Understanding Recursion in JavaScript: A Professional Guide to Coding Basics
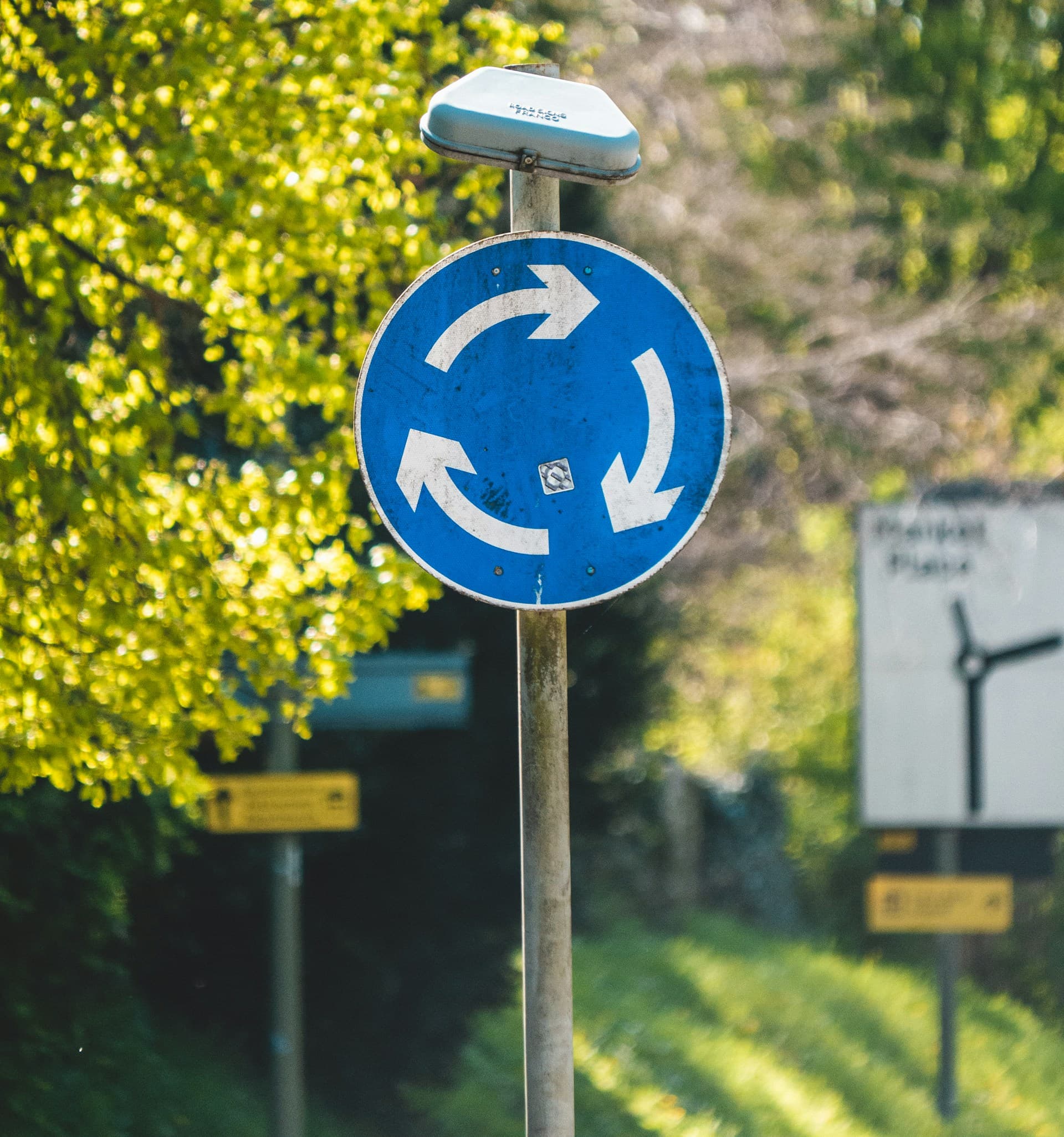
Table of Content
- What Is Recursion?
- Characteristics of Recursive Functions in JavaScript
- 1. Base Case
- 2. Recursive Case
- 3. Progress Toward Base Case
- 4. Stack Frames
- 5. Return Values
- 6. Termination
- 7. Direct or Indirect Recursion
- 8. Pure vs. Impure Recursion
- 9. Tail Recursion (Optimization)
- Real-World Use Cases of Recursion
- 1. Tree Traversal
- 2. Binary Search
- Conclusion
Recursion is a fundamental concept in programming that can solve complex problems elegantly and efficiently by breaking them down into smaller, more manageable parts. In JavaScript, recursion is implemented when a function calls itself to perform a task repeatedly, reducing the problem's complexity with each call until a base condition is met.
What Is Recursion?
Recursion is a programming technique where a function solves a problem by calling itself with a smaller subset of the problem. This process continues until a termination condition, known as the base case, is satisfied. A recursive function typically consists of:
- Base Case: The stopping condition that ends the recursion.
- Recursive Case: The logic that simplifies the problem and calls the function recursively.
Here’s a simple recursive function that calculates the factorial of a number:
function factorial(n) {
if (n === 0) return 1; // Base case
return n * factorial(n - 1); // Recursive case
}
console.log(factorial(5)); // Output: 120
Characteristics of Recursive Functions in JavaScript
1. Base Case
The base case is a condition that stops the recursion. Without it, the recursion will run indefinitely, causing a stack overflow. The base case is the simplest instance of the problem, where the solution is directly known.
Example: For summing numbers in a range, the base case could be when the starting number exceeds the ending number.
if (start > end) return total; // Base case for summing a range
2. Recursive Case
The recursive case is where the function calls itself with a smaller or simpler version of the problem. Each recursive call should work toward reaching the base case by simplifying the input.
Example: Adding the current number to the total and moving to the next number.
return recursiveSum(total + start, start + 1, end);
3. Progress Toward Base Case
To avoid infinite recursion, the problem must reduce in size or complexity with each recursive call. Failing to do so results in a stack overflow.
Example: Incrementing the starting number (start + 1
) ensures progress toward the base case.
4. Stack Frames
Each recursive call creates a new stack frame in memory, storing the function's local variables and state. When the base case is reached, the recursive calls start returning values back up the stack.
Example: For sumAll([1, 4])
, the call stack will look like this:
recursiveSum(0, 1, 4)
recursiveSum(1, 2, 4)
recursiveSum(3, 3, 4)
recursiveSum(6, 4, 4)
recursiveSum(10, 5, 4) (Base case, returns 10)
5. Return Values
A recursive function typically returns the result of its own recursive call, propagating the computed value back up the call stack.
Example:
return recursiveSum(total + start, start + 1, end); // Return is essential
6. Termination
A recursive function must terminate at some point; otherwise, it will lead to infinite recursion. Always ensure the input to the recursive function is modified so that the base case is eventually reached.
7. Direct or Indirect Recursion
-
Direct Recursion: The function directly calls itself.
function factorial(n) { if (n === 1) return 1; // Base case return n * factorial(n - 1); // Recursive case }
-
Indirect Recursion: A function calls another function, which then calls the original function.
function A(x) { if (x <= 0) return; return B(x - 1); } function B(x) { if (x <= 0) return; return A(x - 2); }
8. Pure vs. Impure Recursion
-
Pure Recursion: The function relies only on its arguments and returns values without side effects.
function sumRange(start, end) { if (start > end) return 0; // Base case return start + sumRange(start + 1, end); // Recursive case }
-
Impure Recursion: The function may involve side effects, like modifying a global variable.
let total = 0; function sumRangeImpure(start, end) { if (start > end) return; total += start; // Side effect sumRangeImpure(start + 1, end); }
9. Tail Recursion (Optimization)
Tail recursion is a form of recursion where the recursive call is the last operation performed by the function. While some languages optimize tail-recursive functions, JavaScript does not consistently do so.
Example:
function tailSum(start, end, total = 0) {
if (start > end) return total; // Base case
return tailSum(start + 1, end, total + start); // Recursive case
}
Real-World Use Cases of Recursion
1. Tree Traversal
Recursion is ideal for traversing tree-like structures such as the DOM, file systems, or organizational hierarchies.
Example: DOM Traversal
function traverseDOM(node) {
console.log(node.tagName); // Process the current node
node.children.forEach((child) => traverseDOM(child)); // Recursive call
}
traverseDOM(document.body); // Starts traversal from the <body> element
2. Binary Search
Binary search efficiently finds an element in a sorted array by repeatedly dividing the array into halves.
Example: Binary Search
function binarySearch(arr, target, low = 0, high = arr.length - 1) {
if (low > high) return -1; // Base case: Element not found
const mid = Math.floor((low + high) / 2);
if (arr[mid] === target) return mid; // Target found
if (arr[mid] > target) return binarySearch(arr, target, low, mid - 1); // Search left
return binarySearch(arr, target, mid + 1, high); // Search right
}
Conclusion
Recursion in JavaScript is a powerful tool for solving problems that can be broken into smaller subproblems. By understanding key concepts such as base cases, recursive cases, and stack frames, and applying best practices, you can implement robust and efficient recursive solutions for real-world tasks like tree traversal, search algorithms, and combinatorial problems.
Mastering recursion equips you to handle complex programming challenges elegantly, making it an indispensable skill for any JavaScript developer.