Constrain to the Array Member, Not the Array: A TypeScript Generics Deep Dive
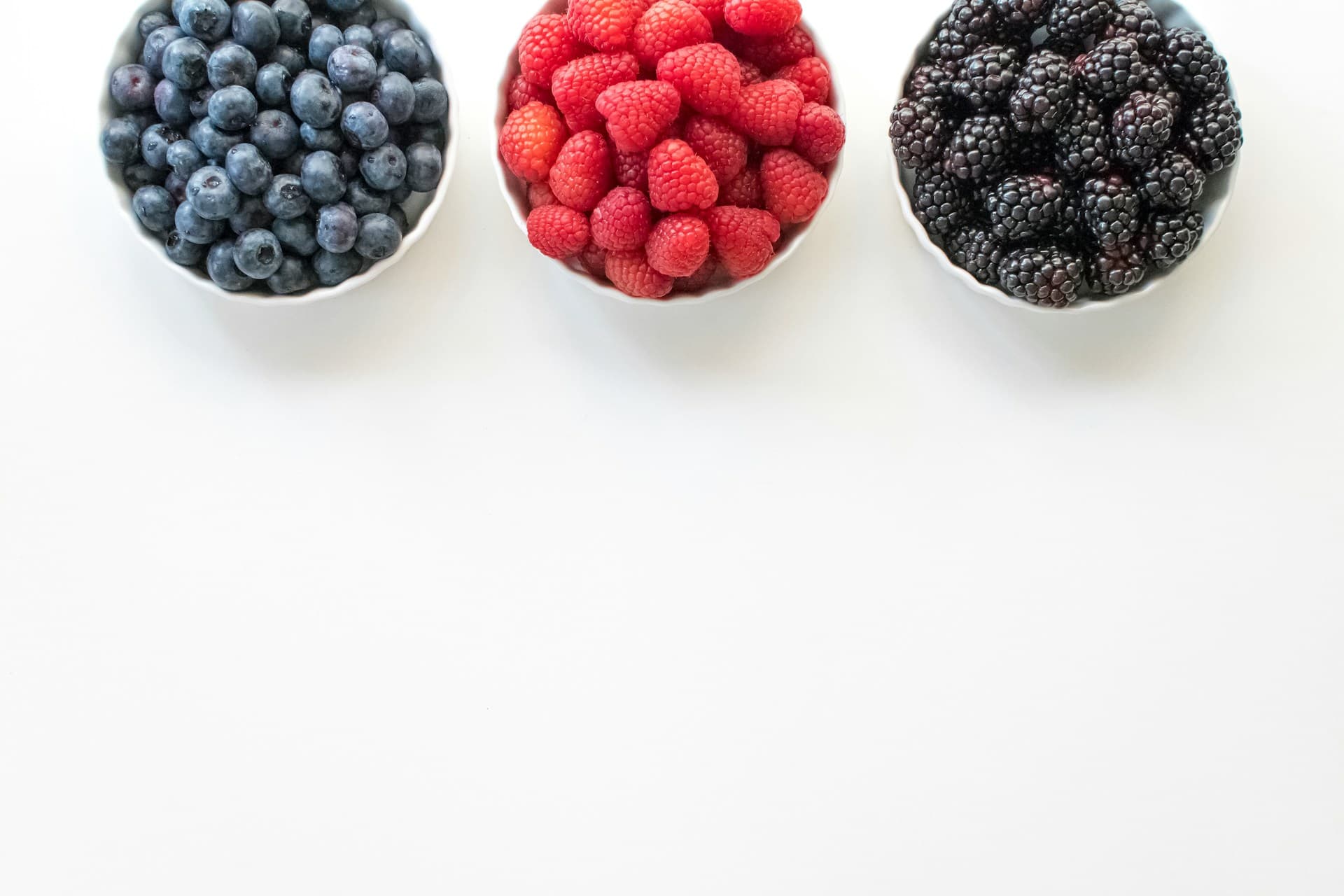
TypeScript’s powerful type inference system can sometimes be a double-edged sword. On one hand, it saves you from having to annotate everything manually; on the other, it may not always infer types as specifically as you’d like—especially when it comes to arrays.
In this article, we'll explore how tweaking your generics can lead to more precise type inference, using a different example to illustrate the concept.
The Problem: Inference on Entire Arrays
Imagine you want to create a function that defines a basket of fruits. You might start with something like this:
const createFruitBasket = <T extends string[]>(fruits: T) => {
return fruits;
};
At first glance, this seems like a straightforward approach. You provide an array of strings (fruit names), and TypeScript infers T
to be a string[]
. However, there’s a subtle catch: **Here’s your article formatted in **.mdx** format for publishing:
---
title: "Constrain to the Array Member, Not the Array: A TypeScript Generics Deep Dive"
description: "Learn how to improve TypeScript's type inference by constraining array members instead of entire arrays, leading to more precise and predictable types."
image: "../../public/posts/typescript-generics.jpg"
publishedAt: "2025/02/12"
updatedAt: "2025/02/12"
author: "Roy Lopez"
isPublished: true
tags:
- typescript
- generics
- type inference
---
TypeScript’s powerful type inference system can sometimes be a double-edged sword. On one hand, it saves you from having to annotate everything manually; on the other, it may not always infer types as specifically as you’d like—especially when it comes to arrays.
In this article, we'll explore how tweaking your generics can lead to more precise type inference, using a different example to illustrate the concept.
## The Problem: Inference on Entire Arrays
Imagine you want to create a function that defines a basket of fruits. You might start with something like this:
```typescript
const createFruitBasket = <T extends string[]>(fruits: T) => {
return fruits;
};
```
At first glance, this seems like a straightforward approach. You provide an array of strings (fruit names), and TypeScript infers T
to be a string[]
. However, there’s a subtle catch: TypeScript infers the type as just string[]
rather than the specific literal types of the fruits you passed in.**
For example, calling:
const basket = createFruitBasket(["apple", "banana", "cherry"]);
Results in basket
being inferred simply as string[]
—you lose the detail that it’s specifically an array containing "apple"
, "banana"
, and "cherry"
.
A More Granular Approach: Constrain the Array Member
To capture the literal types of each element in the array, you need to shift your focus from the array as a whole to its individual elements. Instead of constraining the entire array, you can constrain each member of the array . Here’s how you might refactor the function:
const createFruitBasket = <T extends string>(fruits: T[]) => {
return fruits;
};
Now, when you call:
const basket = createFruitBasket(["apple", "banana", "cherry"]);
TypeScript infers basket
as ("apple" | "banana" | "cherry")[]
.
This small change tells the compiler to look at each element of the array, preserving the specific literal types rather than generalizing them all to
string
.
Why This Matters
The lesson here is simple: always opt for the lowest-level representation that captures your intent .When you constrain a higher-level structure (like an array) without focusing on its constituents, you risk losing important type information. By specifying the constraint on the individual elements (i.e., T extends string
), you provide TypeScript with the hint it needs to infer the most precise types possible.This approach is particularly useful when you rely on the specific values later in your code. Whether you’re handling configuration options, command names, or fruit names , the precision in types can lead to fewer bugs and more predictable behavior .
Conclusion
When working with generics in TypeScript, it’s crucial to guide the compiler to infer types as deeply and accurately as possible. In our fruit basket example, switching from a generic array type to a generic element type preserved the literal values of the array items.
Remember: When given the choice, always constrain to the lowest level that represents the core values you care about. This technique can be a small but powerful tool in your TypeScript toolbox. Happy coding! 🚀