Understanding the Variadic Syntax (...) in Go: A Comprehensive Guide
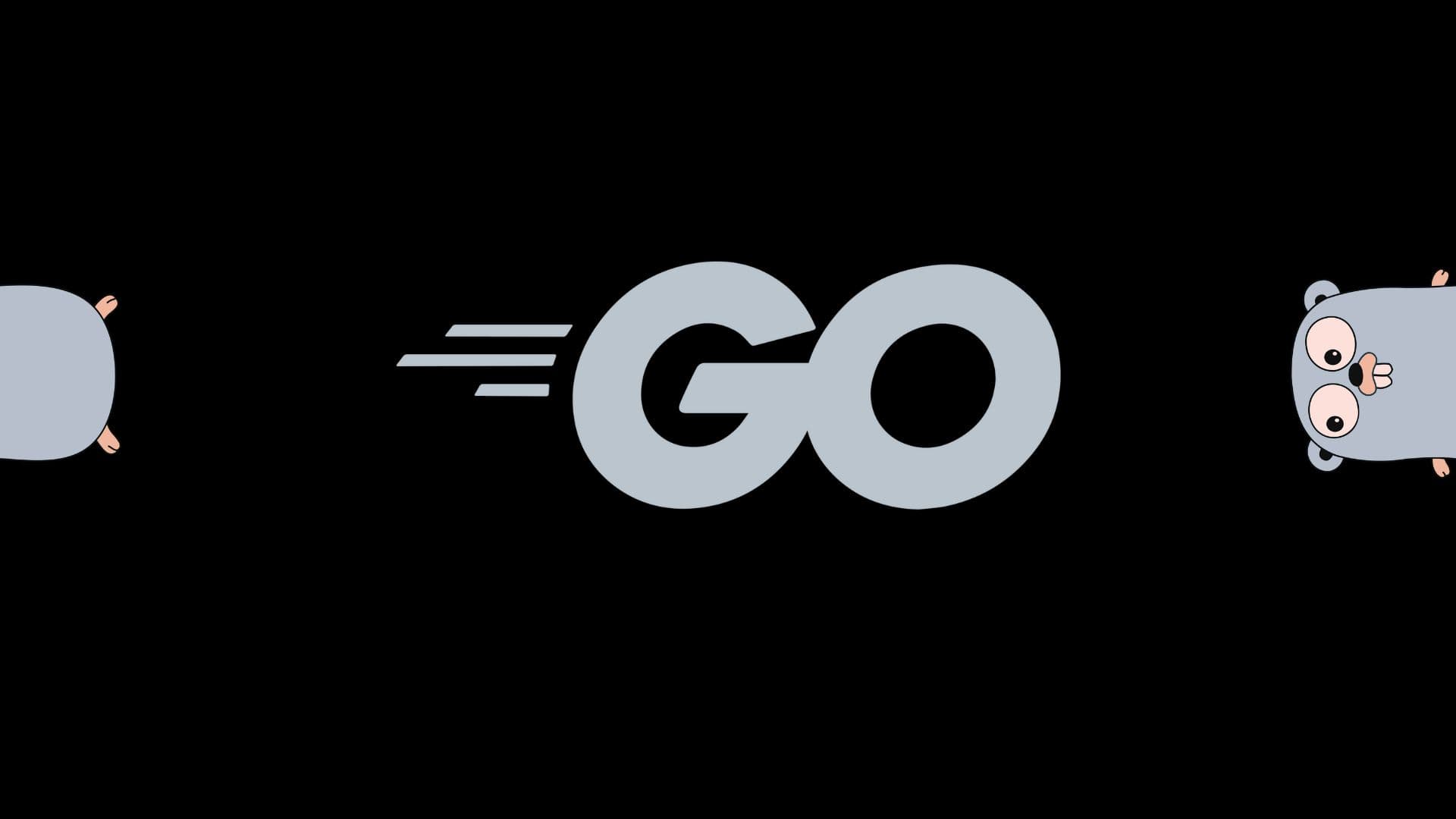
Table of Content
- What is the `...` Syntax?
- Example 1: A Simple Variadic Function
- Example 2: Variadic Functions with Different Types
- Example 3: Passing a Slice to a Variadic Function
- Example 4: Variadic Functions with Mixed Types
- Important Rules for Variadic Parameters:
- Example 5: Using Variadic Parameters with Structs
- Summary:
- LinkedIn Copy
In Go, the ...
syntax, also known as variadic parameters, allows you to create functions that accept a variable number of arguments. This feature is extremely useful when you don’t know the exact number of arguments that will be passed to the function. In this article, we will explore how the ...
syntax works, its common use cases, and examples to clarify the concept.
What is the ...
Syntax?
In Go, the ...
is used to define a variadic parameter. A variadic parameter lets you pass zero, one, or multiple arguments of the same type to a function. When you declare a variadic parameter, Go automatically collects these arguments into a slice.
The syntax for a variadic parameter is:
func functionName(paramType ...dataType) {
// function body
}
Here, paramType
is the name of the parameter, and dataType
is the type of the variable-length argument.
Example 1: A Simple Variadic Function
Let’s start with a simple function that accepts a variable number of integers and calculates their sum:
package main
import (
"fmt"
)
func sum(numbers ...int) int {
total := 0
for _, num := range numbers {
total += num
}
return total
}
func main() {
fmt.Println(sum(1, 2, 3)) // Output: 6
fmt.Println(sum(10, 20)) // Output: 30
fmt.Println(sum(5, 5, 5, 5, 5)) // Output: 25
fmt.Println(sum()) // Output: 0
}
In the sum
function:
numbers ...int
allows the function to accept any number ofint
arguments.- Inside the function,
numbers
is treated as a slice of integers ([]int
), and we can iterate over it with afor
loop.
Example 2: Variadic Functions with Different Types
A variadic function can also have regular parameters alongside the variadic ones. These regular parameters must always come before the variadic parameters in the function signature.
Here’s an example where we have a regular prefix
string parameter followed by a variadic words ...string
parameter:
package main
import "fmt"
func printWords(prefix string, words ...string) {
fmt.Println(prefix)
for _, word := range words {
fmt.Println(word)
}
}
func main() {
printWords("Words:", "Go", "is", "awesome")
// Output:
// Words:
// Go
// is
// awesome
printWords("More Words:", "Variadic", "functions", "are", "cool")
// Output:
// More Words:
// Variadic
// functions
// are
// cool
printWords("Empty Call:") // No variadic arguments passed
// Output:
// Empty Call:
}
In this example:
- The
prefix
is a regular parameter. - The
words ...string
is the variadic parameter, and we can pass any number of strings to it. When no additional arguments are passed,words
is an empty slice ([]string
).
Example 3: Passing a Slice to a Variadic Function
If you already have a slice of elements and want to pass it to a variadic function, you can "unpack" the slice into individual arguments using the ...
operator.
package main
import "fmt"
func printNumbers(numbers ...int) {
for _, num := range numbers {
fmt.Println(num)
}
}
func main() {
nums := []int{1, 2, 3, 4, 5}
// Pass individual elements
printNumbers(1, 2, 3)
// Pass the entire slice with the '...' spread operator
printNumbers(nums...)
// Output:
// 1
// 2
// 3
// 1
// 2
// 3
// 4
// 5
}
In this example:
nums...
unpacks the slicenums
and passes each element as an individual argument to theprintNumbers
function.- If you omit
...
, the slice will be passed as a single argument, which would cause a compilation error because the function expects a variable number of individual integers.
Example 4: Variadic Functions with Mixed Types
You can use variadic parameters with any type. Here’s an example with mixed types, where we pass multiple values to a logger function that prints messages with different severity levels:
package main
import "fmt"
// Logger function with a variadic string parameter
func logMessage(level string, messages ...string) {
fmt.Printf("[%s] ", level)
for _, msg := range messages {
fmt.Printf("%s ", msg)
}
fmt.Println()
}
func main() {
logMessage("INFO", "Server", "started", "on", "port", "8080")
// Output: [INFO] Server started on port 8080
logMessage("ERROR", "Failed", "to", "connect", "to", "database")
// Output: [ERROR] Failed to connect to database
logMessage("DEBUG", "User", "logged", "in")
// Output: [DEBUG] User logged in
}
Important Rules for Variadic Parameters:
-
Only One Variadic Parameter: A function can only have one variadic parameter, and it must be the last parameter in the function signature. You cannot have multiple variadic parameters.
// Invalid: multiple variadic parameters func invalidFunction(a ...int, b ...string) {} // Valid: one variadic parameter func validFunction(a string, b ...int) {}
-
Calling with Zero Arguments: You can always call a variadic function with zero arguments for the variadic parameter. In such cases, the variadic parameter will be an empty slice.
-
Passing a Slice: When passing an existing slice to a variadic function, you need to "unpack" the slice using
...
.
Example 5: Using Variadic Parameters with Structs
Here’s an advanced example where a variadic function processes a list of structs:
package main
import "fmt"
type Person struct {
Name string
Age int
}
// Variadic function to process a list of Person structs
func printPeople(people ...Person) {
for _, person := range people {
fmt.Printf("Name: %s, Age: %d
", person.Name, person.Age)
}
}
func main() {
person1 := Person{"Alice", 25}
person2 := Person{"Bob", 30}
printPeople(person1, person2)
// Output:
// Name: Alice, Age: 25
// Name: Bob, Age: 30
}
Summary:
...
(variadic parameters) allow a function to accept a variable number of arguments.- The variadic arguments are treated as a slice inside the function.
- You can mix regular parameters and variadic parameters, but the variadic parameter must be the last one.
- You can pass an existing slice to a variadic function by using the
...
operator to "unpack" the slice.
Variadic parameters provide a powerful and flexible way to handle functions that need to work with a variable number of arguments. They make your code more concise and adaptable, especially for cases like logging, sum calculations, and formatting messages.
You can download the full blog post here.
LinkedIn Copy
🚀 Master Go's Variadic Syntax (...): A Comprehensive Guide!
📝 In this article, we dive deep into Go’s variadic syntax (using ...
) and explore how it enables you to create flexible functions that accept any number of arguments. From handling sums, strings, slices, to mixing different types in one function—variadic parameters are a game-changer for your Go code. Check out examples and learn how to apply this technique in your projects.
🔗 Read the full guide here: [link] #GoLang #Programming #Go #Developers #CodingTips #Backend