Understanding the ||= Operator in JavaScript: Simplifying Default Value Assignments
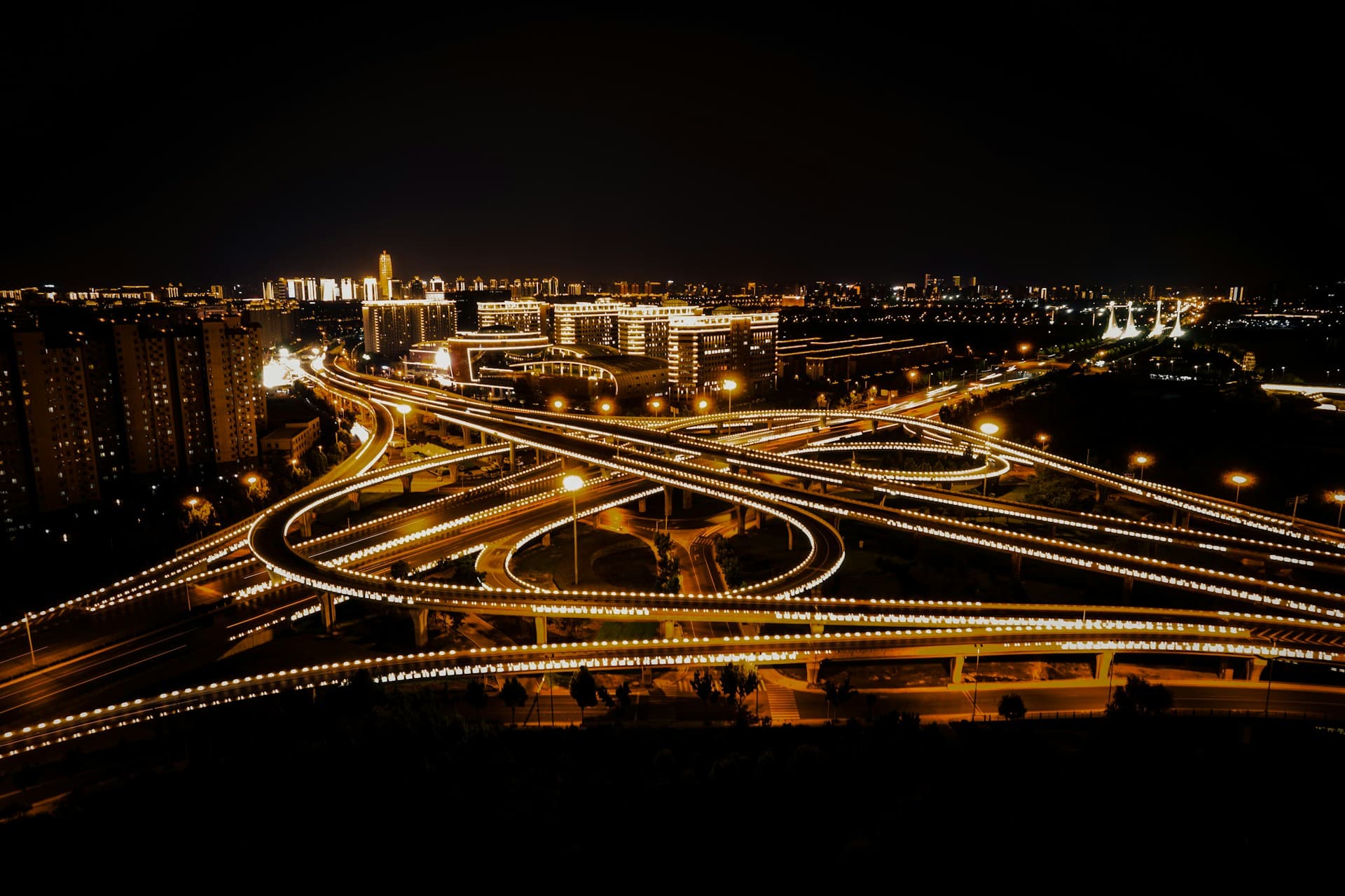
The ||=
operator in JavaScript, also known as the Logical OR Assignment Operator, is a powerful shorthand for assigning values to variables only when their current values are "falsy." This operator combines a logical OR operation with assignment, streamlining a common coding pattern and making your code more concise and readable.
In this article, we'll explore the syntax, behavior, and practical applications of the ||=
operator, complete with examples and use cases.
Syntax and Behavior
The basic syntax of the
||=
operator is as follows:x ||= y;
This is functionally equivalent to:
x || (x = y);
How It Works
- Evaluation: The left-hand side operand (
x
) is evaluated first. - Assignment: If
x
is falsy (e.g.,false
,0
,null
,undefined
,NaN
, or an empty string), the value ofy
is assigned tox
. - Short-circuiting: If
x
is truthy, the assignment does not occur, andy
is not evaluated. This ensures efficient execution.
Examples
Basic Usage
Let's look at an example to understand how the ||=
operator works in practice:
let userName = { firstName: "Alice", lastName: "" };
// Using logical OR assignment operator
userName.firstName ||= "Bob"; // No change, as firstName is truthy
console.log(userName.firstName); // Output: "Alice"
userName.lastName ||= "Smith"; // Changes, as lastName is falsy
console.log(userName.lastName); // Output: "Smith"
What’s Happening Here?
- The value of
firstName
remains"Alice"
because it is truthy.- The value of
lastName
changes to"Smith"
because its initial value (""
) is falsy.
Common Use Cases
1. Setting Default Values
The ||=
operator is ideal for assigning default values when variables are uninitialized or set to falsy values.
let config = { timeout: undefined, retries: 0 };
// Default timeout
config.timeout ||= 3000; // Assigns 3000, as timeout is undefined
console.log(config.timeout); // Output: 3000
// Retries remain 0 because 0 is falsy but intentional
config.retries ||= 5;
console.log(config.retries); // Output: 5
2. Conditional Initialization
When working with objects or variables that might not be set, the ||=
operator simplifies initialization.
let settings = {};
settings.theme ||= "dark"; // Initializes theme only if it's undefined or falsy
console.log(settings.theme); // Output: "dark"
3. Fallback for User Input
This operator is helpful when you need to ensure that user inputs have valid default values.
let userInput = "";
userInput ||= "Default Value"; // Assigns default when input is empty
console.log(userInput); // Output: "Default Value"
Advantages of the ||=
Operator
- Conciseness: Reduces boilerplate code by combining logical OR and assignment in a single expression.
- Readability: Makes the intent clear—assigning a value only if the current one is falsy.
- Efficiency: Leverages short-circuiting, avoiding unnecessary evaluations.
Caveats and Considerations
1. Falsy vs. Intentional Values
The ||=
operator treats all falsy values (like 0
, false
, and ""
) the same. If you need to distinguish between these values, consider using a different approach.
let score = 0;
score ||= 100; // Replaces 0 with 100, which might not be desired
console.log(score); // Output: 100
2. Type Compatibility
Ensure that the types of x
and y
are compatible with your intended use, as mismatched types could lead to unexpected behavior.
let count;
count ||= "Not a Number"; // Works, but might introduce unintended type issues
console.log(count); // Output: "Not a Number"
Conclusion
The ||=
operator is a versatile tool in JavaScript, allowing developers to write cleaner and more efficient code for assigning default values and initializing variables. By understanding its behavior and use cases, you can simplify your code and reduce the risk of common logical errors.
However, it's essential to use it thoughtfully, especially when handling falsy values like 0
or false
.
By integrating the ||=
operator into your coding practices, you can make your JavaScript code both concise and expressive—hallmarks of modern, maintainable programming.