup);
Use Case: Ensures that servers stop accepting new requests and terminate gracefully, preventing potential data corruption or incomplete requests.
3. Catching uncaughtException
The uncaughtException
event is triggered when an unhandled error occurs. While it can be a lifesaver in preventing application crashes, relying on it is discouraged because it can leave your application in an inconsistent state.
Example: Logging Uncaught Exceptions
process.on("uncaughtException", (err) => {
console.error("An uncaught exception occurred:", err);
// Perform necessary cleanup
process.exit(1); // Exit with a non-zero status
});
Use Case: Logs critical errors for debugging but exits the process to prevent further unpredictable behavior.
4. Handling warning
Events
The warning
event is emitted for non-critical issues, such as deprecations or potential memory leaks. This can be useful for monitoring and addressing issues proactively.
Example: Monitoring Warnings
process.on("warning", (warning) => {
console.warn(`Warning: ${warning.name} - ${warning.message}`);
console.warn(warning.stack);
});
Use Case: Tracks warnings in production logs to ensure they are addressed during maintenance.
5. Using Custom Events with process.emit
Although process.on
is typically used with predefined events, it can also handle custom events emitted using process.emit
.
Example: Emitting Custom Events
process.on("customEvent", (message) => {
console.log(`Received custom event: ${message}`);
});
process.emit("customEvent", "Hello, custom event!");
Use Case: Enables internal messaging within the application.
Best Practices for Using process.on
1. Always Clean Up Resources
Handle events like exit
, SIGINT
, and SIGTERM
to clean up resources such as database connections, file handles, or external APIs.
process.on("exit", () => {
console.log("Cleaning up resources...");
// Perform cleanup
});
2. Avoid Relying on uncaughtException
Use structured error handling (e.g., try-catch
blocks) and middleware for predictable behavior. Use uncaughtException
only as a last resort.
process.on("uncaughtException", (err) => {
console.error("Unexpected error:", err);
process.exit(1); // Exit to prevent inconsistencies
});
3. Gracefully Shut Down Servers
Use signals like SIGINT
and SIGTERM
to handle graceful shutdowns, ensuring no in-flight requests are dropped.
4. Log Warnings for Proactive Debugging
Listen for the warning
event to catch potential issues like deprecations and memory leaks before they become critical.
5. Be Mindful of Asynchronous Operations
The exit
event does not wait for asynchronous operations to complete. Use synchronous cleanup logic or manage asynchronous tasks explicitly.
process.on("exit", () => {
console.log("Performing sync cleanup.");
});
Conclusion
The process.on
method is a powerful tool for managing the lifecycle and behavior of Node.js applications. By understanding and leveraging key events like exit
, SIGINT
, and uncaughtException
, developers can build robust, maintainable, and production-ready applications.
Following best practices, such as graceful shutdowns and proper resource cleanup, ensures your applications remain reliable and performant.
Whether you're handling unexpected crashes, managing server shutdowns, or monitoring application warnings,
process.on
is an essential part of every Node.js developer’s toolkit.