Two Strategies for Typing Object Keys in TypeScript: A Deep Dive into Generics
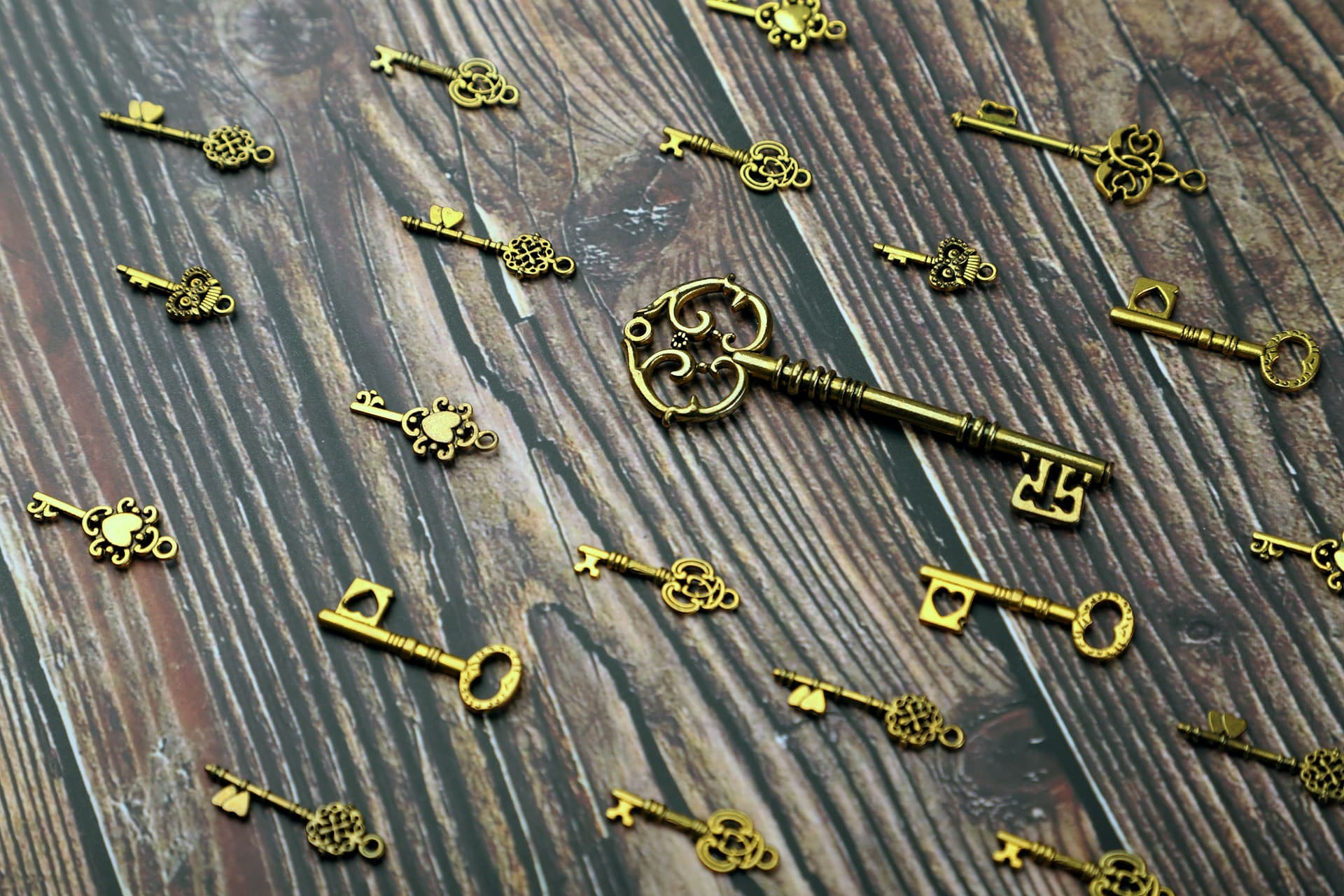
When building utility functions in TypeScript, you might often need to extract the keys of an object while preserving their types. There are two common approaches to typing such functions, and understanding both can help you decide which best fits your use case.
In this article, we’ll explore these two strategies with alternative examples and clear explanations.
Approach 1: Typing the Entire Object
In the first approach, we capture the whole object as a generic type parameter. This method ties the keys directly to the type of the object you pass in. However, you must be aware that the built-in Object.keys
method always returns a plain array of strings. To overcome this, we cast the result to a more precise type.
Consider the following example:
function extractKeys<T extends object>(obj: T): Array<keyof T> {
// Object.keys returns string[] by default, so we force the type to Array<keyof T>
return Object.keys(obj) as Array<keyof T>;
}
// Example usage:
interface User {
id: number;
name: string;
isActive: boolean;
}
const user: User = { id: 1, name: "Alice", isActive: true };
const keys = extractKeys(user);
// Type of keys: Array<"id" | "name" | "isActive">
How It Works
Generic Constraint : By declaring
<T extends object>
, we ensure that the function only accepts objects. Type Casting : SinceObject.keys(obj)
inherently returnsstring[]
, we cast it toArray<keyof T>
to reflect the actual keys of the object. Mapping : This approach maintains a one-to-one mapping between the properties of the input object and the returned keys.
Approach 2: Focusing Exclusively on the Keys
Sometimes you might care only about the keys themselves and not about the entire structure of the object. In this approach, the generic parameter is dedicated solely to representing the keys. To do this, we constrain the generic type to extend string
and use a Record
type for the input.
Here’s an alternative example:
function extractSpecificKeys<K extends string>(obj: Record<K, unknown>): K[] {
// We again cast the result of Object.keys, but now we know our keys are of type K
return Object.keys(obj) as K[];
}
// Example usage:
const settings = {
theme: "dark",
language: "en",
notifications: true,
};
const settingKeys = extractSpecificKeys(settings);
// Type of settingKeys: Array<"theme" | "language" | "notifications">
How It Works
Generic Constraint on Keys : We declare
<K extends string>
to ensure that the generic only captures string keys. Record Type : By typing the argument asRecord<K, unknown>
, we indicate that we don’t care about the values, only the keys. Precise Typing : This approach isolates the key extraction logic and directly maps the keys into the generic slot, resulting in a concise and clear function signature.
Comparing the Two Approaches
Approach 1: The Full Object Perspective
✅ Pros:
-
Provides a complete mapping between the object’s properties and the keys.
-
Useful when the context of the entire object is necessary elsewhere in your code.
❌ Cons:
-
The generic captures more information than needed if you’re only interested in the keys.
-
Requires an explicit cast to match the precise key types.
Approach 2: The Focused Key Perspective
✅ Pros:
-
Isolates the key extraction, making the intent crystal clear.
-
Results in a more elegant and focused type signature when only keys matter.
❌ Cons:
-
Limits the function to objects where the value types are irrelevant.
-
Doesn’t retain the broader context of the object’s full type.
Choosing the Right Strategy
The decision between these two strategies depends largely on your requirements:
-
Use Approach 1 if you want the generic to represent the entire object. This method is more idiomatic when the full type context is beneficial elsewhere in your code.
-
Use Approach 2 if you are solely interested in the keys. This approach is more straightforward and minimizes unnecessary complexity by focusing directly on the keys.
Both methods effectively leverage TypeScript generics to maintain type safety, so choose the one that aligns best with your design goals.
Conclusion
Mastering the art of generics in TypeScript often involves subtle decisions like these. Whether you choose to represent the whole object or just its keys, understanding the trade-offs will help you write clearer, more maintainable code. By tailoring your generic types to the specific parts of your data that matter, you can harness the full power of TypeScript’s type system. Happy coding! 🚀