When to Use Synchronous and Asynchronous File Methods in Node.js
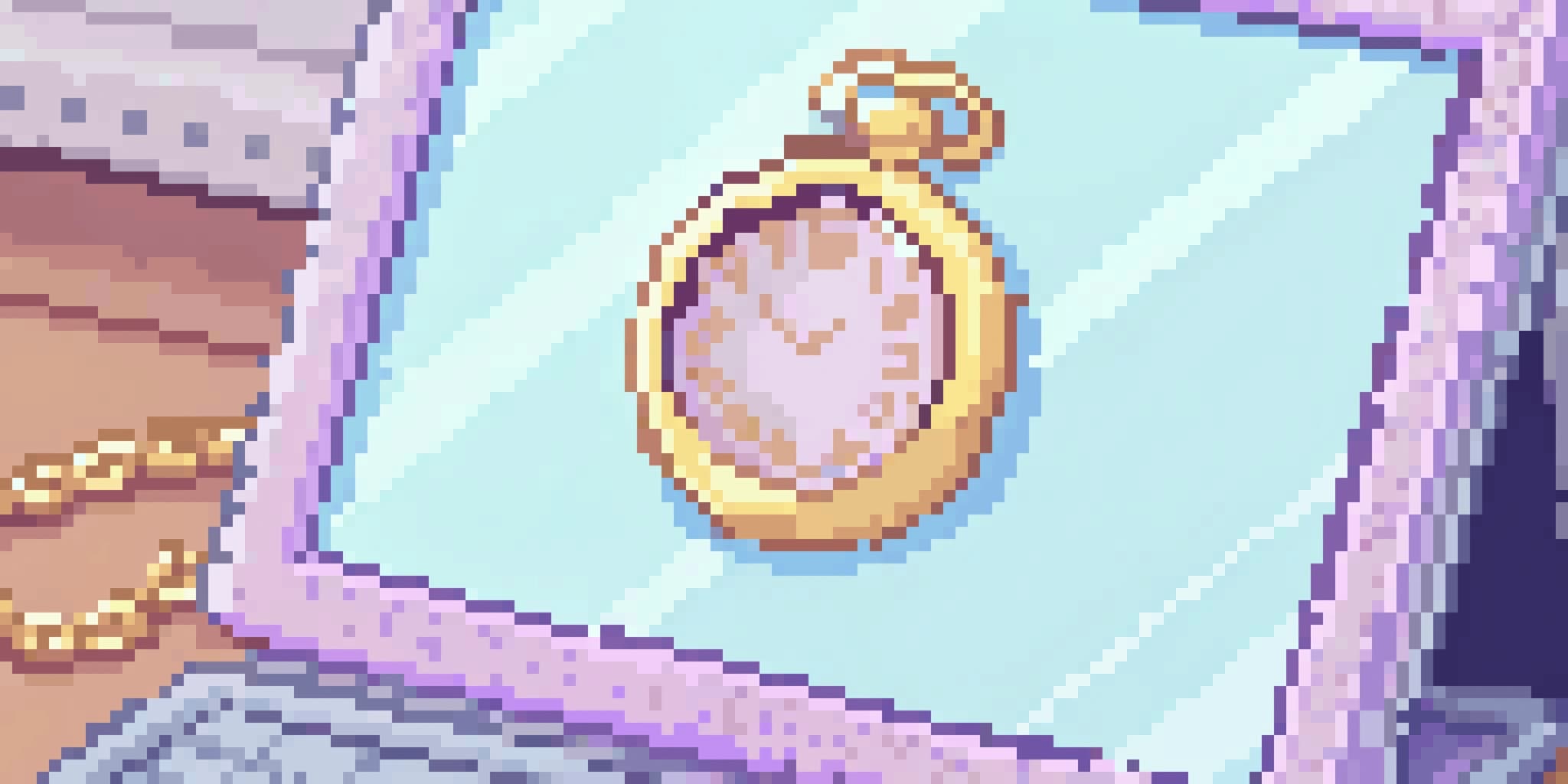
Table of Content
- Understanding Synchronous and Asynchronous File Methods
- Synchronous File Methods
- Asynchronous File Methods
- When to Use Synchronous File Methods
- 1. During Startup or Initialization
- 2. Scripts or CLI Tools
- When to Use Asynchronous File Methods
- 1. In Server Environments
- 2. For Large Files or Long Operations
- Key Considerations When Choosing Sync vs. Async
- Best Practices
- Conclusion
Node.js, renowned for its non-blocking I/O capabilities, provides two types of file system methods: synchronous (sync) and asynchronous (async). Choosing the right method is critical to ensuring efficient performance and scalability in your applications. This article explores the differences between these methods, when to use each, and how they impact your application's behavior.
Key takeaway: Use synchronous methods for simplicity in startup routines or scripts, but rely on asynchronous methods for scalability and performance in production environments.
Understanding Synchronous and Asynchronous File Methods
Synchronous File Methods
Synchronous methods in Node.js block the execution of code until the current operation completes. These methods are typically marked with a Sync
suffix (e.g., readFileSync
, writeFileSync
).
Example:
const fs = require("fs");
try {
const data = fs.readFileSync("file.txt", "utf-8");
console.log(data);
} catch (err) {
console.error(err);
}
How it works:
- Node.js halts further code execution until the file is fully read.
- The result (or error) is returned immediately after the operation completes.
Asynchronous File Methods
Asynchronous methods use callbacks, promises, or async/await
to execute file operations without blocking the event loop. These methods allow other parts of your application to continue executing while waiting for the I/O operation to finish.
Example using callbacks:
const fs = require("fs");
fs.readFile("file.txt", "utf-8", (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
Example using promises/async-await:
const fs = require("fs/promises");
async function readFile() {
try {
const data = await fs.readFile("file.txt", "utf-8");
console.log(data);
} catch (err) {
console.error(err);
}
}
readFile();
When to Use Synchronous File Methods
1. During Startup or Initialization
Synchronous methods are suitable when performing file operations during the application's initialization phase. For example, reading configuration files before starting the server.
const fs = require("fs");
const config = JSON.parse(fs.readFileSync("config.json", "utf-8"));
// Initialize application with config
- Blocking the event loop is acceptable since the application is not yet serving requests.
- Synchronous operations simplify the code as callbacks or promises are unnecessary.
2. Scripts or CLI Tools
For small scripts or command-line tools, synchronous methods are often simpler and easier to manage.
const fs = require("fs");
const data = fs.readFileSync(process.argv[2], "utf-8");
console.log(data);
- Performance and scalability are less critical in one-off executions.
- Blocking is rarely noticeable in small, single-task scripts.
When to Use Asynchronous File Methods
1. In Server Environments
Async methods shine in server-side code where high-concurrency handling is required. Blocking the event loop with synchronous operations can degrade performance under high load.
Example:
const fs = require("fs");
require("http")
.createServer((req, res) => {
fs.readFile("file.txt", "utf-8", (err, data) => {
if (err) {
res.writeHead(500);
res.end("Error reading file");
return;
}
res.writeHead(200);
res.end(data);
});
})
.listen(3000);
- Prevents the server from becoming unresponsive.
- Allows the event loop to handle other requests while waiting for I/O operations to complete.
2. For Large Files or Long Operations
Async methods ensure responsiveness when working with large files or lengthy I/O operations.
Example:
const fs = require("fs");
fs.readFile("large-file.txt", "utf-8", (err, data) => {
if (err) {
console.error(err);
return;
}
console.log("File read successfully");
});
- Keeps the application responsive, even with large datasets.
- Improves user experience by avoiding freezes.
Key Considerations When Choosing Sync vs. Async
-
Performance Requirements:
- Use async methods for scalable applications handling multiple users or requests.
- Use sync methods for quick operations where blocking is acceptable.
-
Complexity vs. Simplicity:
- Sync methods are easier to write and debug but block the event loop.
- Async methods ensure responsiveness but involve managing callbacks or promises.
-
Environment Context:
- Development/Prototyping: Sync methods may be quicker to implement.
- Production/Server: Always prefer async methods for scalability.
-
Error Handling:
- Sync methods throw exceptions directly, simplifying error management in small scripts.
- Async methods require explicit error handling via callbacks or
try-catch
.
Best Practices
- Default to Async: Always use async methods unless you have a specific reason to use sync.
- Use Promises or Async/Await: Avoid callback hell with modern async syntax.
- Minimize Blocking Code: Even in scripts, avoid sync methods for large files or slow I/O.
Conclusion
Choosing between synchronous and asynchronous file methods in Node.js depends on the use case and performance requirements. Synchronous methods are best for startup tasks and simple scripts, while asynchronous methods are essential for scalable and responsive applications. By understanding the implications of each approach, you can build efficient, reliable, and high-performance Node.js applications.