Top-Level Await in JavaScript: A Game-Changing Feature
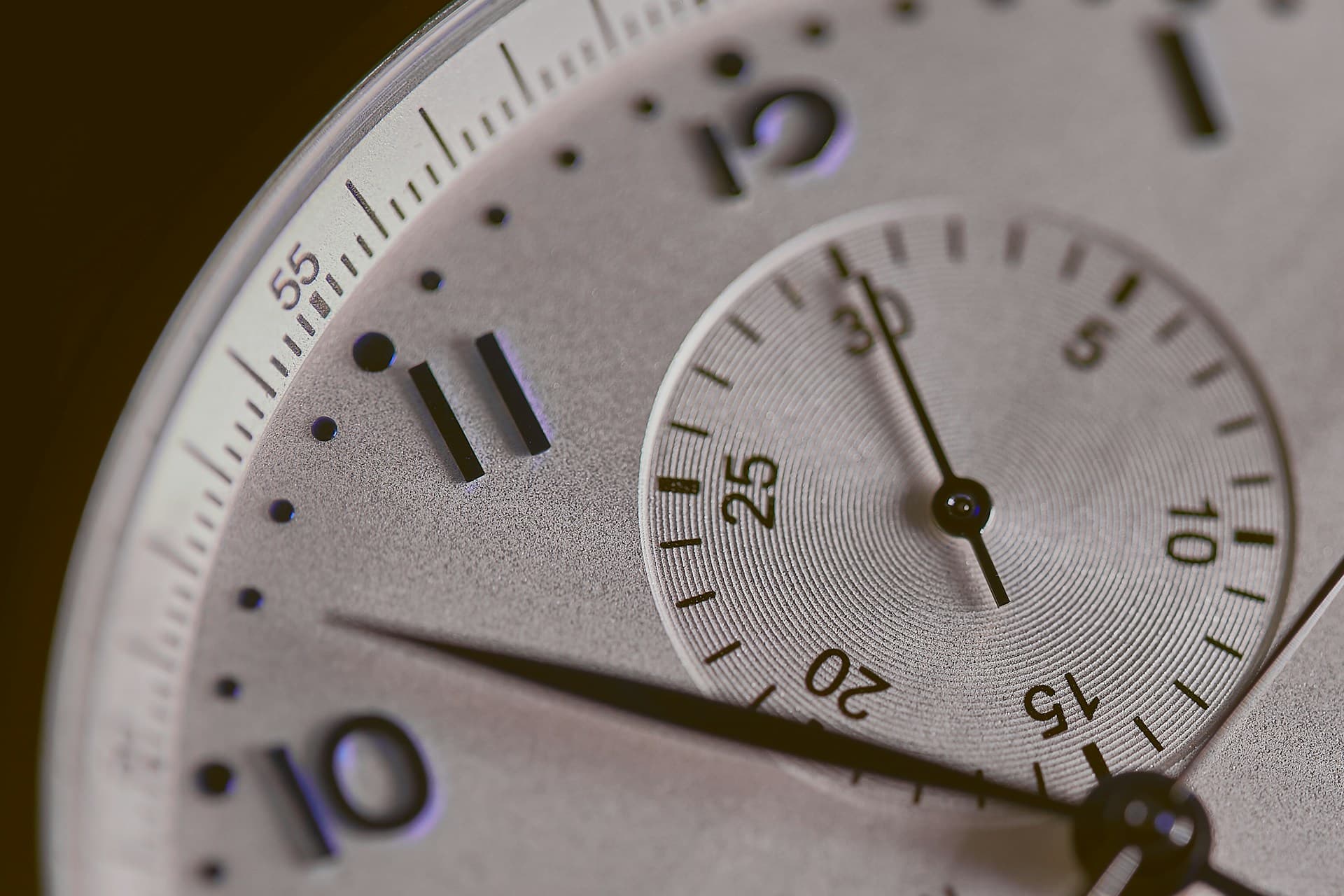
The Top-Level Await feature introduced in ECMAScript 2022 (ES13) allows developers to use the
await
keyword outside of asynchronous functions. This simplifies asynchronous code, especially in modular JavaScript, by enabling direct use ofawait
in the global scope. Let’s explore what this means, its advantages, and how it compares to older patterns.
Understanding the Need for Top-Level Await
Before Top-Level Await, using await
was only possible inside an asynchronous function. This limitation required workarounds to handle asynchronous operations in the module's top level, such as wrapping code in async
functions or leveraging immediately invoked async functions (IIFEs).
Problem Scenario: Fetching Data Before Module Execution
Imagine you're writing a module that fetches configuration data from an API before proceeding with the rest of your code. Here’s how you’d handle it before Top-Level Await:
// configModule.mjs
// Old Way: Using an Immediately Invoked Async Function
(async function () {
const response = await fetch("https://api.example.com/config");
const config = await response.json();
console.log("Config:", config);
// You would need to assign the value to some exported variable
// so other modules can access it later
module.exports = config;
})();
While effective, this pattern can be cumbersome. You’d need to rely on an IIFE or modular tricks to ensure the fetched data is available globally or exported for other modules to use.
The New Way: Top-Level Await
With Top-Level Await, you can write asynchronous code directly at the top level of your module without wrapping it in a function. Here’s how you can rewrite the same example:
// configModule.mjs
// New Way: Top-Level Await
const response = await fetch("https://api.example.com/config");
const config = await response.json();
console.log("Config:", config);
// Export the config for use in other modules
export default config;
This is simpler and more readable. The await
keyword is used directly, eliminating the need for additional boilerplate.
How Top-Level Await Works
Top-Level Await can only be used in JavaScript ES Modules (ESM). It pauses the execution of the module until the awaited promise resolves or rejects. If the module is imported into another module, the importer will wait until the imported module finishes executing.
Example of Module Dependency:
// dataModule.mjs
export const data = await fetch("https://api.example.com/data").then((res) =>
res.json(),
);
// app.mjs
import { data } from "./dataModule.mjs";
console.log("Fetched Data:", data);
Here, the app.mjs
module waits for the dataModule.mjs
module to resolve its await
before proceeding.
Benefits of Top-Level Await
- Improved Readability: Simplifies asynchronous code by eliminating boilerplate and nested function definitions.
- Streamlined Module Design: Modules can directly depend on asynchronous operations without additional setup or state management.
- Error Handling at Top-Level: Allows direct use of
try...catch
for better error handling in the global scope.
Example:
try {
const response = await fetch("https://api.example.com/data");
const data = await response.json();
console.log("Data:", data);
} catch (error) {
console.error("Failed to fetch data:", error);
}
Considerations and Limitations
- Execution Blocking: Since Top-Level Await pauses module execution, it can introduce performance bottlenecks if used excessively or improperly.
- Only in Modules: Top-Level Await does not work in CommonJS modules or scripts not designated as ES Modules.
- Careful Module Dependencies: Circular dependencies between modules using Top-Level Await can lead to deadlocks.
Comparison: Old vs. New
Feature | Old Way (Pre ES13) | New Way (Top-Level Await) |
---|---|---|
Code Complexity | Requires wrapping code in async /IIFEs | Directly use await at top level |
Readability | Can be cluttered with async wrappers | Cleaner and more readable |
Ease of Use in Modules | Requires explicit state management | Simplified, built-in async handling |
Conclusion
Top-Level Await is a powerful addition to JavaScript, especially for developers working with asynchronous operations in modular code. By allowing await
directly at the top level, it reduces boilerplate, improves readability, and simplifies workflows.
While adopting this feature, it’s essential to use it judiciously to avoid potential performance pitfalls. As more projects migrate to ES Modules, Top-Level Await is set to become a staple in modern JavaScript development.