When to Prefer Map Over Object in JavaScript
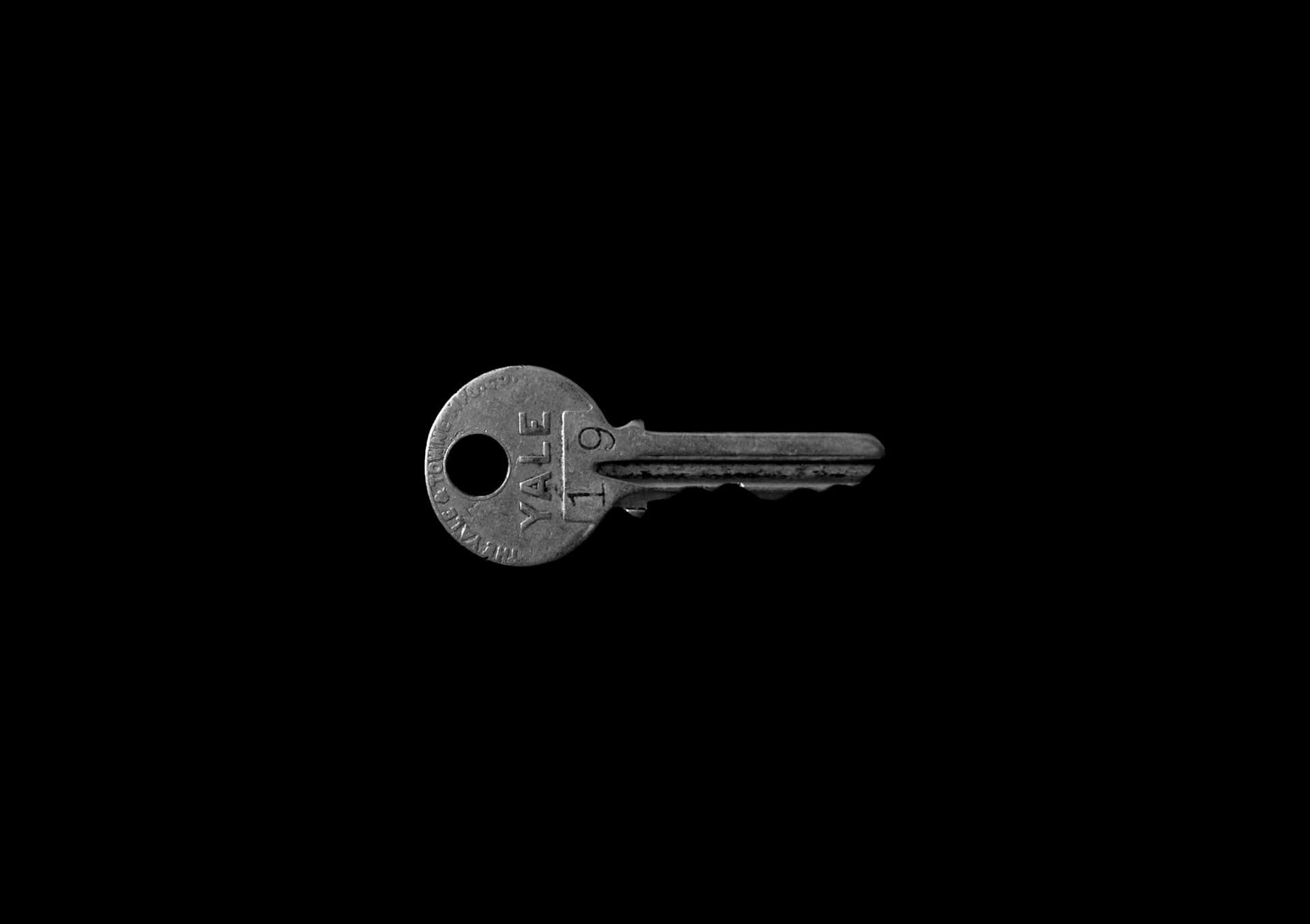
Table of Content
- When to Prefer Map Over Object in JavaScript
- TL;DR
- Why `Object` Falls Short as a Hash Map
- **Key Limitations**
- Why `Map` Excels for Hash Maps
- **Key Advantages**
- Performance Benchmarks
- **String Keys**
- **Integer Keys**
- **Memory Usage**
- When to Use `Map` Over `Object`
- **Use `Object` When:**
- **Use `Map` When:**
- Browser Compatibility
- Conclusion
When to Prefer Map Over Object in JavaScript
JavaScript objects are versatile, allowing you to group data together easily. But since ES6, the Map
object has entered the scene, offering unique capabilities for certain use cases. While many developers default to using objects for everything, this can lead to performance and security issues.
In this article, we’ll explore the differences between Object
and Map
, their use cases, performance characteristics, and when you should choose one over the other.
TL;DR
- Use
Object
for fixed, finite records like configuration objects or one-time use data structures. - Use
Map
for dynamic dictionaries or hash maps with frequent updates, especially when keys aren't known ahead of time. Map
is generally faster thanObject
for insertion, deletion, and iteration, and it consumes less memory.
Why Object
Falls Short as a Hash Map
Key Limitations
-
String-Only Keys
Object
keys must be strings or symbols. Non-string keys are converted to strings, leading to unexpected behavior:const foo = []; const obj = { [foo]: "value" }; console.log(obj); // { "": "value" }
-
Inheritance Issues
By default, objects inherit fromObject.prototype
, leading to unwanted properties and potential prototype pollution:const hashMap = {}; // inherits from Object.prototype console.log(hashMap.toString); // [Function: toString]
This can also cause name collisions when a user-defined property shadows an inherited one.
-
Cumbersome API
Common tasks like determining size, clearing properties, or checking existence are more complicated with objects compared toMap
:const obj = { a: undefined }; console.log(Object.keys(obj).length); // 1 (size workaround) console.log(obj.hasOwnProperty("a")); // true
-
Performance Overhead
Objects require additional workarounds for tasks like iterating over keys or deleting properties.
Why Map
Excels for Hash Maps
Key Advantages
-
Flexible Key Types
Map
supports any data type as keys, including objects and functions:const map = new Map(); const key = {}; map.set(key, "value"); console.log(map.get(key)); // "value"
-
Better Ergonomics
Map
offers dedicated APIs for common tasks:size
: Easily get the number of entries.clear
: Remove all entries in one operation.has
: Check if a key exists.get
: Retrieve a value associated with a key.
-
Performance Benefits
Benchmarks show thatMap
is faster thanObject
for most operations, especially as the number of entries grows.Operation Object Map Insertion Slower Faster Deletion Moderate Faster Iteration Slower Faster -
Memory Efficiency
Map
consumes less memory than an object of the same size.
Performance Benchmarks
String Keys
For non-numeric string keys, Map
outperforms Object
in all operations:
- Insertion:
Map
is initially twice as fast asObject
but converges as the size grows. - Iteration:
Map
maintains a consistent advantage.
Integer Keys
For integer keys (e.g., 1
, 2
), objects can sometimes outperform Map
due to V8 optimizations, particularly for small-sized objects. However, as size increases, Map
regains its advantage.
Memory Usage
Map
consistently uses 20–50% less memory than Object
.
When to Use Map
Over Object
Use Object
When:
- The structure has a fixed set of properties (e.g., a configuration object).
- You prioritize simplicity over performance.
- Keys are known and limited to strings or symbols.
Use Map
When:
- You need a dynamic key-value store with frequent updates.
- Keys are of diverse types (e.g., objects, numbers, or functions).
- Iteration order and performance are critical.
- You need a reliable size API or frequent key checks.
Browser Compatibility
Map
is widely supported in modern browsers, including IE 11 (though IE 11 is officially obsolete). Unless you're targeting legacy environments, you can safely use Map
.
Conclusion
Map
is a better choice than Object
for hash maps, offering more features, better performance, and fewer pitfalls. However, Object
still excels as a simple record structure for finite, predefined fields. Understanding their differences will help you make more informed decisions in your JavaScript development.