Understanding Streams in Node.js: A Comprehensive Guide with Real-World Examples
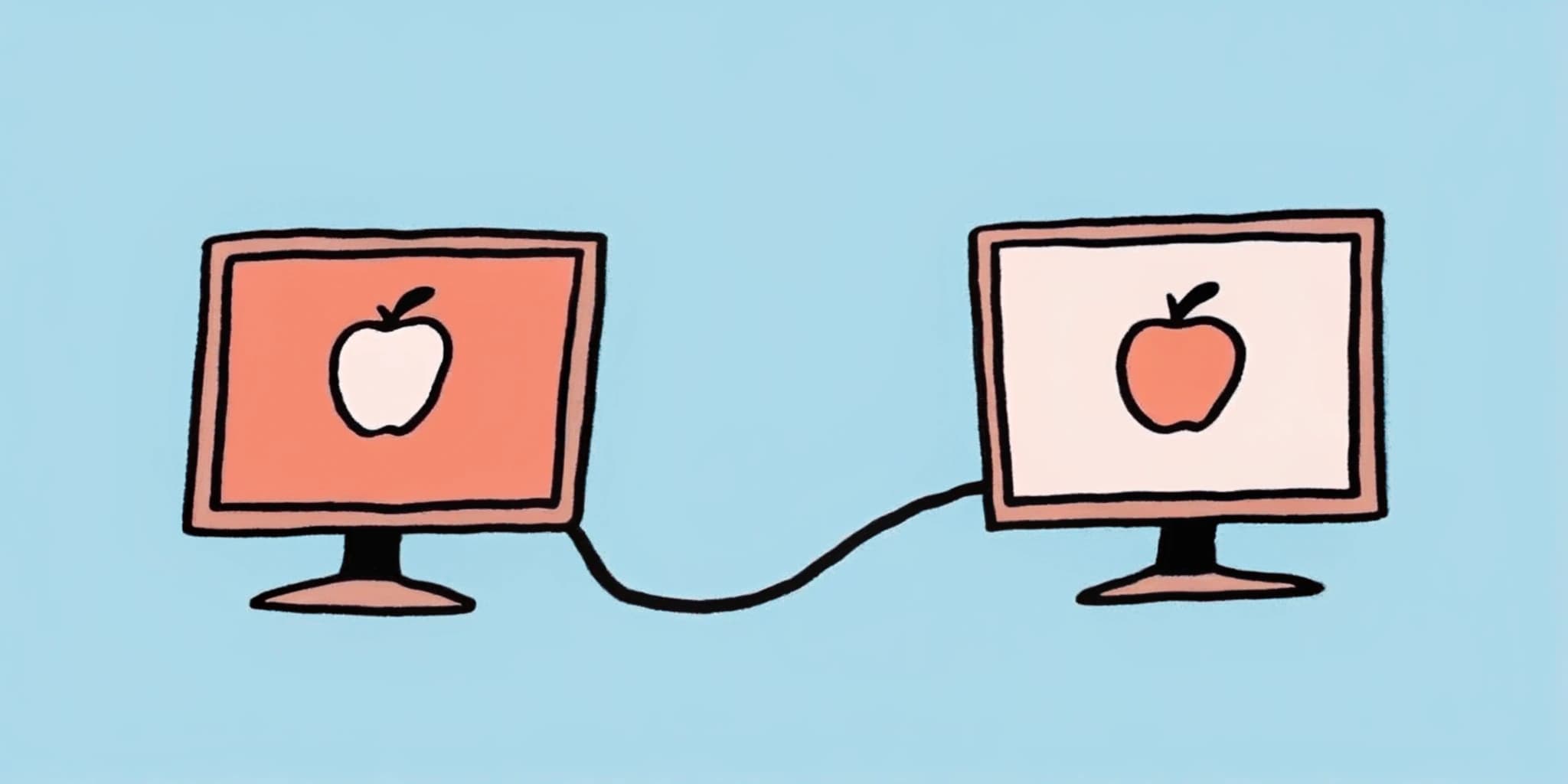
Table of Content
- What are Streams in Node.js?
- Why Use Streams?
- Types of Streams in Node.js
- How Streams Work: Events and Modes
- Key events to know:
- Working with Streams: Real-World Examples
- 1. Reading a Large File
- 2. Writing to a File
- 3. Piping Streams
- Example: Copying a File
- 4. Transform Streams: Compressing a File
- When to Use Streams in Your Application
- Conclusion
Streams are one of the most powerful and efficient features of Node.js. They enable processing large amounts of data piece by piece (chunk by chunk) without requiring the entire dataset to be loaded into memory. This makes them an essential tool for building scalable and efficient applications, especially those that deal with large files or continuous data transfer.
In this article, we’ll explore how streams work in Node.js, their types, and real-world applications to demonstrate their practicality.
What are Streams in Node.js?
In Node.js, streams are objects that allow data to be read or written in a sequential manner. They are instances of the EventEmitter
class and leverage events like data
, end
, error
, and finish
to handle their operations.
Streams come in handy when dealing with:
- File processing (e.g., reading and writing large files)
- Network communications (e.g., HTTP requests and responses)
- Real-time data transfer (e.g., video or audio streaming)
Why Use Streams?
- Memory Efficiency: Streams process data in chunks, not all at once, avoiding high memory consumption.
- Time Efficiency: Data is processed as it arrives, reducing latency.
- Scalability: Ideal for applications requiring continuous data flow.
Types of Streams in Node.js
- Readable Streams: Used for reading data sequentially. Examples include reading files, receiving HTTP requests, and reading from databases.
- Writable Streams: Used for writing data sequentially. Examples include writing files, sending HTTP responses, and logging data.
- Duplex Streams: Can read and write data. Examples include network sockets.
- Transform Streams: A special type of Duplex stream that can modify or transform the data as it is read or written. Examples include compression and encryption.
How Streams Work: Events and Modes
Streams in Node.js operate in two modes:
- Flowing Mode: Data is read automatically and provided via events.
- Paused Mode: Data must be explicitly read using methods like
.read()
.
Key events to know:
data
: Emitted when a chunk of data is available.end
: Emitted when there is no more data to read.error
: Emitted when an error occurs during reading or writing.finish
: Emitted when all data has been flushed to the underlying system.
Working with Streams: Real-World Examples
1. Reading a Large File
Instead of loading an entire file into memory, use a Readable stream to process it chunk by chunk.
const fs = require("fs");
// Create a readable stream
const readableStream = fs.createReadStream("largefile.txt", {
encoding: "utf8",
});
// Handle events
readableStream.on("data", (chunk) => {
console.log("Received chunk:", chunk);
});
readableStream.on("end", () => {
console.log("File reading completed.");
});
readableStream.on("error", (err) => {
console.error("Error reading file:", err);
});
2. Writing to a File
Writable streams allow incremental writing to files.
const fs = require('fs');
// Create a writable stream
const writableStream = fs.createWriteStream('output.txt');
// Write data in chunks
writableStream.write('This is the first line.
');
writableStream.write('This is the second line.
');
// End the stream
writableStream.end(() => {
console.log('Data has been written to the file.');
});
3. Piping Streams
One of the most powerful features of Node.js streams is the ability to pipe them.
Example: Copying a File
const fs = require("fs");
// Create readable and writable streams
const readableStream = fs.createReadStream("source.txt");
const writableStream = fs.createWriteStream("destination.txt");
// Pipe the streams
readableStream.pipe(writableStream);
writableStream.on("finish", () => {
console.log("File copied successfully.");
});
4. Transform Streams: Compressing a File
Transform streams are ideal for modifying data as it passes through.
const fs = require("fs");
const zlib = require("zlib");
// Create a readable stream
const readableStream = fs.createReadStream("input.txt");
// Create a writable stream
const compressedStream = fs.createWriteStream("input.txt.gz");
// Create a transform stream
const gzip = zlib.createGzip();
// Pipe streams together
readableStream.pipe(gzip).pipe(compressedStream);
compressedStream.on("finish", () => {
console.log("File has been compressed.");
});
When to Use Streams in Your Application
Streams are particularly useful when:
- Handling large files (e.g., log processing, backups).
- Working with real-time data (e.g., chat applications, video streaming).
- Building APIs or services that deal with continuous data flows (e.g., IoT).
Conclusion
Streams in Node.js are a cornerstone for building efficient and scalable applications. By leveraging their ability to process data incrementally, you can significantly reduce memory overhead and improve application performance. Whether you're working with files, network data, or real-time streaming, Node.js streams provide a robust solution.
Understanding how to use streams effectively, as shown in the examples above, will empower you to handle complex data-processing tasks with ease. So, start experimenting with streams in your projects and see the difference they can make!