Understanding Buffers in Node.js: A Comprehensive Guide
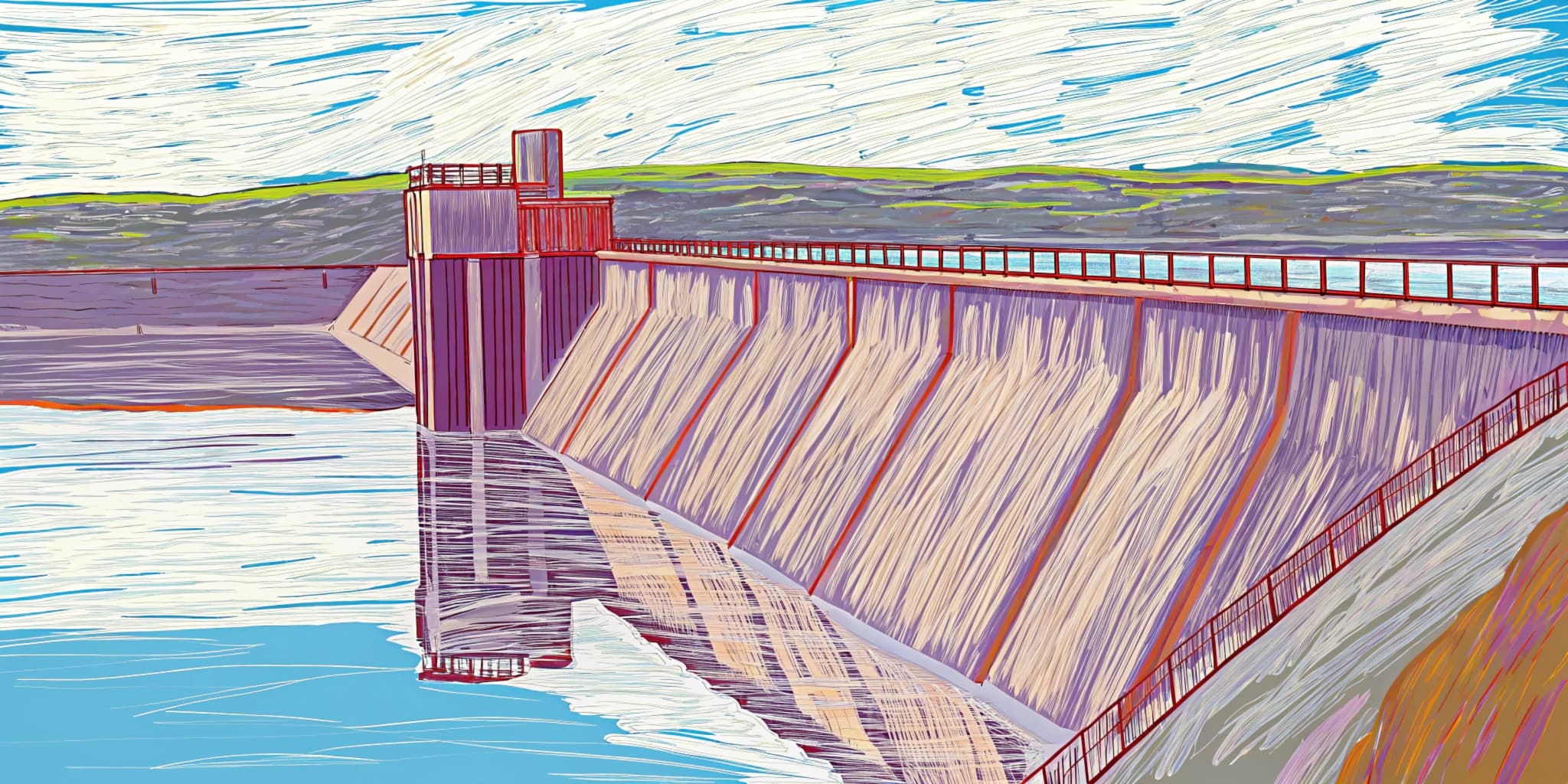
Table of Content
- What is a Buffer in Node.js?
- How Buffers Work
- Memory Allocation
- Buffer API
- Allocating a New Buffer
- Creating a Buffer from Existing Data
- Unsafe Buffer Allocation
- Encoding and Decoding
- Real-World Use Cases of Buffers
- File System Operations
- Networking
- Streaming Data
- Data Encoding/Decoding
- Cryptography
- Key Advantages of Using Buffers
- Best Practices When Using Buffers
- Conclusion
Node.js is a powerful platform for building scalable and efficient server-side applications. One of its standout features is its non-blocking I/O operations, which allow it to handle data streams efficiently. A critical piece of this functionality is the Buffer class, which is essential for working with binary data directly. In this article, we’ll explore what a Buffer is, how it works, and its real-world applications in Node.js.
What is a Buffer in Node.js?
A Buffer is a temporary storage area for raw binary data. It’s similar to an array of integers but is specifically designed to handle binary data. Unlike JavaScript strings, which are Unicode-based, Buffers are sequences of bytes. This makes Buffers particularly useful for handling data that comes from or goes to a stream (like files, network connections, or other I/O operations) where you might not know the total size of the data upfront.
Buffers are part of the buffer
module in Node.js and are globally available, meaning you can use them without requiring additional imports.
How Buffers Work
Memory Allocation
When you create a Buffer, Node.js allocates a fixed amount of memory outside the V8 heap (the engine’s memory space for JavaScript objects). This helps manage large data efficiently without stressing the garbage collector. Buffers are sized in bytes and cannot be resized once created.
Buffer API
The Buffer API offers various methods for creating, reading, writing, and manipulating binary data. Some common ways to create a Buffer include:
Allocating a New Buffer
const buf = Buffer.alloc(10); // Creates a Buffer of size 10, initialized to zeros
Creating a Buffer from Existing Data
const buf = Buffer.from("Hello, World!"); // Converts a string into a Buffer
Unsafe Buffer Allocation
const buf = Buffer.allocUnsafe(10); // Creates an uninitialized Buffer (faster but may contain old data)
Encoding and Decoding
Buffers can encode and decode data in various formats, such as UTF-8, Base64, or hexadecimal. This makes them highly versatile for tasks like converting data formats or encoding data for transmission.
Real-World Use Cases of Buffers
File System Operations
Buffers are commonly used when reading or writing files in binary formats. For example, reading an image or video file requires handling raw data efficiently:
const fs = require("fs");
fs.readFile("example.png", (err, data) => {
if (err) throw err;
console.log(data); // Logs raw binary data as a Buffer
});
Networking
Buffers are critical when dealing with TCP or UDP sockets. Network packets are received as binary streams, and Buffers help in assembling or parsing them.
const net = require("net");
const server = net.createServer((socket) => {
socket.on("data", (chunk) => {
console.log(`Received: ${chunk.toString()}`);
});
});
server.listen(8080, () => console.log("Server running on port 8080"));
Streaming Data
Streams in Node.js process data incrementally, and Buffers are used to temporarily store chunks of data before they are processed or sent further. For example, when streaming video content:
const fs = require("fs");
const readStream = fs.createReadStream("video.mp4");
readStream.on("data", (chunk) => {
console.log(`Received ${chunk.length} bytes of data`);
});
Data Encoding/Decoding
Buffers allow seamless conversion between data formats, such as converting a binary image to a Base64-encoded string:
const fs = require("fs");
const imageBuffer = fs.readFileSync("example.png");
const base64Image = imageBuffer.toString("base64");
console.log(base64Image);
Cryptography
Cryptographic operations often require handling raw binary data. Buffers are essential for tasks like generating hashes or encrypting data:
const crypto = require("crypto");
const hash = crypto.createHash("sha256");
hash.update("Some data to hash");
const result = hash.digest("hex");
console.log(result); // Logs the hashed value
Key Advantages of Using Buffers
- Performance: Buffers provide direct access to memory, bypassing the overhead of JavaScript’s higher-level abstractions.
- Stream Compatibility: Since streams work with chunks of data, Buffers complement them perfectly for managing partial data flows.
- Flexibility: Buffers support various encodings and offer a rich API for manipulation.
Best Practices When Using Buffers
- Use
Buffer.alloc
Instead ofBuffer.allocUnsafe
: Always useBuffer.alloc
for security, as it initializes memory to zero. UseBuffer.allocUnsafe
only when performance is critical, and you can ensure old data doesn’t leak. - Limit Buffer Sizes: Avoid creating overly large Buffers to prevent memory exhaustion.
- Handle Encoding Explicitly: Always specify the encoding when converting Buffers to strings or vice versa.
const buf = Buffer.from("Hello", "utf8");
console.log(buf.toString("utf8")); // Explicitly specifies UTF-8 encoding
Conclusion
Buffers are an indispensable part of Node.js for managing binary data efficiently. Whether you’re working with file systems, streams, or cryptographic operations, understanding Buffers and their API is crucial for writing performant and reliable applications. By leveraging Buffers effectively, you can build applications that handle raw data seamlessly and operate at peak efficiency.