Understanding the is Operator in TypeScript: A Comprehensive Guide
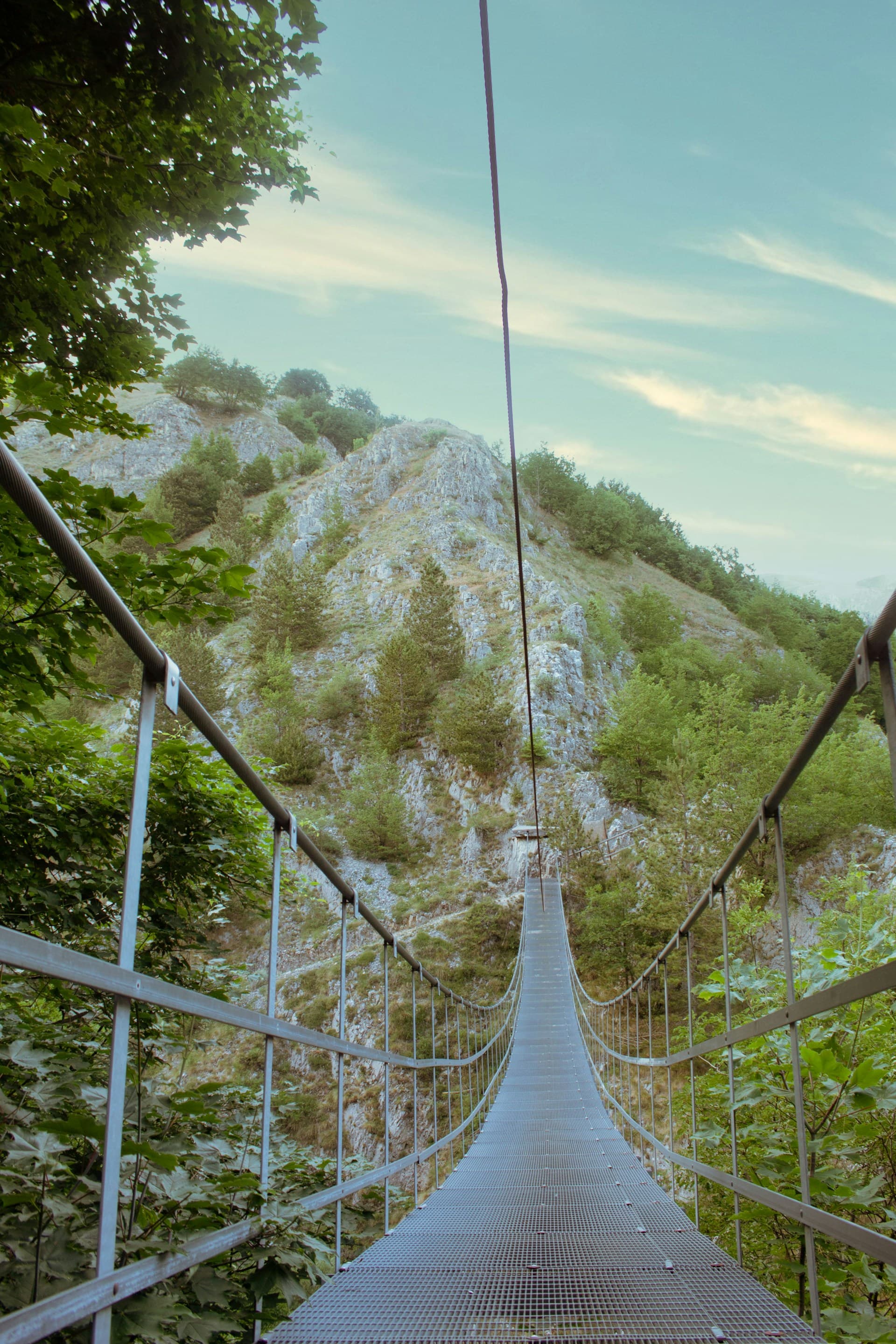
Table of Content
- What is the is Operator?
- Syntax:
- Why Use the is Operator?
- Creating a Type Guard with the is Operator
- Example: Type Guard for Discriminated Union
- Key Points:
- Use Cases for the is Operator
- **1. Refining Union Types**
- **2. Validating API Responses**
- **3. Checking for Optional Properties**
- Best Practices for Using the is Operator
- Common Pitfalls and How to Avoid Them
- Conclusion
TypeScript’s static type system is one of its most powerful features, enabling developers to write safer and more predictable code. Among its tools is the is operator, used in custom type guard functions to narrow types effectively. This article explores what the is operator is, how it works, and its practical applications in real-world TypeScript projects.
What is the is Operator?
The is operator in TypeScript is a return type modifier used in custom type guard functions. It helps inform the TypeScript compiler about the refined type of a variable when a specific condition is met.
Syntax:
variable is Type
A function using the is
operator must return a boolean value. If the function returns true
, TypeScript treats the variable as the specified type within the condition's scope.
Why Use the is Operator?
The is operator offers several advantages:
- Custom Type Guards: Enables reusable logic for determining types that TypeScript cannot infer.
- Improved Code Readability: Encapsulates complex type-checking logic for better expressiveness and maintainability.
- Enhanced Type Safety: Leverages TypeScript's control flow analysis to reduce the need for manual assertions (
as
).
Creating a Type Guard with the is Operator
A type guard function with the is
operator typically examines a variable’s structure or properties to determine its type.
Example: Type Guard for Discriminated Union
interface Cat {
name: string;
meow: () => void;
}
interface Dog {
name: string;
bark: () => void;
}
function isCat(animal: Cat | Dog): animal is Cat {
return (animal as Cat).meow !== undefined;
}
function interactWithAnimal(animal: Cat | Dog) {
if (isCat(animal)) {
animal.meow(); // TypeScript knows `animal` is a Cat.
} else {
animal.bark(); // TypeScript knows `animal` is a Dog.
}
}
Key Points:
- Logic: The
isCat
function checks for the presence of themeow
method, unique toCat
. - Behavior: The
is
operator signals TypeScript that when the function returnstrue
, the input is of typeCat
.
Use Cases for the is Operator
1. Refining Union Types
The is operator elegantly narrows union types based on custom conditions.
type Vehicle =
| { type: "car"; speed: number }
| { type: "boat"; capacity: number };
function isCar(vehicle: Vehicle): vehicle is { type: "car"; speed: number } {
return vehicle.type === "car";
}
function describeVehicle(vehicle: Vehicle) {
if (isCar(vehicle)) {
console.log(`Car with speed ${vehicle.speed}`);
} else {
console.log(`Boat with capacity ${vehicle.capacity}`);
}
}
2. Validating API Responses
Validate and narrow API responses dynamically.
interface ApiResponseSuccess {
success: true;
data: string[];
}
interface ApiResponseError {
success: false;
error: string;
}
type ApiResponse = ApiResponseSuccess | ApiResponseError;
function isSuccess(response: ApiResponse): response is ApiResponseSuccess {
return response.success === true;
}
function handleApiResponse(response: ApiResponse) {
if (isSuccess(response)) {
console.log("Data:", response.data);
} else {
console.log("Error:", response.error);
}
}
3. Checking for Optional Properties
The is operator can verify optional properties and refine types.
interface Person {
name: string;
age?: number;
}
function hasAge(person: Person): person is Person & { age: number } {
return person.age !== undefined;
}
const john: Person = { name: "John", age: 30 };
const jane: Person = { name: "Jane" };
if (hasAge(john)) {
console.log(`${john.name} is ${john.age} years old.`);
}
if (!hasAge(jane)) {
console.log(`${jane.name} has an unknown age.`);
}
Best Practices for Using the is Operator
- Keep Type Guards Simple: Write concise and straightforward logic to ensure clarity.
- Use for Reusability: Encapsulate complex checks in type guard functions to improve organization.
- Combine with Discriminated Unions: Use the is operator to systematically handle multiple types.
- Validate Runtime Behavior: Ensure type guard logic matches runtime behavior to avoid errors.
- Leverage IDE Assistance: Use TypeScript-aware IDEs like VS Code to visualize type narrowing.
Common Pitfalls and How to Avoid Them
- Overconfidence in Assertions: Avoid runtime mismatches by ensuring type guard logic reflects real-world data structures.
- Ignoring Exhaustive Checks: Always account for all possible cases in union types to prevent unhandled scenarios.
- Relying on
as
: Use type guards instead ofas
to leverage TypeScript's type-checking capabilities.
Conclusion
The is operator is a cornerstone of custom type guards in TypeScript, empowering developers to handle complex type-checking scenarios with precision and clarity. Mastering its use will enhance the safety and maintainability of your code, leveraging the full potential of TypeScript's type system.
Incorporate the is operator into your TypeScript toolkit to build robust applications and minimize type-related runtime errors.